How to Convert Boolean to Int in Java
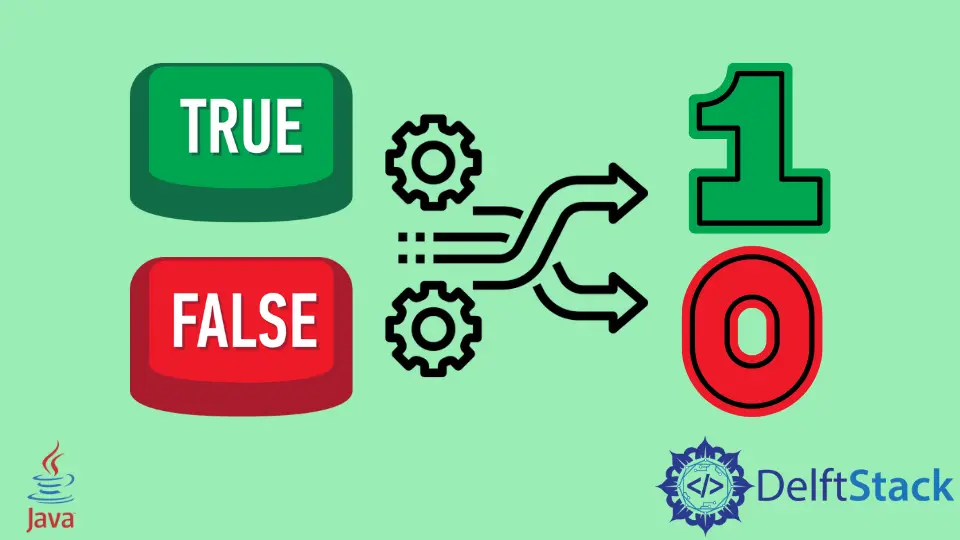
This article explores the significance of converting boolean to int in Java and presents various concise methods, including the ternary operator, if-else
statements, boolean.hashCode()
, boolean.compare()
, and boolean.valueOf()
combined with compareTo()
.
Importance of Converting Boolean to Int in Java
Converting boolean to int in Java is crucial for seamless integration with numerical operations, simplifying conditional expressions, and enhancing data compatibility. It enables boolean values to participate in arithmetic calculations and facilitates the consistent representation of true as 1
and false as 0
, aligning with integer-based logic and storage requirements in various programming scenarios.
Methods to Convert Boolean to Int
Using the Ternary Operator
The ternary operator in Java provides a concise and expressive way to convert a boolean to an int compared to other methods. Its succinct syntax condenses the conversion logic into a single line, enhancing code readability.
This brevity is particularly valuable in scenarios where simplicity and clarity are priorities, making the code more maintainable and easier to understand compared to longer if-else
constructs or other methods. The ternary operator, with its streamlined form, is a preferred choice for boolean to int conversion, offering efficiency without compromising code readability.
Code Example:
public class BooleanToIntConversion {
public static void main(String[] args) {
// Example boolean values
boolean isTrue = true;
boolean isFalse = false;
// Convert boolean to int using ternary operator
int trueValue = isTrue ? 1 : 0;
int falseValue = isFalse ? 1 : 0;
// Display the results
System.out.println("Converted value for true: " + trueValue);
System.out.println("Converted value for false: " + falseValue);
}
}
We begin by declaring two boolean variables, isTrue
and isFalse
, assigning them the values true
and false
. The heart of the conversion lies in the utilization of the ternary operator.
This compact operator efficiently converts the boolean conditions of isTrue
and isFalse
into integers, storing the results in trueValue
and falseValue
. If the boolean condition is true, the result is 1
; otherwise, it is 0
.
Finally, the converted values are printed to the console, allowing for quick and straightforward verification. This approach, utilizing the ternary operator, streamlines the conversion process, making the code concise and easy to understand.
Output:
The output confirms the successful conversion of boolean values to integers using the ternary operator. This approach simplifies the code and contributes to a more expressive and maintainable programming style.
Using if-else
Statements
The if-else
statement in Java serves as a reliable and traditional approach for converting a boolean to an int. Its importance lies in providing a clear and explicit control flow, allowing developers to define specific logic for true and false conditions.
This approach is particularly valuable when the conversion requirements involve complex conditions or additional processing. The if-else
statement enhances code readability, making the conversion logic easily understandable.
In scenarios where a more elaborate decision-making process is needed, the if-else
statement stands out as a robust and flexible choice for boolean to int conversion in Java.
Code Example:
public class BooleanToIntConversion {
public static void main(String[] args) {
// Example boolean values
boolean isTrue = true;
boolean isFalse = false;
// Convert boolean to int using if-else statements
int trueValue = convertBooleanToInt(isTrue);
int falseValue = convertBooleanToInt(isFalse);
// Display the results
System.out.println("Converted value for true: " + trueValue);
System.out.println("Converted value for false: " + falseValue);
}
// Method to convert boolean to int using if-else
private static int convertBooleanToInt(boolean value) {
if (value) {
return 1;
} else {
return 0;
}
}
}
In this code breakdown, we start by declaring and initializing two boolean variables, isTrue
and isFalse
, with the values true
and false
. We then invoke a method called convertBooleanToInt
that takes these boolean values as parameters, producing results stored in trueValue
and falseValue
.
Inside the method, an if-else
statement is employed to determine the integer value to return based on the input boolean. If the boolean
is true, the method returns 1
; otherwise, it returns 0
.
The converted values are eventually displayed on the console. This use of if-else
statements offers a straightforward and readable control flow for boolean to int conversion, adding clarity to the code.
Output:
The output confirms the successful conversion of boolean values to integers using if-else
statements. This method, while more lengthy compared to some alternatives, offers explicit control over the conversion logic and is a valuable tool in the Java developer’s arsenal.
Using the boolean.hashCode()
Method
The importance of using boolean.hashCode()
in converting boolean to int in Java lies in its simplicity and efficiency. This method directly provides an integer representation of boolean values, where true
is mapped to 1231
and false
to 1237
.
In Java’s Boolean.hashCode()
method, true
is represented by the integer value 1231
, and false
is represented by 1237
. These specific values are chosen to create distinct hash codes for the two boolean states, ensuring a low likelihood of collisions in hash-based data structures.
The numbers 1231
and 1237
are primes, enhancing their effectiveness in generating unique hash codes and minimizing the risk of hash collisions, thereby supporting reliable boolean to int conversion.
Its concise syntax eliminates the need for conditional statements or complex conversions, making the code more readable and straightforward. In scenarios where a quick and direct boolean to int mapping is required, boolean.hashCode()
stands out as a convenient and effective choice, streamlining the conversion process.
Code Example:
public class BooleanToIntConversion {
public static void main(String[] args) {
// Example boolean values
boolean isTrue = true;
boolean isFalse = false;
// Convert boolean to int using boolean.hashCode()
int trueValue = Boolean.hashCode(isTrue);
int falseValue = Boolean.hashCode(isFalse);
// Display the results
System.out.println("Converted value for true: " + trueValue);
System.out.println("Converted value for false: " + falseValue);
}
}
In breaking down the code, we start by declaring and initializing two boolean variables, isTrue
and isFalse
, with the values true
and false
. The essence of the conversion lies in the application of the boolean.hashCode()
method to these boolean values.
This method directly transforms the booleans into integers, with true
corresponding to 1231
and false
to 1237
. The resulting integer values are stored in trueValue
and falseValue
.
Finally, to confirm the successful conversion, the code prints these values to the console. The use of boolean.hashCode()
streamlines the process, offering a concise and effective means of converting boolean values to integers in Java.
Output:
The output confirms the successful conversion of boolean values to integers using the boolean.hashCode()
method. This approach offers simplicity and readability, making it a valuable tool for Java developers when faced with boolean to int conversion tasks.
Using the boolean.compare()
Method
The significance of using boolean.compare()
in converting boolean to int in Java lies in its direct and straightforward approach. This method inherently compares boolean values, returning an integer that signifies their relative order.
For true
, the result is 1
, and for false
, it is 0
. This simplicity eliminates the need for explicit if-else
constructs or complex conversions, resulting in concise and readable code.
In scenarios where a quick and intuitive boolean to int mapping is essential, boolean.compare()
serves as a clear and efficient choice, enhancing the efficiency and readability of the conversion process.
Code Example:
public class BooleanToIntConversion {
public static void main(String[] args) {
// Example boolean values
boolean isTrue = true;
boolean isFalse = false;
// Convert boolean to int using boolean.compare()
int trueValue = Boolean.compare(isTrue, false);
int falseValue = Boolean.compare(isFalse, false);
// Display the results
System.out.println("Converted value for true: " + trueValue);
System.out.println("Converted value for false: " + falseValue);
}
}
In understanding the code, we commence by declaring and initializing two boolean variables, isTrue
and isFalse
, set to true
and false
values, respectively. The crux of the conversion lies in the application of the Boolean.compare()
method to these boolean values.
This method efficiently compares the booleans with a reference value (false
) and produces an integer result stored in trueValue
and falseValue
. Subsequently, for verification purposes, the code prints these converted values to the console.
The utilization of boolean.compare()
simplifies the conversion process, offering a succinct and effective means of translating boolean values into integers in Java.
Output:
The output confirms the successful conversion of boolean values to integers using the boolean.compare()
method. This method offers a clean and efficient solution, particularly beneficial in scenarios where a direct comparison is meaningful in the context of boolean to int conversion.
Using the boolean.valueOf()
and compareTo()
Methods
The significance of using Boolean.valueOf()
and compareTo()
in converting boolean to int in Java lies in their combined ability to provide a clear and direct mapping of boolean values to integers. Boolean.valueOf()
converts booleans to Boolean objects, and when coupled with compareTo()
, it allows for straightforward comparison against a reference boolean value, such as Boolean.FALSE
.
This concise approach eliminates the need for complex logic or multiple steps, providing a readable and efficient solution for boolean to int conversion. In scenarios where a concise and expressive method is preferred, the combination of Boolean.valueOf()
and compareTo()
stands out as an effective choice, simplifying the conversion process.
Code Example:
public class BooleanToIntConversion {
public static void main(String[] args) {
// Example boolean values
boolean isTrue = true;
boolean isFalse = false;
// Convert boolean to int using boolean.valueOf() and compareTo()
int trueValue = Boolean.valueOf(isTrue).compareTo(Boolean.FALSE);
int falseValue = Boolean.valueOf(isFalse).compareTo(Boolean.FALSE);
// Display the results
System.out.println("Converted value for true: " + trueValue);
System.out.println("Converted value for false: " + falseValue);
}
}
In dissecting the code, we initiate by declaring and initializing two boolean variables, isTrue
and isFalse
, set to true
and false
values, respectively. The pivotal conversion involves using Boolean.valueOf()
to transform these booleans into Boolean
objects.
The subsequent application of the compareTo()
method compares these Boolean
objects with Boolean.FALSE
, yielding integer values stored in trueValue
and falseValue
. Finally, for confirmation purposes, the code prints these converted values to the console.
The combination of Boolean.valueOf()
and compareTo()
simplifies the boolean to int conversion process, presenting a concise and effective approach in Java.
Output:
The output confirms the successful conversion of boolean values to integers using boolean.valueOf()
and compareTo()
. This approach offers a clear and concise syntax, making it a valuable tool for Java developers dealing with boolean to int conversion tasks.
Conclusion
Each method for converting a boolean to an int in Java offers distinct advantages.
The ternary operator ensures brevity and clarity, the if-else
method enhances readability and reusability, boolean.hashCode()
provides a brief approach, boolean.compare()
offers simplicity through direct comparison, and the combination of boolean.valueOf()
and compareTo()
leverages natural order.
The choice depends on specific needs, emphasizing the flexibility and efficiency of Java in handling boolean to int conversions.
Related Article - Java Integer
- How to Handle Integer Overflow and Underflow in Java
- How to Reverse an Integer in Java
- How to Convert Int to Binary in Java
- The Max Value of an Integer in Java
- Minimum and Maximum Value of Integer in Java
- How to Convert Integer to Int in Java