How to Convert List to ArrayList in Java
-
Importance of Converting a
List
to anArrayList
in Java -
Methods on How to Convert a
List
to anArrayList
in Java -
Best Practices in Converting a
List
to anArrayList
in Java - Conclusion
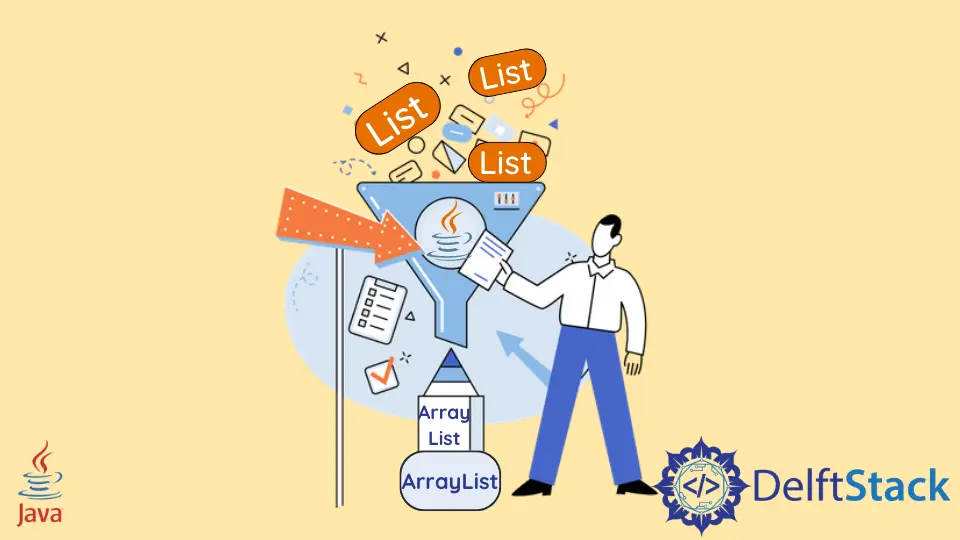
Explore the significance, diverse methods including the ArrayList
constructor, addAll()
, and Java 8 streams, along with best practices for converting a List to an ArrayList
in Java.
Learn more about the difference between List
and ArrayList
here.
Importance of Converting a List
to an ArrayList
in Java
Converting a List
to an ArrayList
in Java is crucial for enhanced flexibility and functionality. ArrayList
offers dynamic resizing, efficient random access, and additional methods compared to a basic List
.
This conversion allows developers to leverage specific features of ArrayList
when manipulating and managing collections, improving performance and simplifying code implementation.
Methods on How to Convert a List
to an ArrayList
in Java
Using the ArrayList
Constructor
Using the ArrayList
constructor in Java to convert a List
to an ArrayList
is important for its simplicity and directness. It efficiently creates an ArrayList
and copies elements in a single step, minimizing code complexity compared to other methods like addAll
or Java 8 streams, making the conversion straightforward and readable.
Consider a scenario where you have a List
of strings, and you want to convert it into an ArrayList
. Below is a complete working code example demonstrating the use of the ArrayList
constructor for this purpose:
import java.util.ArrayList;
import java.util.List;
public class ListToArrayListExample {
public static void main(String[] args) {
// Create a List of strings
List<String> stringList = new ArrayList<>();
stringList.add("Java");
stringList.add("is");
stringList.add("awesome");
// Convert List to ArrayList using ArrayList constructor
ArrayList<String> arrayList = new ArrayList<>(stringList);
// Display the elements of the ArrayList
System.out.println("ArrayList elements: " + arrayList);
}
}
In this code breakdown, we start by importing essential classes from the java.util
package, namely ArrayList
and List
. The class definition establishes a structure named ListToArrayListExample
.
The program’s execution unfolds within the main
method. Creating a List<String>
named stringList
, we populate it with three string elements.
The pivotal step involves using the ArrayList
constructor to convert stringList
into an ArrayList<String>
, streamlining the conversion process.
The concluding action is to display the elements of the resulting ArrayList
on the console. This systematic breakdown illuminates the logic and flow of the code, facilitating understanding for both newcomers and experienced developers.
When you run the program, the output will be:
Converting a List
to an ArrayList
using the ArrayList
constructor is a concise and effective approach. It simplifies the code and enhances readability.
By understanding this method, developers can efficiently manage collections in their Java applications. The presented example serves as a foundation for incorporating this technique into various scenarios, facilitating a seamless transition from List
to ArrayList
with ease.
Using the addAll()
Method
The addAll()
method is crucial for its efficiency in converting a List
to an ArrayList
in Java. It simplifies the process by dynamically adding all elements from the source List
to the target ArrayList
.
This concise approach reduces code complexity compared to other methods, providing a clear and effective means of achieving the conversion with minimal effort.
Consider a situation where you have a List
of integers, and you want to transform it into an ArrayList
. Below is a complete working code example illustrating the use of the addAll()
method for this purpose:
import java.util.ArrayList;
import java.util.List;
public class ListToArrayListAddAllExample {
public static void main(String[] args) {
// Create a List of integers
List<Integer> integerList = List.of(1, 2, 3, 4, 5);
// Convert List to ArrayList using addAll() method
ArrayList<Integer> arrayList = new ArrayList<>();
arrayList.addAll(integerList);
// Display the elements of the ArrayList
System.out.println("ArrayList elements: " + arrayList);
}
}
In this code breakdown, we begin by importing essential classes, namely ArrayList
and List
, from the java.util
package. The class definition establishes a structure named ListToArrayListAddAllExample
.
The program’s execution initiates within the main
method. Creating a List<Integer>
named integerList
with five integer elements, the pivotal step involves using the addAll()
method to seamlessly add all elements from integerList
to the ArrayList
named arrayList
.
Finally, the resulting ArrayList
elements are displayed on the console. This systematic breakdown enhances clarity, making the conversion from List
to ArrayList
straightforward and concise.
When you run the program, the output will be:
The addAll()
method provides a concise and efficient way to convert a List
to an ArrayList
in Java. By dynamically adding elements from one collection to another, developers can adapt to changing requirements without the need for explicit iteration.
This example demonstrates the simplicity and effectiveness of the addAll()
method, showcasing its utility in creating an ArrayList
populated with elements from an existing List
. Incorporating this technique into your codebase enhances flexibility and readability, making it a valuable tool in Java programming.
Using the Java 8 Streams
Java 8 streams offer a concise and expressive way to convert a List
to an ArrayList
, enhancing code readability. The streamlined syntax facilitates a declarative approach, reducing boilerplate code.
Leveraging functions like stream()
, collect()
, and toCollection()
, Java 8 streams provide an elegant and efficient method for this conversion, aligning with modern programming paradigms and simplifying complex operations.
Consider a scenario where you have a List
of strings, and you wish to transform it into an ArrayList
. Below is a complete working code example showcasing the usage of Java 8 streams for this conversion:
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class ListToArrayListStreamsExample {
public static void main(String[] args) {
// Create a List of strings
List<String> stringList = List.of("Java", "is", "streamlining", "collections");
// Convert List to ArrayList using Java 8 Streams
ArrayList<String> arrayList =
stringList.stream().collect(Collectors.toCollection(ArrayList::new));
// Display the elements of the ArrayList
System.out.println("ArrayList elements: " + arrayList);
}
}
We start by importing the necessary classes for our Java program. The class, named ListToArrayListStreamsExample
, encapsulates our code structure.
The program’s execution unfolds within the main
method, the starting point of our application. Creating a List
of strings, we then use Java 8 streams to transform this List
into an ArrayList
.
Leveraging the collect
method and Collectors.toCollection
, we seamlessly accumulate the stream elements into a new ArrayList
. This concise and expressive approach aligns with modern programming paradigms.
Finally, we display the elements of the resulting ArrayList
, showcasing the simplicity and elegance of Java 8 streams.
Upon executing the program, the output will be:
Utilizing Java 8 streams to convert a List
to an ArrayList
brings a concise and expressive approach to collection manipulation. The example demonstrates how, with a single line of code, you can leverage the power of streams and collectors to achieve this conversion elegantly.
Incorporating Java 8 features not only enhances code readability but also aligns with modern programming paradigms, making your codebase more maintainable and adaptable.
Best Practices in Converting a List
to an ArrayList
in Java
Utilize the ArrayList
Constructor
When converting a List
to an ArrayList
, employing the ArrayList
constructor is a straightforward and efficient practice. Pass the existing List
as a parameter to the constructor, simplifying the conversion process.
Leverage the addAll()
Method
The addAll()
method is a versatile option for appending all elements from one collection to another. Use it to dynamically add elements from the source List
to the target ArrayList
, enhancing flexibility and code readability.
Embrace Java 8 Streams
In Java 8 and above, leverage the power of streams for concise and expressive code. Utilize the stream()
method, collect()
operation, and toCollection()
collector to seamlessly convert a List
to an ArrayList
, aligning with modern programming paradigms.
Mindful Generic Types
When using methods like toArray()
, pay attention to generic types. Ensure proper casting to avoid unchecked cast warnings and maintain type safety in the conversion process.
Consider Library Functions
Explore libraries like Google Guava, offering utility functions like Lists.newArrayList()
. These can provide additional options and simplify the code when converting collections.
Aim for Readability
Prioritize code readability in your conversion approach. Choose the method that aligns with the overall style of your codebase and enhances understanding for developers who maintain or review the code.
Conclusion
Converting a List
to an ArrayList
in Java is a pivotal operation, offering improved flexibility and functionality. Whether utilizing the simplicity of the ArrayList
constructor, the versatility of addAll()
, or the expressiveness of Java 8 streams, each method has its strengths.
Best practices, such as mindful generic typing and consideration of library functions, contribute to code readability and maintainability. By adopting these practices, developers can ensure efficient and adaptable collection management, aligning with modern programming paradigms in Java.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java List
- How to Split a List Into Chunks in Java
- How to Get First Element From List in Java
- How to Find the Index of an Element in a List Using Java
- How to Filter List in Java
- Differences Between List and Arraylist in Java
- List vs. Array in Java