Associative Array in Java
- Implement Associative Array and Add an Element
- Remove Element From the Associative Array
- Traverse Elements of the Associative Array in Java
- Associative Array in Java
- Conclusion
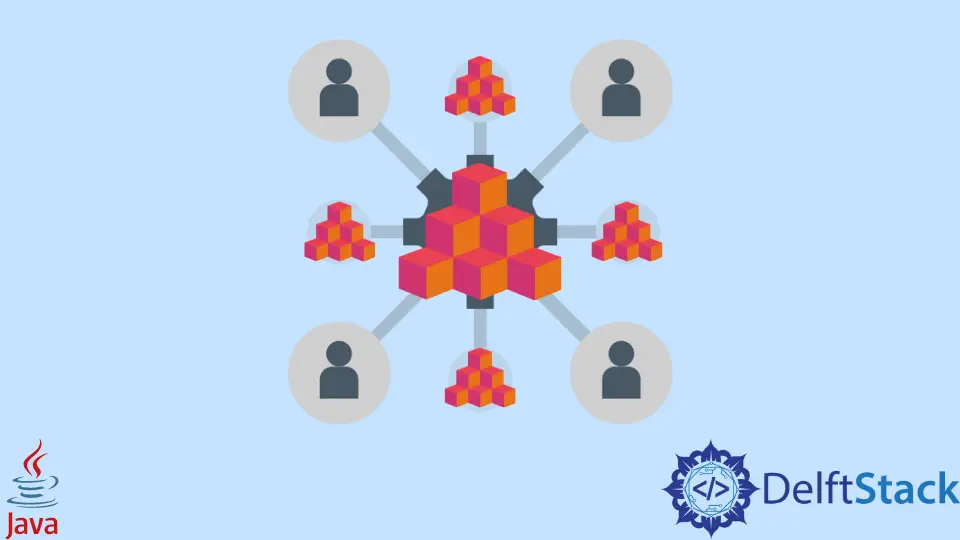
An associative array is a type of array that stores the set of elements in key and value pairs. It is a collection of keys and values where the key is unique and is associated with one value.
In Java, an associative array is implemented using the Map
interface and its various implementations, with the most common one being HashMap
. Also referred to as a map or dictionary, an associative array allows developers to store and retrieve values based on unique keys.
Each key is associated with a specific value, enabling efficient data retrieval. The Map
interface provides methods like put(key, value)
for adding elements, get(key)
for retrieving values, and remove(key)
for deleting entries.
This data structure is invaluable for scenarios where data needs to be organized and accessed based on specific identifiers, providing a flexible and dynamic way to manage key-value pairs in Java programs.
Implement Associative Array and Add an Element
To implement and add an associative array using HashMaps
in Java, you first create a HashMap
object by specifying the data types for the keys and values.
In Java, the implementation of an associative array is typically done using the Map
interface and its various implementations, such as HashMap
. Here’s the basic syntax for creating and adding elements to an associative array using a HashMap
:
import java.util.HashMap;
import java.util.Map;
public class AssociativeArrayExample {
public static void main(String[] args) {
// Create a HashMap with specified key and value types
Map<KeyType, ValueType> associativeArray = new HashMap<>();
// Add elements to the associative array
associativeArray.put(key1, value1);
associativeArray.put(key2, value2);
// ... add more key-value pairs as needed
}
}
In Java, the basic syntax for implementing an associative array involves declaring a variable of type Map<KeyType, ValueType>
, where KeyType
represents the data type for keys (e.g., String
, Integer
) and ValueType
represents the data type for values (e.g., Integer
, Double
).
You then create an instance of a Map
implementation, such as HashMap
, by using new HashMap<>()
. Subsequently, you can add key-value pairs using the put
method, specifying the key and its corresponding value.
You would replace key1
, key2
, value1
, value2
, etc., with the actual values you want to store in the associative array. The put
method is used to add key-value pairs to the HashMap
.
This basic syntax provides a foundation for creating and populating an associative array in Java using the HashMap
implementation.
By repeating this process for different keys and values, you build your associative array. Here’s a concise example:
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap
Map<String, Integer> associativeArray = new HashMap<>();
// Add elements to the associative array
associativeArray.put("One", 1);
associativeArray.put("Two", 2);
associativeArray.put("Three", 3);
System.out.println(associativeArray);
}
}
In this example, the HashMap
associativeArray
is created to store String keys and Integer values, and three key-value pairs are added using the put
method. This establishes the foundation for an associative array, allowing efficient retrieval of values based on their corresponding keys.
Remove Element From the Associative Array
In Java, removing an element from an associative array is straightforward using the remove
method provided by the Map
interface.
First, you need to identify the key of the element you want to remove. Once the key is known, you can call the remove(key)
method on the Map
object, specifying the key of the element you wish to delete.
Example:
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap
Map<String, Integer> associativeArray = new HashMap<>();
// Add elements to the associative array
associativeArray.put("One", 1);
associativeArray.put("Two", 2);
associativeArray.put("Three", 3);
System.out.println(associativeArray);
// Remove an element from the associative array
associativeArray.remove("Two");
System.out.println(associativeArray);
}
}
This simple syntax allows you to efficiently eliminate specific elements from the associative array based on their keys.
Traverse Elements of the Associative Array in Java
Traversing elements of an associative array in Java is crucial for efficiently working with and processing the stored data. It allows developers to iterate through the key-value pairs, enabling operations such as data analysis, manipulation, or retrieval.
By traversing the associative array, you can access and utilize the values associated with specific keys, making it essential for tasks like searching for elements, performing calculations, or generating specific outputs. Different traversal methods, such as using keySet()
, entrySet()
, forEach()
, or values()
provide flexibility in accessing and processing the data based on the requirements of the program.
Traverse Elements of the Associative Array Using keySet()
In Java, traversing elements of an associative array using keySet()
is a straightforward and efficient process. The keySet()
method, provided by the Map
interface, returns a set containing all the keys present in the associative array.
By iterating over this set using a loop, such as a for-each
loop, you can access each key and use it to retrieve the corresponding values from the associative array. This method is particularly useful when you need to perform operations on both keys and values simultaneously.
For example, if you have a HashMap
named associativeArray
, you can traverse its elements using for (KeyType key : associativeArray.keySet())
and then access the associated values using associativeArray.get(key)
.
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap
Map<String, Integer> associativeArray = new HashMap<>();
// Add elements to the associative array
associativeArray.put("One", 1);
associativeArray.put("Two", 2);
associativeArray.put("Three", 3);
// Traverse the associative array using keySet()
System.out.println("Traversing Associative Array using keySet():");
for (String key : associativeArray.keySet()) {
System.out.println("Key: " + key + ", Value: " + associativeArray.get(key));
}
}
}
This approach ensures a clear and organized way to process the contents of the associative array based on its keys.
Traverse Elements of the Associative Array Using entrySet()
and Iterator
The entrySet()
method, available in the Map
interface, returns a set view of the key-value pairs (entries) in the associative array. By obtaining an iterator from this set using iterator()
, you can sequentially access each entry.
The iterator’s hasNext()
method checks if there are more entries, and next()
retrieves the next entry in the iteration. Each entry can then be accessed using entry.getKey()
for the key and entry.getValue()
for the corresponding value.
This method is advantageous when you need to perform specific operations on both keys and values during traversal.
For instance, if you have a HashMap
named associativeArray
, you can traverse its elements using Iterator<Map.Entry<KeyType, ValueType>> iterator = associativeArray.entrySet().iterator()
.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap
Map<String, Integer> associativeArray = new HashMap<>();
// Add elements to the associative array
associativeArray.put("One", 1);
associativeArray.put("Two", 2);
associativeArray.put("Three", 3);
// Traverse the associative array using entrySet() and Iterator
System.out.println("Traversing Associative Array using entrySet() and Iterator:");
Iterator<Map.Entry<String, Integer>> iterator = associativeArray.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
}
}
This approach facilitates efficient processing of key-value pairs in a clear and organized manner.
Traverse Elements of the Associative Array Using forEach()
Method
The forEach()
method, introduced in Java 8 and available in the Map
interface, accepts a lambda expression that defines the action to be performed on each key-value pair. When applied to the result of entrySet()
, it iterates through all entries, and the lambda expression specifies the behavior for each entry, including accessing the key and value.
For example, if you have a HashMap
named associativeArray
, you can traverse its elements using associativeArray.forEach((key, value) -> {/* Your logic here */})
.
Example:
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap
Map<String, Integer> associativeArray = new HashMap<>();
// Add elements to the associative array
associativeArray.put("One", 1);
associativeArray.put("Two", 2);
associativeArray.put("Three", 3);
// Traverse the associative array using forEach() method
System.out.println("Traversing Associative Array using forEach() method:");
associativeArray.forEach(
(key, value) -> System.out.println("Key: " + key + ", Value: " + value));
}
}
This method simplifies the syntax for iterating through the associative array and is particularly useful for concise operations on both keys and values. Combining it with lambda expressions enhances code readability and reduces boilerplate, making the traversal process more streamlined and intuitive.
Traverse Elements of the Associative Array Using for
Loop and values()
The values()
method, part of the Map
interface, returns a collection view of all the values in the associative array. By using this method in conjunction with a for-each
loop, you can iterate through each value in the collection.
For example, if you have a HashMap
named associativeArray
, you can traverse its elements using for (ValueType value : associativeArray.values())
. This method is useful when the primary interest is in the values, and direct access to keys is not necessary for the operation at hand.
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap
Map<String, Integer> associativeArray = new HashMap<>();
// Add elements to the associative array
associativeArray.put("One", 1);
associativeArray.put("Two", 2);
associativeArray.put("Three", 3);
// Traverse the associative array using for loop and values()
System.out.println("Traversing Associative Array using for loop and values():");
for (int value : associativeArray.values()) {
System.out.println("Value: " + value);
}
}
}
It provides a concise way to iterate through and manipulate the values within the associative array, simplifying code and enhancing readability for tasks that involve working primarily with the stored data values.
Associative Array in Java
Associative arrays play a crucial role in programming by allowing developers to associate a value with a unique key. In Java, one of the most versatile and widely used implementations of associative arrays is through the HashMap
class.
Associative arrays, also known as maps or dictionaries, are data structures that store key-value pairs. Each key is unique, and the associated value can be retrieved efficiently.
HashMaps
, part of Java’s Collections framework, provide a convenient and efficient way to implement associative arrays.
Let’s dive into a complete code example that demonstrates the creation, addition, removal, and traversal of elements in a HashMap
.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap
Map<String, Integer> associativeArray = new HashMap<>();
// Add elements to the associative array
associativeArray.put("One", 1);
associativeArray.put("Two", 2);
associativeArray.put("Three", 3);
// Display the associative array after addition
System.out.println("Associative Array after Addition: " + associativeArray);
// Remove an element from the associative array
associativeArray.remove("Two");
// Display the associative array after removal
System.out.println("Associative Array after Removal: " + associativeArray);
// Traverse the associative array using various methods
System.out.println("\nTraversing Associative Array:");
// Method 1: Using keySet()
System.out.println("\nTraversing Associative Array using ketSet()");
for (String key : associativeArray.keySet()) {
System.out.println("Key: " + key + ", Value: " + associativeArray.get(key));
}
// Method 2: Using Iterator
System.out.println("\nTraversing Associative Array using entrySet() and iterator");
Iterator<Map.Entry<String, Integer>> iterator = associativeArray.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
// Method 3: Using forEach() method
System.out.println("\nTraversing Associative Array using value()");
associativeArray.forEach(
(key, value) -> System.out.println("Key: " + key + ", Value: " + value));
}
}
To create a HashMap
in Java, we start by importing the required classes and initializing a new HashMap
instance, often named associativeArray
.
To add key-value pairs to the HashMap
, the put
method is employed. This method allows us to associate a specific value with a given key in the associative array.
For removing an element from the HashMap
, we utilize the remove
method. By specifying the key of the element to be removed, we can efficiently eliminate entries from the associative array.
There are three methods to traverse a HashMap
in Java.
The first method, using keySet()
, involves obtaining a set of keys from the HashMap
. This set allows iteration through the keys, providing access to their corresponding values.
The second method, using Iterator
, employs an iterator obtained from the entrySet()
method. This iterator allows simultaneous traversal of both keys and values, offering flexibility in handling key-value pairs.
The third method, using forEach()
method, introduced in Java 8, enables concise iteration through the HashMap
by applying a specified action to each key-value pair. This modern approach enhances code readability and simplifies the traversal process, making it an effective choice for working with associative arrays in Java.
Conclusion
The provided code illustrates the fundamental operations associated with HashMaps
in Java. By creating, adding, removing, and traversing elements, developers can harness the power of associative arrays for efficient data storage and retrieval.