Convertir OutputStream a cadena en Java
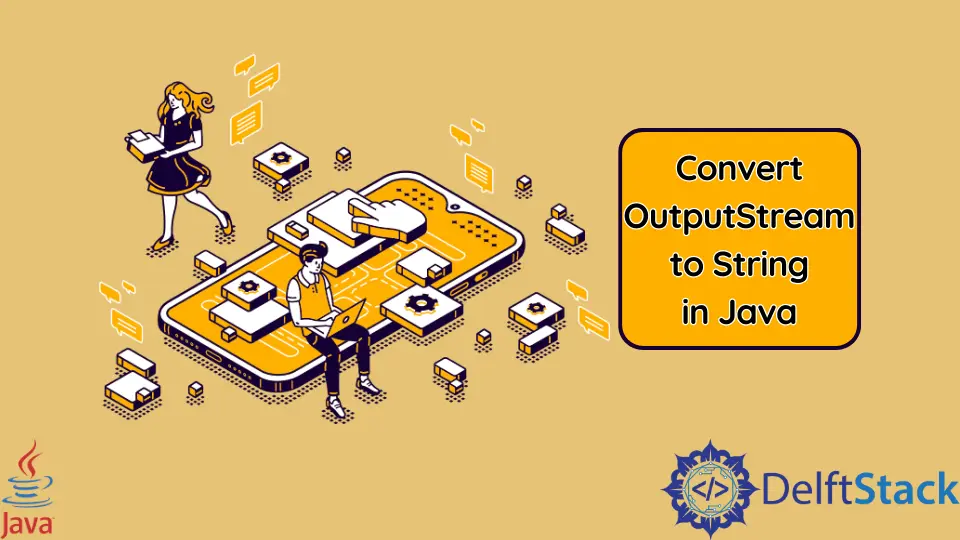
Este tutorial demuestra cómo convertir OutputStream
a String
en Java.
Convierta OutputStream
a String
en Java
OutputStream
es una clase abstracta del paquete io
de java que incluye subclases como FileOutputStream
, ByteArrayOutputStream
, ObjectOutputStream
, etc.
Por otro lado, String
es un conjunto simple de caracteres. Por lo tanto, convertir OutputStream
en String
es fácil. Para hacer eso, podemos usar el método java.io.ByteArrayOutputStream.toString()
.
O podemos lanzar un OutputStream
a una String
. Escribamos ejemplos de código para convertir OutputStream
en String
.
Ejemplo uno:
Este código incluye los cinco pasos. Primero, inicialice una Cadena
vacía. En segundo lugar, cree una matriz con valores ASCII
de su Cadena
. En el tercer paso, crea un objeto de OutputStream
.
Cuarto, use el método escribir
para copiar la matriz Byte
al objeto. Finalmente, imprima las cadenas finales.
package delftstack;
import java.io.*;
class Example1 {
public static void main(String[] args) throws IOException {
// Initialize empty string and byte array
String DemoString = "";
byte[] ByteArray = {72, 101, 108, 108, 111, 33, 32, 84, 104, 105, 115, 32, 105, 115, 32, 100,
101, 108, 102, 116, 115, 116, 97, 99, 107, 46, 99, 111, 109, 46, 46};
// create ByteArrayOutputStream
ByteArrayOutputStream Output_Stream = new ByteArrayOutputStream();
// Now write byte array to the output stream
Output_Stream.write(ByteArray);
// convert buffers using the toString method into String type
DemoString = Output_Stream.toString();
// print The String
System.out.println("The converted String is: " + DemoString);
}
}
El código anterior convertirá el ByteArrayOutputStream
con valor ASCII
en String
. Vea la salida a continuación.
The converted String is: Hello! This is delftstack.com..
Ejemplo dos:
Aquí, podemos escribir el código siguiendo cuatro pasos. Primero, crea un objeto de ByteArrayOutputStream
. En segundo lugar, inicialice una variable vacía como una variable String
.
En tercer lugar, use el método escribir
para copiar la Cadena
en el Stream de salida
. Finalmente, inicializa un String
final de la siguiente forma.
String Final_String = new String(Output_Stream.toByteArray());
Vamos a aprenderlo escribiendo el siguiente código.
package delftstack;
import java.io.*;
class Example2 {
public static void main(String[] args) throws IOException {
// declare ByteArrayOutputStream
ByteArrayOutputStream Output_Stream = new ByteArrayOutputStream();
// Initiale string
String DemoString = "Hello! This is delftstack.com..";
// write the bytes to the output stream
Output_Stream.write(DemoString.getBytes());
// convert the stream to byte array and use typecasting
String Final_String = new String(Output_Stream.toByteArray());
// print the strings
System.out.println("The Demo String is: " + DemoString);
System.out.println("The final String is: " + Final_String);
}
}
El código anterior convertirá el ByteArrayOutputStream
en un String
. Vea el ejemplo a continuación.
The Demo String is: Hello! This is delftstack.com..
The final String is: Hello! This is delftstack.com..
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook