Matriz asociativa en Java
- Utilice la matriz asociativa en Java
- Resumen de la matriz asociativa en Java
- Implementar una matriz asociativa en Java
- Crear una matriz asociativa en Java
- Agregar elementos a la matriz asociativa en Java
- Elementos transversales de la matriz asociativa en Java
-
Elementos transversales de la matriz asociativa utilizando el método
forEach()
en Java 8
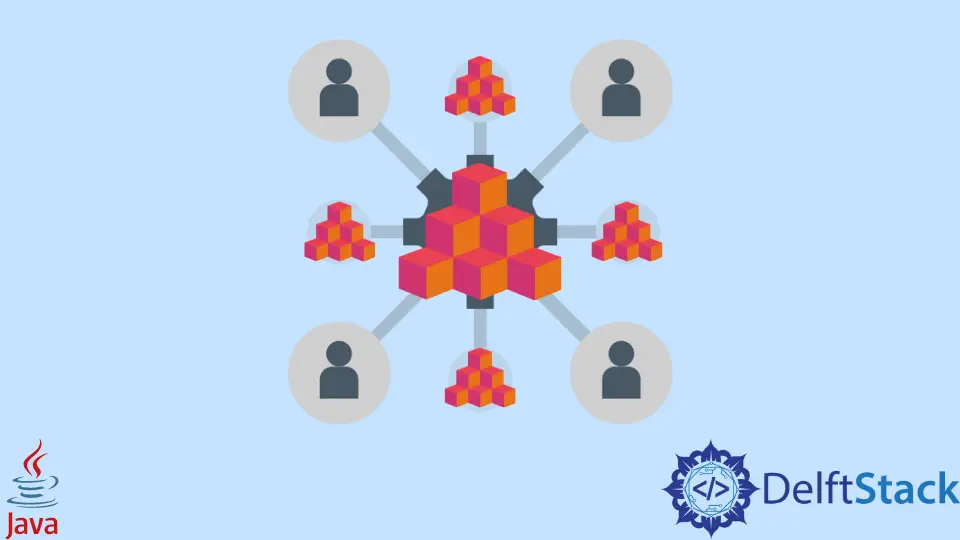
Una matriz asociativa es un tipo de matriz que almacena el conjunto de elementos en pares de clave
y valor
. Es una colección de claves y valores donde la clave es única y está asociada con un valor.
Si tenemos que acceder al elemento desde la matriz asociativa, debemos llamar al nombre de la matriz y pasar la clave a cuyo valor queremos acceder
.
Utilice la matriz asociativa en Java
Por ejemplo, tenemos una matriz denominada marcas que almacena el número de lista y las calificaciones de los estudiantes.
Entonces, si tenemos que acceder a la nota de un estudiante en particular, entonces podemos llamar así a las marcas 105
, donde las notas son el nombre de una matriz y 105
es el número de registro del estudiante, no un número de índice que es no es posible en una matriz si estamos usando el lenguaje Java.
Por lo tanto, la matriz asociativa no es compatible con Java, pero podemos lograrlo fácilmente usando HashMap
. Java no admite matrices asociativas, pero se puede implementar mediante Map.
Resumen de la matriz asociativa en Java
HashMap<String, String> hashmap = new HashMap<>();
// method to add the key,value pair in hashmap
hashmap.put("Key1", "Value1");
hashmap.put("Key2", "Value2");
hashmap.put("Key3", "Value3");
// and many more...
// get the value 1 and 2
System.out.println(hashmap.get("Key1"));
System.out.println(hashmap.get("Key2"));
// and many more...
Implementar una matriz asociativa en Java
Para implementar una matriz asociativa en Java, usamos HashMap
, una clase de implementación de la interfaz Map. Entendamos paso a paso.
Primero, importe e inicialice el HashMap
, es decir, cree una instancia de HashMap usando las siguientes declaraciones.
import java.util.HashMap;
HashMap<String, String> hashmap = new HashMap<>();
Luego, utilizando el método put()
, agregue el valor clave al HashMap
.
hashmap.put("Key1", "Value1");
Convierta el HashMap
a Set usando el método entrySet()
para eliminar claves duplicadas.
Set<Map.Entry<String, String> > set = map.entrySet();
Convierta el conjunto en una ArrayList
, que es una matriz que queremos.
List<Map.Entry<String, String>> list = new ArrayList<>(set);
Crear una matriz asociativa en Java
En este ejemplo, usamos la clase HashMap
para implementar la matriz asociativa en Java.
Mira, contiene datos en formato de par clave-valor, y usamos el método getKey()
para acceder a la clave y el método getValue()
para acceder a los valores.
import java.io.*;
import java.util.*;
public class SimpleTesting {
public static void main(String[] args) {
HashMap<String, String> hashmap = new HashMap<>();
hashmap.put("Virat", "Batsman");
hashmap.put("Bumrah", "Bowler");
hashmap.put("Jadeja", "All-rounder");
hashmap.put("Pant", "Wicket-Keeper");
Set<Map.Entry<String, String>> s = hashmap.entrySet();
List<Map.Entry<String, String>> array = new ArrayList<>(s);
for (int i = 0; i < array.size(); i++) {
System.out.println(array.get(i).getKey() + " is " + array.get(i).getValue());
}
}
}
Producción :
Pant is Wicket-Keeper
Jadeja is All-rounder
Bumrah is Bowler
Virat is Batsman
Como ya hemos discutido, esa clave debe ser única. Si insertamos las mismas claves en la matriz asociativa, descartará uno de los pares clave-valor
.
Hemos insertado dos claves iguales, Virat
, en el siguiente código. Vea el ejemplo a continuación.
import java.io.*;
import java.util.*;
public class SimpleTesting {
public static void main(String[] args) {
HashMap<String, String> hashmap = new HashMap<>();
hashmap.put("Virat", "Batsman");
hashmap.put("Bumrah", "Bowler");
hashmap.put("Jadeja", "All-rounder");
hashmap.put("Pant", "Wicket-Keeper");
hashmap.put("Virat", "Captain");
Set<Map.Entry<String, String>> s = hashmap.entrySet();
List<Map.Entry<String, String>> array = new ArrayList<>(s);
for (int i = 0; i < array.size(); i++) {
System.out.println(array.get(i).getKey() + " is " + array.get(i).getValue());
}
}
}
Producción :
Pant is Wicket-Keeper
Jadeja is All-rounder
Bumrah is Bowler
Virat is Captain
Agregar elementos a la matriz asociativa en Java
Podemos agregar un elemento a una matriz en el mapa usando el método put()
. De manera similar, podemos eliminar un elemento de una matriz usando el método remove()
.
Podemos averiguar el tamaño de la matriz utilizando el método size()
.
import java.util.HashMap;
public class SimpleTesting {
public static void main(String[] args) {
HashMap<String, String> fruits = new HashMap<String, String>();
fruits.put("Apple", "Red");
fruits.put("Banana", "Yellow");
fruits.put("Guava", "Green");
fruits.put("Blackberries", "Purple");
System.out.println("The Size of fruits Map is : " + fruits.size());
// Remove Banana from the HashMap
fruits.remove("Banana");
// To find out the size of the Hashmap
System.out.println("The Size of fruits Map is : " + fruits.size());
// Check whether the key is present in the Hashmap or not
String fruit_key = "Apple";
if (fruits.containsKey(fruit_key)) {
System.out.println("The colour of " + fruit_key + " is: " + fruits.get(fruit_key));
} else {
System.out.println("There is no entry for the fruit of " + fruit_key);
}
}
}
Producción :
The Size of fruits Map is : 4
The Size of fruits Map is : 3
The colour of Apple is: Red
Elementos transversales de la matriz asociativa en Java
Podemos usar el bucle for-each
para recorrer la matriz asociativa. Dado que HashMap
pertenece al paquete java.util
, podemos usar el bucle foreach
para iterar sus elementos.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
HashMap<String, String> fruits = new HashMap<String, String>();
fruits.put("Apple", "Red");
fruits.put("Banana", "Yellow");
fruits.put("Guava", "Green");
fruits.put("Blackberries", "Purple");
System.out.println("The Size of fruits Map is : " + fruits.size());
for (Map.Entry element : fruits.entrySet()) {
String key = (String) element.getKey();
System.out.println(key + " : " + element.getValue());
}
}
}
Producción :
The Size of fruits Map is : 4
Guava : Green
Apple : Red
Blackberries : Purple
Banana : Yellow
Elementos transversales de la matriz asociativa utilizando el método forEach()
en Java 8
Si está trabajando con Java 8 o una versión superior, puede usar el método forEach()
para recorrer los elementos de la matriz. El método forEach()
requiere una expresión lambda
como argumento.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
HashMap<String, String> fruits = new HashMap<String, String>();
fruits.put("Apple", "Red");
fruits.put("Banana", "Yellow");
fruits.put("Guava", "Green");
fruits.put("Blackberries", "Purple");
System.out.println("The Size of fruits Map is : " + fruits.size());
fruits.forEach((k, v) -> System.out.println(k + " : " + v));
}
}
Producción :
The Size of fruits Map is : 4
Guava : Green
Apple : Red
Blackberries : Purple
Banana : Yellow
Este tutorial estudió que Java técnicamente no es compatible con la matriz asociativa, pero podemos lograrlo fácilmente usando HashMap
.