How to Convert ArrayList to Set in Java
- Convert ArrayList to HashSet Using Naive Approach in Java
- Convert ArrayList to HashSet Using Constructors in Java
- Convert ArrayList to HashSet Using Java 8 Stream API
-
Convert ArrayList to HashSet Using
Set.copyOf()
Method in Java - Convert ArrayList to HashSet Using Apache Commons Collection Library in Java
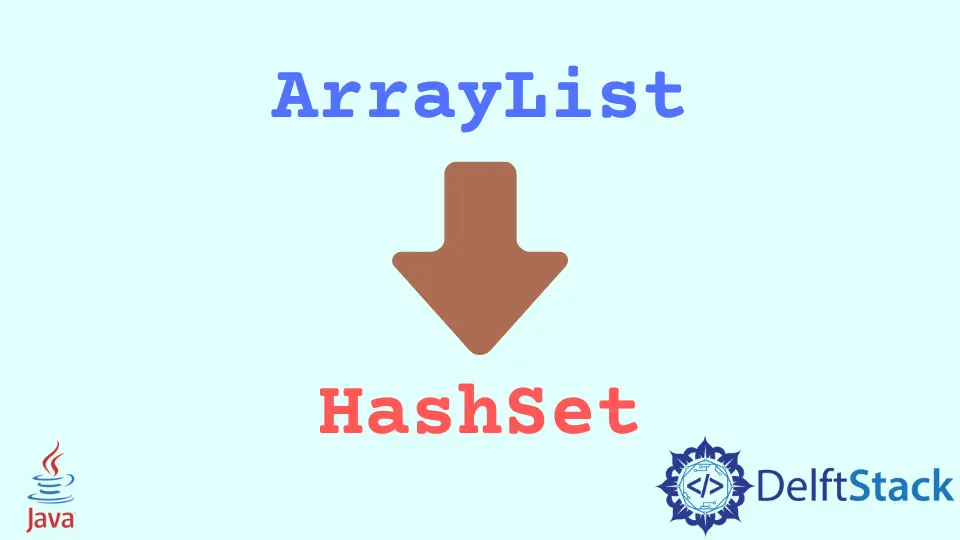
ArrayList is an implementation class of the List interface that is used to store data in linear order, whereas the set is an interface that has the HashSet class to store data. The major difference between these two is the HashSet does not allow duplicate elements and stores unique elements.
This tutorial will introduce different approaches to converting an ArrayList to a HashSet.
Convert ArrayList to HashSet Using Naive Approach in Java
This is the most basic approach to converting a list into a set.
In this approach, we first declare an empty set. We then loop through the list and add its element to the set one by one using the HashSet.add()
method. When the loop terminates, the list is converted into a set.
Look at the code below.
import java.util.*;
public class SimpleTesting {
public static void main(String args[]) {
// initialise a list
List<String> list =
Arrays.asList("Karan", "Rahul", "Jay", "Laxman", "Praful", "Kushagra", "Karan");
// intialise a set
Set<String> set = new HashSet<String>();
// apply for loop
for (String ele : list) {
set.add(ele);
}
// display the set
for (String ele : set) {
System.out.println(ele);
}
}
}
Output:
Rahul
Kushagra
Jay
Karan
Laxman
Praful
In the code above, we created a list that stores some names. We also initialize an empty Set named set.
We then loop through the list and add its elements to set one by one. At last, we print the set’s elements. Notice how the duplicate elements in the list are eliminated.
Convert ArrayList to HashSet Using Constructors in Java
This approach is the most simple of all the approaches. In this approach, we pass the list that needs to be converted into the constructor of the HashSet class.
This eliminates the need for a for
loop as was required in the previous approach. The constructor returns a new set having all the unique elements.
Look at the code below.
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class SimpleTesting {
public static void main(String args[]) {
// initialise a list
List<String> list =
Arrays.asList("Karan", "Rahul", "Jay", "Laxman", "Praful", "Kushagra", "Karan");
// intialise a set
Set<String> set = new HashSet<String>(list);
// display the set
for (String ele : set) {
System.out.println(ele);
}
}
}
Output:
Rahul
Kushagra
Jay
Karan
Laxman
Praful
In the code above, we create a list that stores some names. We also initialize an empty Set named set.
We pass the list as an argument to the constructor of HashMap. At last, we print the set’s elements. Notice how the duplicate elements in the list are eliminated.
Convert ArrayList to HashSet Using Java 8 Stream API
We used the Java 8 Stream API to convert the ArrayList to a HashSet in this approach. We first converted the list into a stream. We then apply the collect
function to the stream.
To convert into a set, we used the Collectors.toSet()
function in the collect
operation to get a set. Look at the code below.
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
public class SimpleTesting {
public static void main(String args[]) {
// initialise a list
List<String> list =
Arrays.asList("Karan", "Rahul", "Jay", "Laxman", "Praful", "Kushagra", "Karan");
// intialise a set using stream
Set<String> set = list.stream().collect(Collectors.toSet());
// display the set
for (String ele : set) {
System.out.println(ele);
}
}
}
Output:
Rahul
Kushagra
Jay
Karan
Laxman
Praful
We converted a list into a stream using the List.stream()
function in the code above. We apply the collect
operation to the stream and pass the Collectors.toSet()
method as an argument.
This results in the conversion of the stream into a set. We then store this set in the variable set.
Convert ArrayList to HashSet Using Set.copyOf()
Method in Java
Java 10 provides a Set.copyof()
method that takes a Collection as its argument and returns an immutable set containing the elements of the given Collection. In this approach, we use the Set.copyOf()
method to convert an ArrayList into a HashSet.
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
public class SimpleTesting {
public static void main(String args[]) {
// initialise a list
List<String> list =
Arrays.asList("Karan", "Rahul", "Jay", "Laxman", "Praful", "Kushagra", "Karan");
// intialise a set
Set<String> set = Set.copyOf(list);
// display the set
for (String ele : set) {
System.out.println(ele);
}
}
}
Output:
Rahul
Kushagra
Jay
Karan
Laxman
Praful
Convert ArrayList to HashSet Using Apache Commons Collection Library in Java
If you are working with the Apache Commons Collection library, use the CollectionUtils.addAll()
method that takes two arguments: target collection object and source collection object.
The values of the source collection object are copied into the target collection object and create a set. Look at the code below.
This code uses the dependency code of the Apache commons library. First, add this dependency to your Java project to execute this code successfully.
<!-- https://mvnrepository.com/artifact/commons-collections/commons-collections -->
<dependency>
<groupId>commons-collections</groupId>
<artifactId>commons-collections</artifactId>
<version>3.2.2</version>
</dependency>
import java.util.*;
public class SimpleTesting {
public static void main(String args[]) {
// initialise a list
List<String> sourcelist =
Arrays.asList("Karan", "Rahul", "Jay", "Laxman", "Praful", "Kushagra", "Karan");
// intialise a set
Set<String> targetset = new HashSet<String>();
// Adding elements
CollectionUtils.addAll(targetset, sourcelist);
// display the set
for (String ele : targetset) {
System.out.println(ele);
}
}
}
Output:
Rahul
Kushagra
Jay
Karan
Laxman
Praful
Related Article - Java ArrayList
- How to Sort Objects in ArrayList by Date in Java
- How to Find Unique Values in Java ArrayList
- Vector and ArrayList in Java
- Differences Between List and Arraylist in Java
- How to Compare ArrayLists in Java
- Merge Sort Using ArrayList in Java