How to Use Printwriter in Java
-
Using
print()
ofPrintWriter
in Java -
Using
println()
ofPrintWriter
in Java -
Using
printf()
ofPrintWriter
in Java
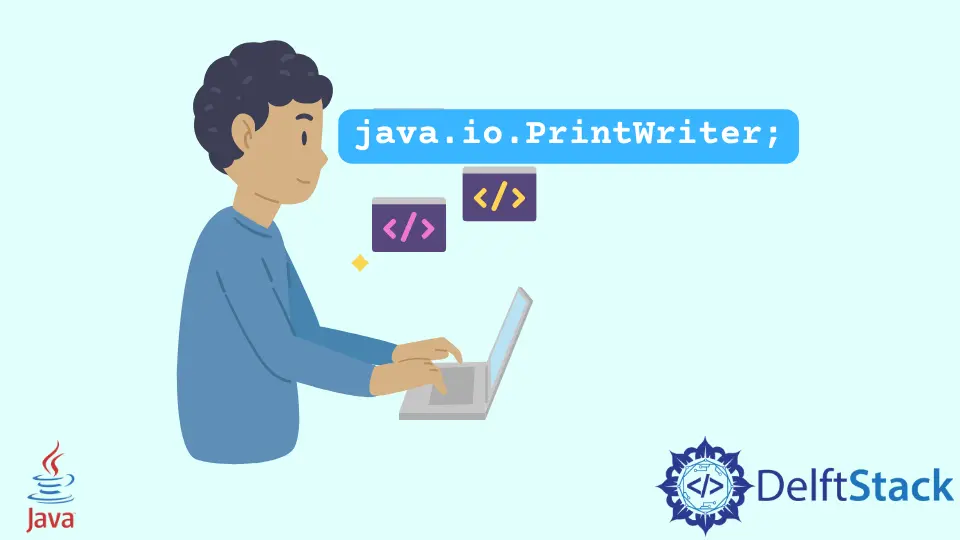
The PrintWriter
class was introduced in Java 7 that extends the Writer
class. PrintWriter
prints formatted representations of objects in a stream as a text output. In the following examples, we will use three common methods of the PrintWriter
class to print a string into a text file. Although we can print several types of objects, we are using string for our examples.
Using print()
of PrintWriter
in Java
We first use print()
that prints the given object in the text output stream. We create an object of the PrintWriter
class printWriter
and pass the file name with the extension. Now we call the print()
method and pass in the string that we want to print as an argument. At last, as the output stream should be closed once its work is over, we call printWriter.close()
.
The output shows the contents of test.txt
after running the program.
import java.io.FileNotFoundException;
import java.io.PrintWriter;
public class PrintWriterExample {
public static void main(String[] args) throws FileNotFoundException {
try {
PrintWriter printWriter = new PrintWriter("test.txt");
printWriter.print("Test PrintWriter Line 1 ");
printWriter.print("Test PrintWriter Line 2 ");
printWriter.print("Test PrintWriter Line 3");
printWriter.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output of test.txt
:
Test PrintWriter Line 1 Test PrintWriter Line 2 Test PrintWriter Line 3
Using println()
of PrintWriter
in Java
The only issue with the print()
method is that it prints the objects in a single line but println()
solves this problem. The println()
method prints the objects and breaks the line once it sees the end of the line. In the below example, we create a PrintWriter
object and then create a loop to run three times and print the string with the value of i
.
Unlike the last example, the output is cleaner, and the line breaks after printing a line.
import java.io.FileNotFoundException;
import java.io.PrintWriter;
public class PrintWriterExample {
public static void main(String[] args) throws FileNotFoundException {
try {
PrintWriter printWriter = new PrintWriter("test.txt");
for (int i = 1; i < 4; i++) {
printWriter.println("This is Line no. " + i);
}
printWriter.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output of test.txt
:
This is Line no .1 This is Line no .2 This is Line no .3
Using printf()
of PrintWriter
in Java
In the last example, we use the printf()
function that formats the string using the given format string and arguments. In the program, we use printf()
and pass two arguments; the first is the string with the format placeholder %d
that denotes an integer, and the second argument is the value to replace the placeholder.
import java.io.FileNotFoundException;
import java.io.PrintWriter;
public class PrintWriterExample {
public static void main(String[] args) throws FileNotFoundException {
try {
PrintWriter printWriter = new PrintWriter("test.txt");
printWriter.printf("This is example no. %d", 2);
printWriter.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output of test.txt
:
This is example no .2
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn