Java での無効な入力例外
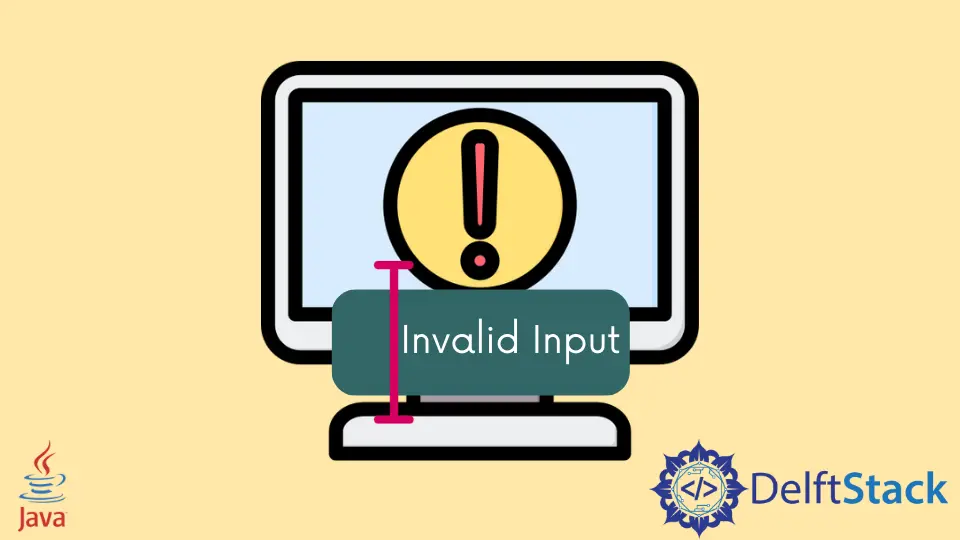
Java は Invalid Input
という名前の例外を提供しませんが、一部の IO 例外とランタイム例外を使用して無効な入力を処理できます。 状況に応じて IO 例外から取得したり、無効な入力に対して ユーザー定義の例外 を作成したりできます。
このチュートリアルでは、Java で無効な入力に対して例外を使用する方法を示します。
Javaで入力引数が無効な場合
メソッドを操作するときに、無効な入力引数を入れると、IllegalArgumentException
がキャッチされることがあります。 以下の例は、Java での IllegalArgumentException
を示しています。
package delftstack;
public class Invalid_Argument {
public static void main(String[] args) {
Thread Demo_Thread = new Thread(new Runnable() {
public void run() {
try {
Thread.sleep(-100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Hello! This is delftstack.com");
}
});
Demo_Thread.setName("Delftstack Thread");
Demo_Thread.start();
}
}
上記のコードは、Thread.sleep()
への入力が無効であるため、IllegalArgumentException
をスローします。 出力を参照してください:
Exception in thread "Delftstack Thread" java.lang.IllegalArgumentException: timeout value is negative
at java.base/java.lang.Thread.sleep(Native Method)
at delftstack.Invalid_Argument$1.run(Invalid_Argument.java:10)
at java.base/java.lang.Thread.run(Thread.java:833)
Javaで入力がnullの場合
null 値がオブジェクトに代入されるか、null 入力を操作しようとすると、NullPointerException
がスローされます。 また、無効入力例外としても使用できます。
例を参照してください:
package delftstack;
import java.io.*;
public class Null_Invalid {
public static void main(String[] args) {
String Demo = null;
try {
if (Demo.equals("delftstack"))
System.out.print("The Strings are equal");
else
System.out.print("The Strings are not equal");
} catch (NullPointerException e) {
System.out.print("The String is null");
e.printStackTrace();
}
}
}
上記のコードは、null 文字列の使用が無効になる可能性があることを示しています。 null 文字列が別の文字列と比較されるため、このコードは NullPointerException
をスローします。
例を参照してください:
The String is null
java.lang.NullPointerException: Cannot invoke "String.equals(Object)" because "Demo" is null
at delftstack.Null_Invalid.main(Null_Invalid.java:9)
Java での無効な入力に対するユーザー定義の例外
ユーザーが ID を入力した場合の例外を作成しましょう。 データベースに存在しない場合は、無効な ID 例外がスローされます。
例を参照してください:
package delftstack;
import java.util.*;
class InValid_ID extends Exception {
public InValid_ID(String ID) {
super(ID);
}
}
public class Invalid_Input {
// Method to find ID
static void find_ID(int input_array[], int ID) throws InValid_ID {
boolean condition = false;
for (int i = 0; i < input_array.length; i++) {
if (ID == input_array[i]) {
condition = true;
}
}
if (!condition) {
throw new InValid_ID("The ID is you Entered is InValid!");
} else {
System.out.println("The ID is you Entered is Valid!");
}
}
public static void main(String[] args) {
Scanner new_id = new Scanner(System.in);
System.out.print("Enter the ID number: ");
int ID = new_id.nextInt();
try {
int Input_Array[] = new int[] {123, 124, 134, 135, 145, 146};
find_ID(Input_Array, ID);
} catch (InValid_ID e) {
System.out.println(e);
e.printStackTrace();
}
}
}
このコードは、入力 ID が無効な場合、つまりデータベースに存在しない場合にスローされる例外を作成します。 同様に、条件に基づいて例外を作成できます。
出力:
Enter the ID number: 122
delftstack.InValid_ID: The ID is you Entered is InValid!
delftstack.InValid_ID: The ID is you Entered is InValid!
at delftstack.Invalid_Input.find_ID(Invalid_Input.java:20)
at delftstack.Invalid_Input.main(Invalid_Input.java:33)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook