Java에서 Null 개체 참조 오류에 대한 가상 메서드 호출 시도
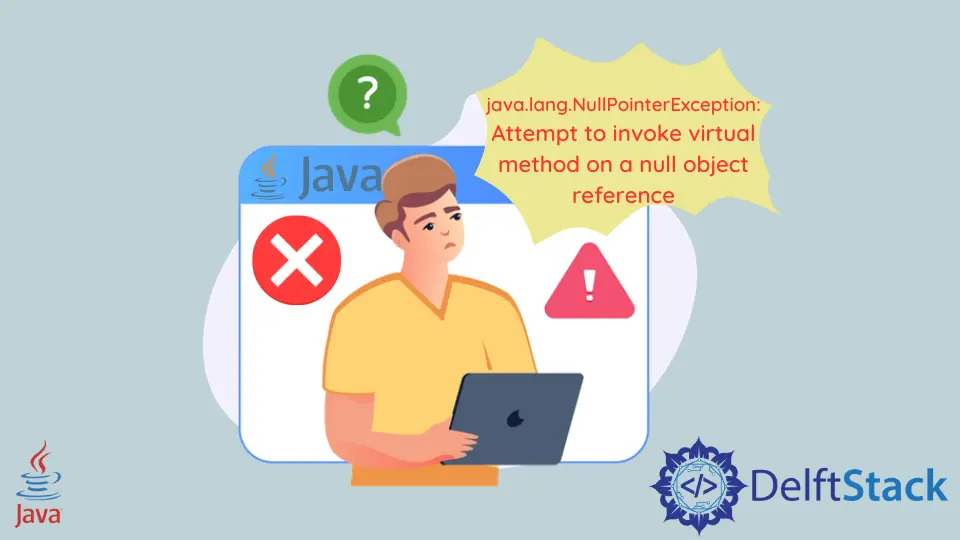
이 자습서는 Java에서 널 개체 참조에 대한 가상 메서드 호출 시도
오류를 해결하는 방법을 보여줍니다.
Java의 null 개체 참조에서 가상 메서드 호출 시도
오류
Android 애플리케이션에서 작업하는 동안 NullPointerException
유형인 Null 개체 참조에서 가상 메서드 호출 시도
오류가 발생할 수 있습니다. null 개체를 사용하여 메서드를 호출하려고 할 때마다 이 오류가 발생합니다.
다음은 null 개체 참조에서 가상 메서드 호출 시도
설명과 함께 NullPointerException
을 발생시키는 Android 플랫폼 기반 시스템입니다.
이 예제에는 세 가지 Java 클래스가 있습니다.
Main_Player.java
:
package delftstack;
import javax.naming.Context;
public class Main_Player {
private Context AppContext;
private SharedPreferences SharedPreferencesSettingsU;
private SharedPreferences.Editor PreferencesEditorU;
private static final int PREFERENCE_MODE = 0;
private static final String UNIQUE_PREFERENCES_FILE = "DemoApp";
public Player(Context AppContext, String Player_Name) {
this.AppContext = AppContext;
Save_Name(Player_Name);
}
public void Save_Name(String nValue) {
SharedPreferencesSettingsU =
AppContext.getSharedPreferences(UNIQUE_PREFERENCES_FILE, PREFERENCE_MODE);
PreferencesEditorU = SharedPreferencesSettingsU.edit();
PreferencesEditorU.putString("keyName", nValue);
PreferencesEditorU.commit();
}
public String Get_Name(Context DemoContext) {
SharedPreferencesSettingsU =
DemoContext.getSharedPreferences(UNIQUE_PREFERENCES_FILE, PREFERENCE_MODE);
String PlayerName = SharedPreferencesSettingsU.getString("keyName", "ANONYMOUS");
return PlayerName;
}
}
Player_Name.java
:
package delftstack;
import javax.naming.Context;
public class Player_Name extends Activity {
private EditText Player_Name;
private Player M_Player;
public static Context AppContext;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.player_name);
AppContext = this;
Player_Name = (EditText) findViewById(R.id.etName);
}
public void onC_Confirm(View btnclick) {
M_Player = new Player(AppContext, String.valueOf(Player_Name.getText()));
// M_Player.saveName();
Intent intent = new Intent(Player_Name.this, Game_Play.class);
startActivity(intent);
}
public void onC_testShPref(View btnclick) {
Intent intent = new Intent(Player_Name.this, Game_Play.class);
startActivity(intent);
}
Game_Play.java
:
public class Game_Play extends Activity {
private TextView Welcome_Player;
private ListView Games_Created;
private Player M_Player;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.play_game);
Welcome_Player = (TextView) findViewById(R.id.tvPlayer_Name);
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
Games_Created = (ListView) findViewById(R.id.listCreatedGames);
Games_Created.setEmptyView(findViewById(R.id.tvNoGames));
}
}
Android 플랫폼에서 위의 시스템을 실행하면 java.lang.NullPointerException: Attempt to invoke virtual method
로 인해 오류가 발생합니다.
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'java.lang.String plp.cs4b.thesis.drawitapp.Main_Player.getName()' on a null object reference
at plp.cs4b.thesis.drawitapp.Game_Play.onCreate(Game_Play.java:20)
at android.app.Activity.performCreate(Activity.java:5933)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1105)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2251)
... 10 more
Game_Play.java
클래스의 아래 코드 라인에서 오류가 발생합니다.
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
이 오류는 M_Player
가 Null
로 오기 때문에 발생합니다. 결국 초기화되지 않습니다. 초기화하면 이 문제를 해결할 수 있습니다.
솔루션 보기:
Game_Play.java
:
package delftstack;
public class Game_Play extends Activity {
private TextView Welcome_Player;
private ListView Games_Created;
// initialize the M_Player
private Player M_Player = new Main_Player(AppContext, "");
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.play_game);
Welcome_Player = (TextView) findViewById(R.id.tvPlayer_Name);
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
Games_Created = (ListView) findViewById(R.id.listCreatedGames);
Games_Created.setEmptyView(findViewById(R.id.tvNoGames));
}
}
따라서 이와 같은 시스템에서 작업할 때마다 메서드가 null
값을 호출하지 않도록 해야 합니다. 그렇지 않으면 이 예외가 발생합니다.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook