How to Find a Set Intersection in Java
Rashmi Patidar
Feb 02, 2024
Java
Java Set
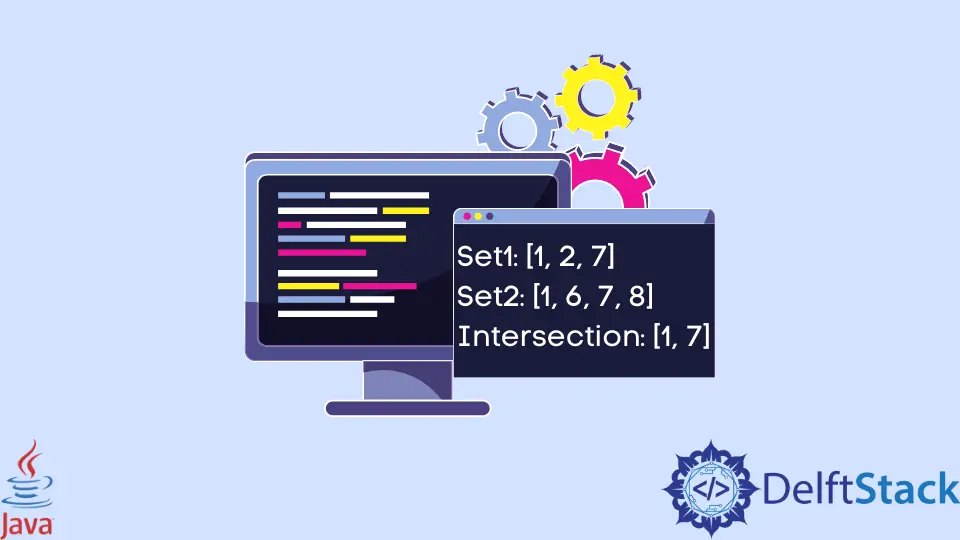
The term Set
is an interface present in the java.util
package. A set is a collection interface that stores unordered lists and does not allow the storage of duplicate entities. Mathematically, the set interface has three properties.
- The elements in the Set are non-null.
- No two elements in the Set can be equal.
- A Set does not preserve insertion order.
Use the Set Insertion and Find the Set Intersection in Java
You can see the program below, which demonstrates the Set insertion and finding the intersection between two sets in Java.
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class SetIntersection {
public static void main(String[] args) {
Set<Integer> s1 = new HashSet<>();
s1.add(2);
s1.add(7);
s1.add(1);
System.out.println("Set1: " + s1);
List list = Arrays.asList(1, 7, 6, 8);
Set<Integer> s2 = new HashSet<>(list);
System.out.println("Set2: " + s2);
Set<Integer> intersection = new HashSet<>(s1);
intersection.retainAll(s2);
System.out.println("Intersection: " + intersection);
}
}