How to Toggle a Boolean Variable in Java
- Toggle a Boolean Variable in Java
-
Using the
NOT
(!
) Operator to Toggle a Boolean Variable in Java -
Using the
XOR
(^
) Operator to Toggle a Boolean Variable in Java - Using Ternary Condition to Toggle a Boolean Variable in Java
- Using Numeric Conversion to Toggle a Boolean Variable in Java
-
Using the Bitwise
NOT
(~
) Operator to Toggle a Boolean Variable in Java -
Using the Bitwise
XOR
(^=
) Operator to Toggle a Boolean Variable in Java -
Using the
if-else
Loop to Toggle a Boolean Variable in Java - Conclusion
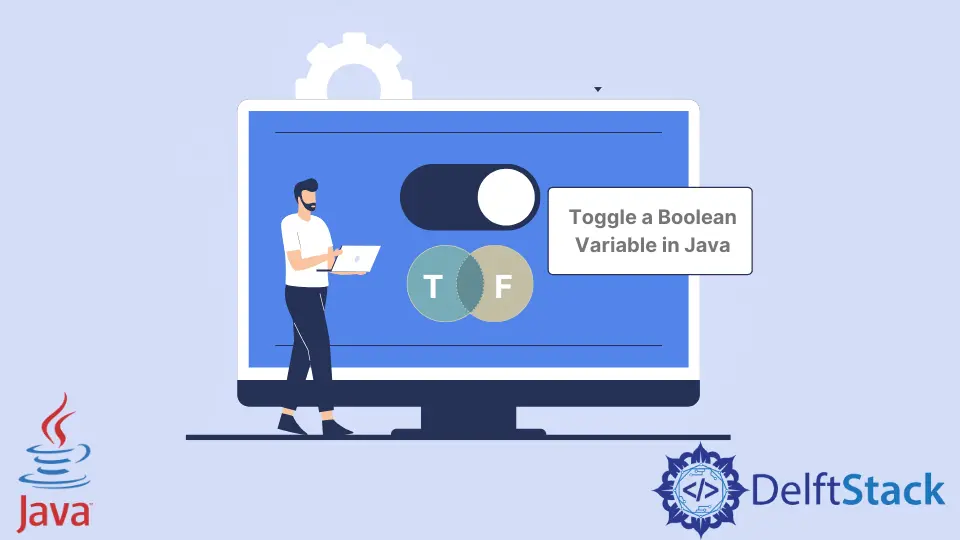
Boolean
is a wrapper class in Java that wraps around boolean
primitives. In object-oriented programming, the class
is a way to maximize object use instead of primitives.
The class concept aroused the solution to bind boolean
or bool
primitive values within the Boolean
class.
Toggle a Boolean Variable in Java
The Boolean
class only has two static values, TRUE
and FALSE
variables. With the static member variables, the Boolean also holds static methods like valueOf()
, parseBoolean
, toString
, and more.
There are many ways for a user to toggle the values of a Boolean variable in a Java program, and we will discuss them in this article one by one.
Using the NOT
(!
) Operator to Toggle a Boolean Variable in Java
The operator !
is also known as the NOT
operator in Java programming. The function of the operator is to negate the operation.
The use of NOT
makes a negative check at places where needed. The use cases can be in the if-else
block, conditional checks in while
and do-while
loops, ternary
operator, and more.
The NOT
operator gets used as a programming practice when validations like not null
are supposed to be made. It gets used with an equals sign to make the equals sign not equal to and then check the condition.
Note that this operator can only be used when the user wants values, like 0
or 1
and true
or false
.
Example:
public class Main {
public static void main(String[] args) {
boolean myBoolean = true;
System.out.println("myBoolean value before: " + myBoolean);
myBoolean = !myBoolean;
System.out.println("myBoolean value after: " + myBoolean);
}
}
Output:
myBoolean value before: true
myBoolean value after: false
In the above code, the not
operator is added along with the Boolean variable to negate its current value.
Since myBoolean
is initially true
, using !myBoolean
will evaluate to false
, and vice versa. So, after this statement, myBoolean
will be toggled to the opposite value.
This is one of the easiest ways to toggle the Boolean variable in one statement.
Using the XOR
(^
) Operator to Toggle a Boolean Variable in Java
The XOR
(exclusive OR
) operator, represented by the caret symbol (^
) in Java, is a bitwise operator that performs a bitwise exclusive OR
operation between the corresponding bits of two operands. In the context of Booleans, the XOR
operator can be used to toggle a Boolean value efficiently.
Here’s how XOR
works:
- If both operands are
false
or both operands aretrue
, the result isfalse
. - If one operand is
false
and the other istrue
, the result istrue
.
Now, let’s see how to use the XOR
operator to toggle a Boolean value in Java:
public class Main {
public static void main(String[] args) {
boolean myBoolean = true;
System.out.println("myBoolean value before: " + myBoolean);
myBoolean = myBoolean ^ true;
System.out.println("myBoolean value after: " + myBoolean);
}
}
Output:
myBoolean value before: true
myBoolean value after: false
When using the XOR
operator to toggle a Boolean value in Java, the process is straightforward. If the Boolean variable myBoolean
is initially set to true
, applying the XOR
operation (true ^ true
) results in false
. Conversely, if myBoolean
is initially false
, the XOR
operation (false ^ true
) evaluates to true
.
In essence, XOR
effectively flips the Boolean value, providing a concise way to toggle between true
and false
states.
This approach is concise and widely used for toggling Boolean values. It takes advantage of the XOR
truth table to switch between true
and false
without the need for conditional statements.
Using Ternary Condition to Toggle a Boolean Variable in Java
The ternary operator, also known as the conditional operator, is a concise way to express conditional statements in Java. It has the following syntax:
condition ? expressionIfTrue : expressionIfFalse;
condition
: This is a Boolean expression that is evaluated.expressionIfTrue
: If the condition istrue
, this expression is evaluated and becomes the result of the entire ternary operation.expressionIfFalse
: If the condition isfalse
, this expression is evaluated and becomes the result.
Now, let’s see how the ternary operator can be used to toggle a Boolean value in Java:
public class Main {
public static void main(String[] args) {
boolean myBoolean = true;
System.out.println("myBoolean value before: " + myBoolean);
// Toggle the boolean value using the ternary operator
myBoolean = (myBoolean) ? false : true;
System.out.println("myBoolean value after: " + myBoolean);
}
}
Output:
myBoolean value before: true
myBoolean value after: false
In this example, myBoolean
is initially set to true
. Then, the ternary operator checks if myBoolean
is true
.
Since myBoolean
is true
, it sets myBoolean
to false
. Thus, after the ternary operation, myBoolean
is toggled to the opposite value.
In summary, the ternary operator quickly toggles the Boolean value based on its current state: if it’s true
, it becomes false
, and if it’s false
, it becomes true
.
Using Numeric Conversion to Toggle a Boolean Variable in Java
Numeric conversion in Java involves converting between numeric types, and it can be used in a somewhat unconventional way to toggle a Boolean value. The idea is to use the numeric representation of 0
and 1
for false
and true
, respectively, and then apply a conversion to toggle the Boolean.
Here’s an example demonstrating this approach:
public class Main {
public static void main(String[] args) {
boolean myBoolean = true;
// Convert boolean to integer representation (0 for false, 1 for true)
int intRepresentation = myBoolean ? 1 : 0;
// Displaying the initial value of myBoolean
System.out.println("myBoolean value before: " + myBoolean);
// Toggle the integer representation
intRepresentation = 1 - intRepresentation;
// Convert back to boolean
myBoolean = intRepresentation == 1;
// Displaying the result
System.out.println("myBoolean value after: " + myBoolean);
}
}
Output:
myBoolean value before: true
myBoolean value after: false
In this example, the ternary operation converts the Boolean value to an integer representation, where true
is represented as 1
and false
as 0
.
An initial value of 1
is given to the myBoolean
, then it is changed to 0
by subtracting 1
from it. Thus, the value of myVariable
was changed from true
to false
, successfully toggling the Boolean value.
While this method works, it’s not a common or recommended practice. Using the logical NOT
(!
) operator or the XOR
operator (^
) is more readable and idiomatic in Java for toggling Boolean values.
Numeric conversion for this purpose might be confusing and less clear in terms of code intent, but it still gets the job done.
Using the Bitwise NOT
(~
) Operator to Toggle a Boolean Variable in Java
The bitwise NOT
operator in Java is represented by the tilde (~
). It’s a unary operator that flips the bits of its operand.
When used with Boolean values, it flips each bit of the underlying binary representation, effectively toggling between 0
and 1
.
Here’s how to use the bitwise NOT
operator to toggle a Boolean value in Java:
public class Main {
public static void main(String[] args) {
boolean myBoolean = true;
System.out.println("myBoolean value before: " + myBoolean);
// Convert boolean to integer representation (0 for false, 1 for true)
int intRepresentation = myBoolean ? 1 : 0;
// Toggle the integer representation using bitwise NOT
intRepresentation = ~intRepresentation;
// Convert back to boolean
myBoolean = intRepresentation == 1;
System.out.println("myBoolean value after: " + myBoolean);
}
}
Output:
myBoolean value before: true
myBoolean value after: false
In this example, myBoolean
is initially set to true
. Then, intRepresentation
is an integer representation of the Boolean value, where true
is represented as 1
and false
as 0
.
The bitwise NOT
operator (~
) is used to toggle the integer representation. The integer representation is then converted back to a Boolean by checking if it’s equal to 1
.
The result is displayed, showing the toggled Boolean value.
The bitwise NOT
(~
) operator is not typically used to toggle Boolean values directly because it works on integer types, and Boolean values are not represented as integers in Java. However, as seen in the example provided, you can still achieve a toggling effect by using an integer representation and converting it back to a Boolean.
Using the Bitwise XOR
(^=
) Operator to Toggle a Boolean Variable in Java
The bitwise XOR
(exclusive OR
) operator in Java is represented by the caret symbol (^
).
It performs a bitwise exclusive OR
operation on corresponding bits of two operands. The result is 1
, where the bits in the operands differ, and 0
, where they are the same.
Here’s a simple explanation using two bits as an example:
0 ^ 0
results in0
0 ^ 1
results in1
1 ^ 0
results in1
1 ^ 1
results in0
Although it is unconventional, using the bitwise XOR
operator to toggle a Boolean value in Java can be done by converting the Boolean value to its integer representation (0
for false
, 1
for true
). Here’s an example:
public class Main {
public static void main(String[] args) {
boolean myBoolean = true;
System.out.println("myBoolean value before: " + myBoolean);
// Convert boolean to integer representation (0 for false, 1 for true)
int intRepresentation = myBoolean ? 1 : 0;
// Toggle the integer representation using bitwise XOR
intRepresentation ^= 1;
// Convert back to boolean
myBoolean = intRepresentation == 1;
System.out.println("myBoolean value after: " + myBoolean);
}
}
Output:
myBoolean value before: true
myBoolean value after: false
In the example, the ternary operation converts the Boolean value to an integer representation where true
is represented as 1
and false
as 0
. Then, the bitwise XOR
operator is used to toggle the integer representation.
Finally, the integer representation is converted back to a Boolean by checking if it’s equal to 1
.
This method effectively toggles the Boolean value using the bitwise XOR
operator in conjunction with integer representation. However, it’s worth noting that using the bitwise XOR
operator to toggle a Boolean value in Java is uncommon since there are much simpler ways to do it.
Using the if-else
Loop to Toggle a Boolean Variable in Java
The if-else
condition is a control flow statement in Java that allows you to execute different blocks of code based on a specified condition. The basic syntax is as follows:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
Now, the if-else
condition can also be used to toggle a Boolean value in Java by checking its current state and then assigning the opposite value. Below is an example of using the if-else
condition to toggle a Boolean value in Java:
public class Main {
public static void main(String[] args) {
boolean myBoolean = true;
System.out.println("myBoolean value before: " + myBoolean);
if (myBoolean) {
myBoolean = false;
} else {
myBoolean = true;
}
System.out.println("myBoolean value after: " + myBoolean);
}
}
Output:
myBoolean value before: true
myBoolean value after: false
In the provided example, we start with a Boolean variable named myBoolean
set to true
. Then, the if-else
condition is used to check the current value of myBoolean
, and it toggles the value of myBoolean
depending on its initial value.
Finally, it prints the final value of myBoolean
after the toggling process.
Conclusion
This article explored various methods to toggle a Boolean variable in Java. It introduced the Boolean
class, which wraps around Boolean primitives, and discussed the use of static values like TRUE
and FALSE
.
The article then delved into different techniques for toggling Boolean values, including using the NOT
(!
) operator, the XOR
(^
) operator, the ternary operator, numeric conversion, the bitwise NOT
(~
) operator, the bitwise XOR
(^
) operator, and the if-else
condition. Each method was explained with examples, highlighting their application and demonstrating the resulting toggled Boolean values.
Overall, developers can choose from these methods based on their preference, readability, and specific use case requirements.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn