How to Attempt to Invoke Virtual Method on a Null Object Reference Error in Java
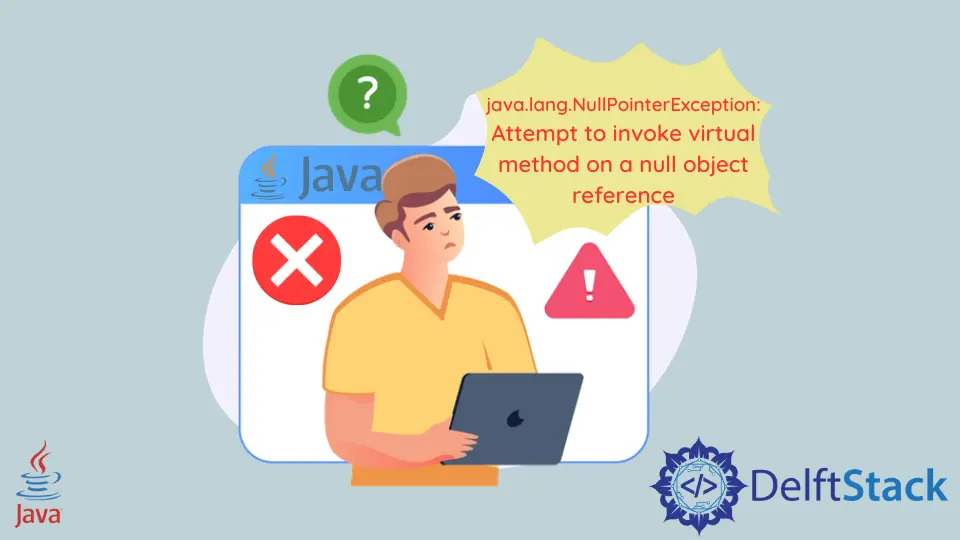
This tutorial demonstrates how to solve the Attempt to invoke virtual method on a null object reference
error in Java.
the Attempt to invoke virtual method on a null object reference
Error in Java
While working on an Android application, the error Attempt to invoke virtual method on a null object reference
can occur, a type of NullPointerException
. Whenever we try to invoke a method using a null object, it will throw this error.
Here is a system based on the Android platform, which throws the NullPointerException
with the attempt to invoke virtual method on a null object reference
description.
This example has three different Java classes.
Main_Player.java
:
package delftstack;
import javax.naming.Context;
public class Main_Player {
private Context AppContext;
private SharedPreferences SharedPreferencesSettingsU;
private SharedPreferences.Editor PreferencesEditorU;
private static final int PREFERENCE_MODE = 0;
private static final String UNIQUE_PREFERENCES_FILE = "DemoApp";
public Player(Context AppContext, String Player_Name) {
this.AppContext = AppContext;
Save_Name(Player_Name);
}
public void Save_Name(String nValue) {
SharedPreferencesSettingsU =
AppContext.getSharedPreferences(UNIQUE_PREFERENCES_FILE, PREFERENCE_MODE);
PreferencesEditorU = SharedPreferencesSettingsU.edit();
PreferencesEditorU.putString("keyName", nValue);
PreferencesEditorU.commit();
}
public String Get_Name(Context DemoContext) {
SharedPreferencesSettingsU =
DemoContext.getSharedPreferences(UNIQUE_PREFERENCES_FILE, PREFERENCE_MODE);
String PlayerName = SharedPreferencesSettingsU.getString("keyName", "ANONYMOUS");
return PlayerName;
}
}
Player_Name.java
:
package delftstack;
import javax.naming.Context;
public class Player_Name extends Activity {
private EditText Player_Name;
private Player M_Player;
public static Context AppContext;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.player_name);
AppContext = this;
Player_Name = (EditText) findViewById(R.id.etName);
}
public void onC_Confirm(View btnclick) {
M_Player = new Player(AppContext, String.valueOf(Player_Name.getText()));
// M_Player.saveName();
Intent intent = new Intent(Player_Name.this, Game_Play.class);
startActivity(intent);
}
public void onC_testShPref(View btnclick) {
Intent intent = new Intent(Player_Name.this, Game_Play.class);
startActivity(intent);
}
Game_Play.java
:
public class Game_Play extends Activity {
private TextView Welcome_Player;
private ListView Games_Created;
private Player M_Player;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.play_game);
Welcome_Player = (TextView) findViewById(R.id.tvPlayer_Name);
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
Games_Created = (ListView) findViewById(R.id.listCreatedGames);
Games_Created.setEmptyView(findViewById(R.id.tvNoGames));
}
}
We run the above system on an Android platform, which will throw an error caused by the java.lang.NullPointerException: Attempt to invoke virtual method
.
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'java.lang.String plp.cs4b.thesis.drawitapp.Main_Player.getName()' on a null object reference
at plp.cs4b.thesis.drawitapp.Game_Play.onCreate(Game_Play.java:20)
at android.app.Activity.performCreate(Activity.java:5933)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1105)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2251)
... 10 more
The error occurs in the below code line in the class Game_Play.java
.
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
This error occurs because the M_Player
comes as Null
. After all, it is not initialized. By initializing, we can solve that issue.
See the solution:
Game_Play.java
:
package delftstack;
public class Game_Play extends Activity {
private TextView Welcome_Player;
private ListView Games_Created;
// initialize the M_Player
private Player M_Player = new Main_Player(AppContext, "");
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.play_game);
Welcome_Player = (TextView) findViewById(R.id.tvPlayer_Name);
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
Games_Created = (ListView) findViewById(R.id.listCreatedGames);
Games_Created.setEmptyView(findViewById(R.id.tvNoGames));
}
}
So whenever working on a system like this, you must ensure that a method is not invoking any null
value; otherwise, it will throw this exception.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook