How to Print Boolean Value Using the printf() Method in Java
-
Print Boolean By Using the
printf()
Method in Java -
Print Boolean By Using the
println()
Method in Java -
Print Boolean By Using the
print()
Method in Java -
Print Boolean By Using the
String.valueOf()
Method in Java -
Print Boolean By Using the
Boolean.toString()
Method in Java -
Print Boolean By Using
StringBuilder
in Java -
Print Boolean By Using
StringBuffer
in Java -
Print Boolean By Using
PrintWriter
in Java - Conclusion
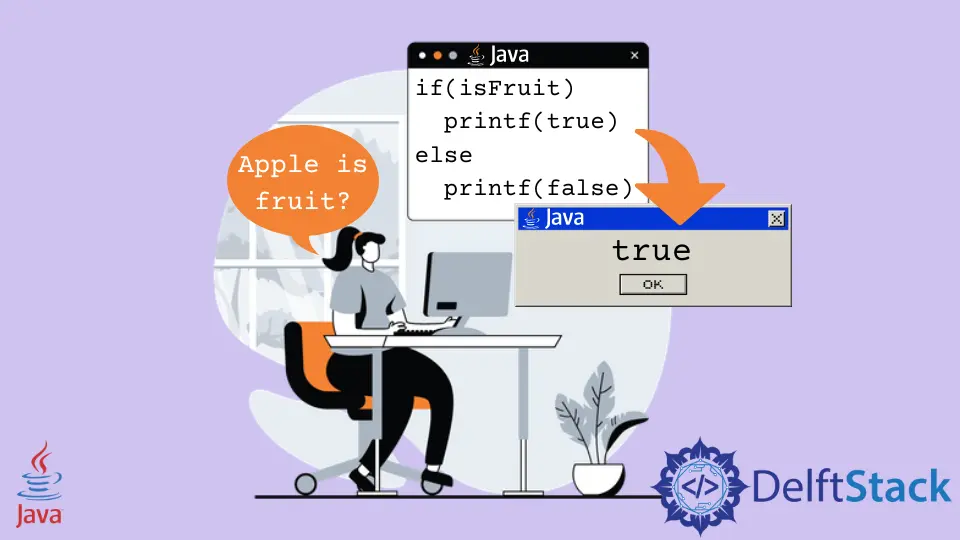
This tutorial introduces several methods to print a Boolean value in Java, building upon the primary focus on the printf()
method.
Boolean is a fundamental data type in Java that can hold either true
or false
literals, typically used in conditional statements. This article will demonstrate various approaches to printing Boolean values using different printing methods in Java.
In Java, to print values to the console, we commonly use the System.out.println()
method, which also works for Boolean values. However, if we need to print formatted output, we use the printf()
method, akin to the printf()
function in the C language.
In Java, the printf()
method belongs to the PrintStream
class and allows formatted output to be displayed on the console. The syntax of this method is as follows:
public PrintStream printf(String format, Object... args)
This method accepts two arguments: a formatted string and the object to print.
The format string can include various format specifiers, including:
Format String | Object Argument/Value |
---|---|
b or B |
It represents a Boolean value. |
h or H |
It represents a hexadecimal value. |
s or S |
It represents a string value. |
c or C |
It represents a character value. |
d |
It represents an integer value. |
f |
It represents a floating value. |
o |
It represents an octal integer value. |
x or X |
It represents a hexadecimal integer. |
e or E |
It represents a decimal number in computerized scientific notation. |
t or T |
It represents date and time conversion characters. |
Let’s understand the printing of Boolean values with some examples.
Print Boolean By Using the printf()
Method in Java
In this example, we used the printf()
method of the PrintStream
class to print a Boolean or formatted output to the console. This method is similar to the println()
method, except it takes two arguments.
To print Boolean values using this method, we have to use the %b
or %B
format specifier. Both specifiers are equivalent and represent a Boolean value.
The %b
specifier formats the Boolean value in lowercase (e.g., true or false), while %B
formats it in uppercase (e.g., TRUE or FALSE).
See the example below.
public class SimpleTesting {
public static void main(String args[]) {
boolean isGreen = true;
findColor(isGreen);
isGreen = false;
findColor(isGreen);
}
static void findColor(boolean isGreen) {
if (isGreen) {
System.out.printf("Apple is green: %b%n", isGreen);
} else {
System.out.printf("Apple is green: %B%n", isGreen);
}
}
}
Output:
Apple is green: true
Apple is green: FALSE
Inside main
, we defined a Boolean variable named isGreen
and set it to true
initially. Then, we called the findColor
method and passed this Boolean variable as an argument.
Inside findColor
, we received the Boolean argument isGreen
and used a conditional statement to check its value. If isGreen
was true
, we utilized the printf()
method to print a message indicating that the apple is green, with the %b
specifier representing the Boolean value in lowercase.
Conversely, if isGreen
was false
, we used %B
in the printf()
method, displaying the Boolean value in uppercase and stating that the apple is not green. We then changed the value of isGreen
to false
and called findColor
again, repeating the process.
Print Boolean By Using the println()
Method in Java
If you don’t want formatted output or the printf()
method, you can use the most used method of Java, the println()
. This method does not require a format specifier, and you can get the result easily to the console.
The syntax for the println()
method is as follows:
public void println(boolean x)
This method accepts a Boolean value (x
) and prints it to the console, followed by a newline character, effectively moving the cursor to the next line.
See the example below.
public class SimpleTesting {
public static void main(String args[]) {
boolean isGreen = true;
findColor(isGreen);
isGreen = false;
findColor(isGreen);
}
static void findColor(boolean isGreen) {
if (isGreen) {
System.out.println("Apple is green: " + isGreen);
} else {
System.out.println("Apple is green: " + isGreen);
}
}
}
Output:
Apple is green: true
Apple is green: false
In this example, we have a Boolean variable: isGreen
. We used the println()
method to print the Boolean value associated with the variable.
Finally, we concatenated the Boolean value with a message to make the output more descriptive.
Print Boolean By Using the print()
Method in Java
You can even use the print()
method without any format specifier string and get the desired result to the console. This method is similar to the println()
method except for printing the result in the same line.
The syntax for the print()
method is as follows:
public void print(boolean x)
This method accepts a Boolean value (x
) and prints it to the console without moving the cursor to the next line.
See the example below.
public class SimpleTesting {
public static void main(String args[]) {
boolean isGreen = true;
findColor(isGreen);
isGreen = false;
findColor(isGreen);
}
static void findColor(boolean isGreen) {
if (isGreen) {
System.out.print("Apple is green: " + isGreen);
} else {
System.out.print("\nApple is green: " + isGreen);
}
}
}
Output:
Apple is green: true
Apple is green: false
In this example, we defined a Boolean variable named isGreen
and set it to true
initially. We then called the findColor
method, passing isGreen
as an argument.
Inside findColor
, we use the print()
method to print the Boolean value associated with the isGreen
variable. We then update the value of isGreen
to false
and call findColor
again, repeating the process for the false
case.
Print Boolean By Using the String.valueOf()
Method in Java
The String.valueOf()
method is a convenient way to convert a Boolean value to its string representation. It’s a static method of the String
class and can be used to convert various types, including Boolean, to their string representations.
The syntax for the String.valueOf()
method is as follows:
public static String valueOf(boolean b)
This method accepts a Boolean value (b
) and returns its string representation.
Let’s explore a practical example to demonstrate how to use the String.valueOf()
method to print Boolean values in Java.
public class PrintBooleanExample {
public static void main(String[] args) {
boolean isGreen = true;
String boolString = String.valueOf(isGreen);
System.out.println("Apple is green: " + boolString);
isGreen = false;
boolString = String.valueOf(isGreen);
System.out.println("Apple is green: " + boolString);
}
}
Output:
Apple is green: trueApple is green: false
In this example, we defined a Boolean variable named isGreen
and set it to true
initially. We then used the String.valueOf()
method to convert the Boolean value isGreen
to its string representation and stored it in the variable boolString
.
We concatenated this string representation with an appropriate message to make the output more descriptive. We repeated the process for the false
case, demonstrating how to use the String.valueOf()
method effectively.
Print Boolean By Using the Boolean.toString()
Method in Java
The Boolean.toString()
method is a static method of the Boolean
class that converts a Boolean value to its string representation. It’s a simple and direct way to convert a Boolean to a string.
Here’s an example of how to use Boolean.toString()
to print a Boolean value:
public class SimpleTesting {
public static void main(String args[]) {
boolean isGreen = true;
String boolString = Boolean.toString(isGreen);
System.out.println("Apple is green: " + boolString);
isGreen = false;
boolString = Boolean.toString(isGreen);
System.out.println("Apple is green: " + boolString);
}
}
Output:
Apple is green: true
Apple is green: false
We started by declaring the same Boolean variable named isGreen
and set it to true
. We then used Boolean.toString(isGreen)
to convert the Boolean value to its string representation, storing it in the boolString
variable.
We then printed the message "Apple is green: "
concatenated with the string representation of the Boolean using System.out.println()
.
Print Boolean By Using StringBuilder
in Java
The StringBuilder
class is efficient for creating and manipulating strings, especially when you need to concatenate multiple strings or add different types of data, including Boolean values.
Here’s an example of using StringBuilder
to print a Boolean value:
public class SimpleTesting {
public static void main(String args[]) {
boolean isGreen = true;
StringBuilder sb = new StringBuilder();
sb.append("Apple is green: ").append(isGreen);
System.out.println(sb.toString());
isGreen = false;
sb.setLength(0); // Clear the StringBuilder
sb.append("Apple is green: ").append(isGreen);
System.out.println(sb.toString());
}
}
Output:
Apple is green: true
Apple is green: false
After declaring the same Boolean variable, we created a StringBuilder
object, sb
, to efficiently build our output string. Using the append()
method, we concatenate the message "Apple is green: "
with the Boolean value (isGreen
).
We converted the StringBuilder
to a string using toString()
and printed it using System.out.println()
.
We repeated the process with isGreen
set to false
, clearing the StringBuilder
before constructing the output for the second Boolean value.
Print Boolean By Using StringBuffer
in Java
Similar to StringBuilder
, the StringBuffer
class is used to create and manipulate strings efficiently. It’s thread-safe but may be slightly slower due to synchronization.
Here’s an example of using StringBuffer
to print a Boolean value:
public class SimpleTesting {
public static void main(String args[]) {
boolean isGreen = true;
StringBuffer sb = new StringBuffer();
sb.append("Apple is green: ").append(isGreen);
System.out.println(sb.toString());
isGreen = false;
sb.setLength(0); // Clear the StringBuffer
sb.append("Apple is green: ").append(isGreen);
System.out.println(sb.toString());
}
}
Output:
Apple is green: true
Apple is green: false
Here, we created a StringBuffer
object, sb
, to efficiently construct our output string. Using the append()
method, we concatenated the message "Apple is green: "
with the Boolean value (isGreen
).
We then converted the StringBuffer
to a string using toString()
and printed it using System.out.println()
. We repeated the process with isGreen
set to false
, clearing the StringBuffer
before constructing the output for the second Boolean value.
Print Boolean By Using PrintWriter
in Java
The PrintWriter
class in Java provides convenient methods for printing formatted representations of objects to a text output stream. It’s a good choice for more advanced output operations.
Here’s an example of using PrintWriter
to print a Boolean value:
import java.io.PrintWriter;
public class SimpleTesting {
public static void main(String args[]) {
boolean isGreen = true;
PrintWriter out = new PrintWriter(System.out);
out.println("Apple is green: " + isGreen);
out.flush();
isGreen = false;
out.println("Apple is green: " + isGreen);
out.flush();
}
}
Output:
Apple is green: true
Apple is green: false
In this example, we created a PrintWriter
object, out
, which is set to write to the standard output (System.out
). Using out.println()
, we print the message "Apple is green: "
concatenated with the Boolean value.
We then explicitly flush the output using flush()
to ensure it’s immediately written to the output stream. We repeated the process with isGreen
set to false
, printing the corresponding message.
The flush()
method is called again to ensure the second message is written to the output stream.
Conclusion
In this article, we explored various methods in Java to print Boolean values to the console. The printf()
method from the PrintStream
class allows for formatted output using the %b
or %B
format specifiers for lowercase and uppercase Boolean representation, respectively.
The println()
method is a simple alternative for printing Boolean values without specific formatting. The print()
method is similar to println()
but prints in the same line.
String.valueOf()
and Boolean.toString()
methods both offer string representations of Boolean values. Additionally, the StringBuilder
and StringBuffer
classes provide efficient ways to concatenate Boolean values with strings.
Lastly, the PrintWriter
class allows for more advanced output operations with the ability to print formatted representations of objects to a text output stream. Understanding these methods provides flexibility in displaying Boolean values according to specific requirements in Java programming.