How to Sort a Set in Java
- Use a List to Display a Sorted Set in Java
-
Use the
TreeSet
to Display a Sorted Set in Java -
Use the
stream()
Function to Display a Sorted Set in Java
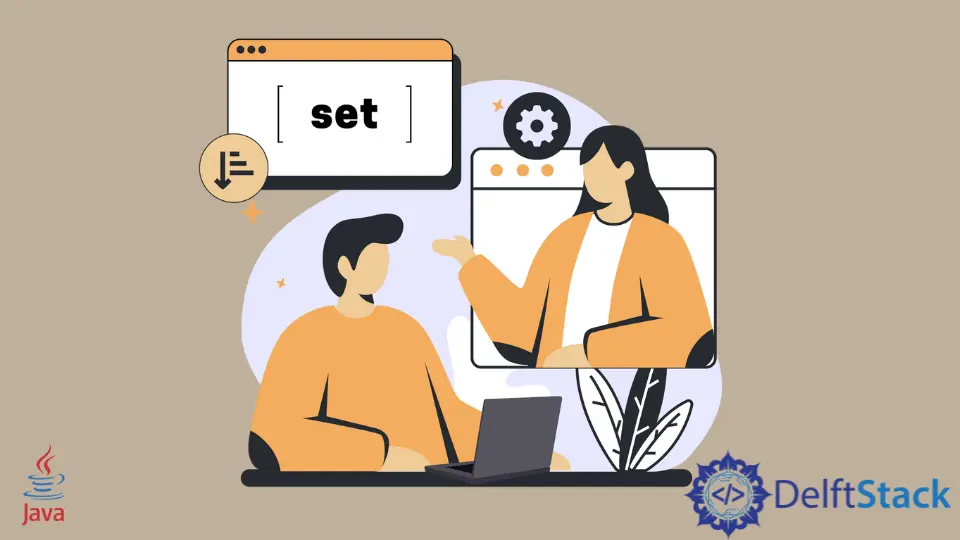
In Java, sets or HashSet
are commonly used to randomly access elements, as hash table elements are accessed using hash codes. Hash code is a unique identity that helps us to identify the elements of the hash table.
A HashSet
is an unordered collection. A set in Java does not have random access methods (like get(i)
where i is an index of that element), which are essential requirements by sorting algorithms. Putting simply, as HashSet
has its elements randomly placed, it does not offer any guarantee to order its elements due to undefined order.
Therefore, we need to think a little out of the box to sort a set in Java. We will discuss a few methods to convert it to a different structure and sort it.
Use a List to Display a Sorted Set in Java
A way to sort a HashSet
is by first converting it to a list and then sorting it.
We will add the elements from the set to the list and then use the sort()
function to sort it.
For example,
import java.util.*;
public class example {
public static void main(String[] args) {
HashSet<Integer> number = new HashSet<>();
// Using add() method
number.add(3);
number.add(6);
number.add(4);
// converting HashSet to arraylist
ArrayList<Integer> al = new ArrayList<>(number);
// sorting the list and then printing
Collections.sort(al);
System.out.println("Sorted list: ");
for (int x : al) {
System.out.print(x + " ");
}
}
}
Output:
Sorted list:
3 4 6
Use the TreeSet
to Display a Sorted Set in Java
TreeSet
class of the Collections
interface provides us with the functionality of tree data structure. We can convert the HashSet
to TreeSet
when we iterate through this collection. The elements are extracted in a well-defined order.
TreeSet
implements NavigableSet
interface, which extends SortedSet
, which further extends the Set
interface.
See the following example.
import java.util.*;
public class example {
public static void main(String[] args) {
HashSet<Integer> number = new HashSet<>();
// Using add() method
number.add(3);
number.add(6);
number.add(4);
// TreeSet gets the value of hashSet
TreeSet myTreeSet = new TreeSet();
myTreeSet.addAll(number);
System.out.println(myTreeSet);
}
}
Output:
[3, 4, 6]
Use the stream()
Function to Display a Sorted Set in Java
There is a concise way to this problem using the stream()
method. The stream API was introduced in Java 8 and is not a data structure in itself. However, it can take objects from different collections and display them in the desired way based on pipeline methods.
We will use the sorted()
method to display the final sequence in a sorted manner for our example.
See the code below.
import java.util.*;
public class example {
public static void main(String[] args) {
// creating hashset hs
HashSet<Integer> hs = new HashSet<>();
// Using add() method to add elements to hs
hs.add(20);
hs.add(4);
hs.add(15);
// before sorting hs
hs.forEach(System.out::println);
System.out.println(); // extra line
// after sorting hs
hs.stream().sorted().forEach(System.out::println); // yes
}
}
Output:
20
4
15
4
15
20