Java で OutputStream を文字列に変換する
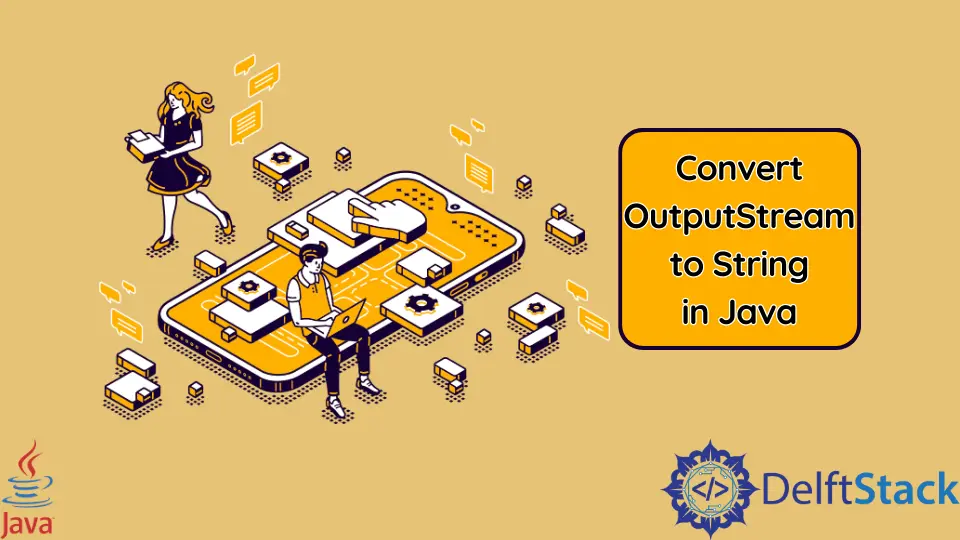
このチュートリアルでは、Java で OutputStream
を String
に変換する方法を示します。
OutputStream
を Java で String
に変換する
OutputStream は Java の io パッケージの抽象クラスで、 FileOutputStream 、 ByteArrayOutputStream 、 ObjectOutputStream などのサブクラスが含まれています。
一方、String
は単純な文字セットです。 したがって、OutputStream
を String
に変換するのは簡単です。 これを行うには、java.io.ByteArrayOutputStream.toString()
メソッドを使用できます。
または、OutputStream
を String
にキャストできます。 OutputStream
を String
に変換するコード例を書きましょう。
例 1:
このコードには 5つのステップが含まれています。 まず、空の String
を初期化します。 次に、String
の ASCII
値で配列を作成します。 3 番目のステップでは、OutputStream
のオブジェクトを作成します。
4 番目に、write
メソッドを使用して Byte
配列をオブジェクトにコピーします。 最後に、最終的な文字列を出力します。
package delftstack;
import java.io.*;
class Example1 {
public static void main(String[] args) throws IOException {
// Initialize empty string and byte array
String DemoString = "";
byte[] ByteArray = {72, 101, 108, 108, 111, 33, 32, 84, 104, 105, 115, 32, 105, 115, 32, 100,
101, 108, 102, 116, 115, 116, 97, 99, 107, 46, 99, 111, 109, 46, 46};
// create ByteArrayOutputStream
ByteArrayOutputStream Output_Stream = new ByteArrayOutputStream();
// Now write byte array to the output stream
Output_Stream.write(ByteArray);
// convert buffers using the toString method into String type
DemoString = Output_Stream.toString();
// print The String
System.out.println("The converted String is: " + DemoString);
}
}
上記のコードは、ASCII
値を持つ ByteArrayOutputStream
を String
に変換します。 以下の出力を参照してください。
The converted String is: Hello! This is delftstack.com..
例 2:
ここでは、4つの手順に従ってコードを記述できます。 まず、ByteArrayOutputStream
のオブジェクトを作成します。 次に、空の変数を String
変数として初期化します。
3 番目に、write
メソッドを使用して String
を OutputStream
にコピーします。 最後に、最終的な String
を次の方法で初期化します。
String Final_String = new String(Output_Stream.toByteArray());
次のコードを書いて学習しましょう。
package delftstack;
import java.io.*;
class Example2 {
public static void main(String[] args) throws IOException {
// declare ByteArrayOutputStream
ByteArrayOutputStream Output_Stream = new ByteArrayOutputStream();
// Initiale string
String DemoString = "Hello! This is delftstack.com..";
// write the bytes to the output stream
Output_Stream.write(DemoString.getBytes());
// convert the stream to byte array and use typecasting
String Final_String = new String(Output_Stream.toByteArray());
// print the strings
System.out.println("The Demo String is: " + DemoString);
System.out.println("The final String is: " + Final_String);
}
}
上記のコードは、ByteArrayOutputStream
を String
に変換します。 以下の例を参照してください。
The Demo String is: Hello! This is delftstack.com..
The final String is: Hello! This is delftstack.com..
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook