Java で Null オブジェクト参照エラーに対して仮想メソッドを呼び出そうとする
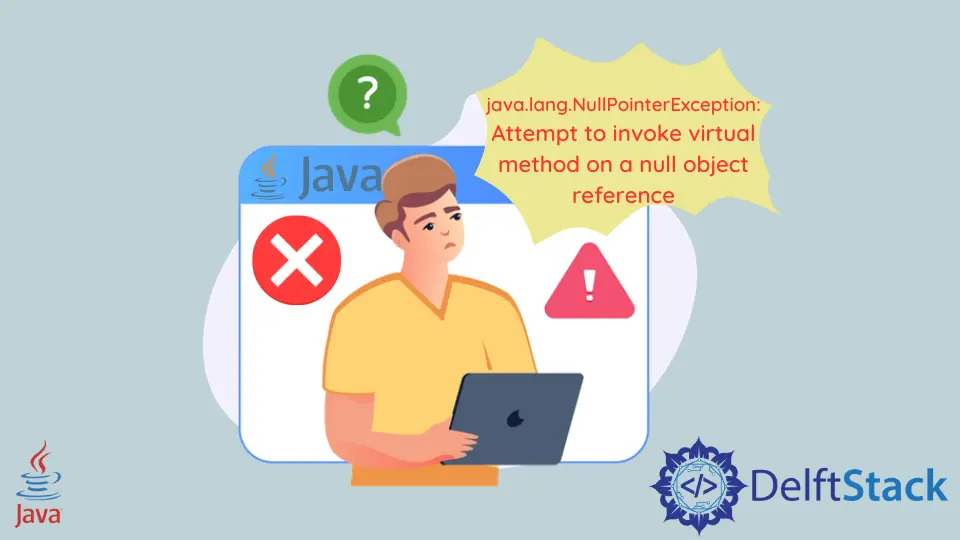
このチュートリアルでは、Java での null オブジェクト参照で仮想メソッドを呼び出そうとしています
エラーを解決する方法を示します。
null オブジェクト参照で仮想メソッドを呼び出そうとしています
Java のエラー
Android アプリケーションでの作業中に、null オブジェクト参照で仮想メソッドを呼び出そうとしています
というエラーが発生する可能性があります。これは、NullPointerException
のタイプです。 null オブジェクトを使用してメソッドを呼び出そうとすると、常にこのエラーがスローされます。
これは Android プラットフォームに基づくシステムで、null オブジェクト参照で仮想メソッドを呼び出そうとする試み
の説明で NullPointerException
をスローします。
この例には、3つの異なる Java クラスがあります。
Main_Player.java
:
package delftstack;
import javax.naming.Context;
public class Main_Player {
private Context AppContext;
private SharedPreferences SharedPreferencesSettingsU;
private SharedPreferences.Editor PreferencesEditorU;
private static final int PREFERENCE_MODE = 0;
private static final String UNIQUE_PREFERENCES_FILE = "DemoApp";
public Player(Context AppContext, String Player_Name) {
this.AppContext = AppContext;
Save_Name(Player_Name);
}
public void Save_Name(String nValue) {
SharedPreferencesSettingsU =
AppContext.getSharedPreferences(UNIQUE_PREFERENCES_FILE, PREFERENCE_MODE);
PreferencesEditorU = SharedPreferencesSettingsU.edit();
PreferencesEditorU.putString("keyName", nValue);
PreferencesEditorU.commit();
}
public String Get_Name(Context DemoContext) {
SharedPreferencesSettingsU =
DemoContext.getSharedPreferences(UNIQUE_PREFERENCES_FILE, PREFERENCE_MODE);
String PlayerName = SharedPreferencesSettingsU.getString("keyName", "ANONYMOUS");
return PlayerName;
}
}
Player_Name.java
:
package delftstack;
import javax.naming.Context;
public class Player_Name extends Activity {
private EditText Player_Name;
private Player M_Player;
public static Context AppContext;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.player_name);
AppContext = this;
Player_Name = (EditText) findViewById(R.id.etName);
}
public void onC_Confirm(View btnclick) {
M_Player = new Player(AppContext, String.valueOf(Player_Name.getText()));
// M_Player.saveName();
Intent intent = new Intent(Player_Name.this, Game_Play.class);
startActivity(intent);
}
public void onC_testShPref(View btnclick) {
Intent intent = new Intent(Player_Name.this, Game_Play.class);
startActivity(intent);
}
Game_Play.java
:
public class Game_Play extends Activity {
private TextView Welcome_Player;
private ListView Games_Created;
private Player M_Player;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.play_game);
Welcome_Player = (TextView) findViewById(R.id.tvPlayer_Name);
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
Games_Created = (ListView) findViewById(R.id.listCreatedGames);
Games_Created.setEmptyView(findViewById(R.id.tvNoGames));
}
}
上記のシステムを Android プラットフォームで実行すると、java.lang.NullPointerException: Attempt to invoke virtual method
が原因でエラーがスローされます。
Caused by: java.lang.NullPointerException: Attempt to invoke virtual method 'java.lang.String plp.cs4b.thesis.drawitapp.Main_Player.getName()' on a null object reference
at plp.cs4b.thesis.drawitapp.Game_Play.onCreate(Game_Play.java:20)
at android.app.Activity.performCreate(Activity.java:5933)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1105)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2251)
... 10 more
クラスGame_Play.java
の以下のコード行でエラーが発生します。
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
このエラーは、M_Player
が Null
になっているために発生します。 結局のところ、それは初期化されていません。 初期化することで、その問題を解決できます。
解決策を参照してください。
Game_Play.java
:
package delftstack;
public class Game_Play extends Activity {
private TextView Welcome_Player;
private ListView Games_Created;
// initialize the M_Player
private Player M_Player = new Main_Player(AppContext, "");
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.play_game);
Welcome_Player = (TextView) findViewById(R.id.tvPlayer_Name);
Welcome_Player.setText("Welcome Back Player, " + String.valueOf(M_Player.getName(this)) + " !");
Games_Created = (ListView) findViewById(R.id.listCreatedGames);
Games_Created.setEmptyView(findViewById(R.id.tvNoGames));
}
}
したがって、このようなシステムで作業するときはいつでも、メソッドが null
値を呼び出していないことを確認する必要があります。 そうでない場合、この例外がスローされます。
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook