How to Create a Concurrent Set in Java
-
Use the
ConcurrentHashMap
andKeySet()
Functions to Create a Concurrent Set in Java -
Use
ConcurrentHashMap
andnewKeySet()
Function to Create a Concurrent Set in Java
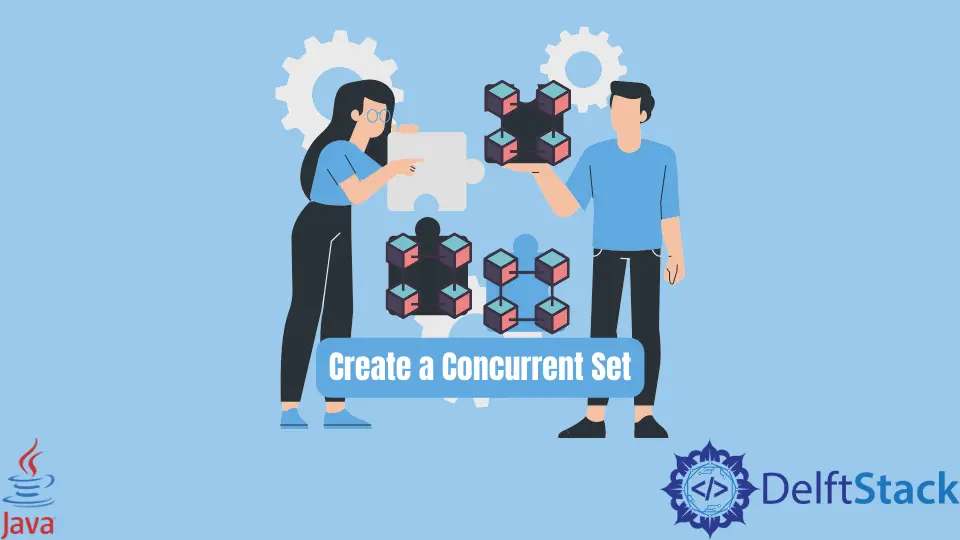
Before JDK 8, the Concurrent Hash Set was not supported in Java. And now, the ConcurrentHashMap
is used to create the Concurrent Set in Java.
The ConcurrentHashMap
has functions KeySet()
and newKeySet()
to create concurrent hash sets in Java.
This tutorial demonstrates how to create concurrent hash sets in Java.
Use the ConcurrentHashMap
and KeySet()
Functions to Create a Concurrent Set in Java
Using ConcurrentHashMap
and KeySet()
we can create a concurrent set in Java.
package delftstack;
import java.io.*;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
class ConcurrentSet {
public static void main(String[] args) {
// Creating a concurrent hash map
ConcurrentHashMap<String, Integer> demo_map = new ConcurrentHashMap<>();
demo_map.put("Delftstack", 10);
demo_map.put("Jack", 20);
demo_map.put("John", 30);
demo_map.put("Mike", 40);
demo_map.put("Michelle", 50);
// use keySet() to create a set from the concurrent hashmap
Set keyset_conc_set = demo_map.keySet();
System.out.println("The concurrent set using keySet() function is : " + keyset_conc_set);
}
}
The code creates a concurrent set of the names from the concurrent HashMap. We can not add more members to the set using the add()
method; it will throw an error.
Output:
The concurrent set using keySet() function is : [Michelle, Mike, Delftstack, John, Jack]
Use ConcurrentHashMap
and newKeySet()
Function to Create a Concurrent Set in Java
The newKeySet()
is used to create a concurrent set that can be manipulated later, adding or removing elements from the set.
package delftstack;
import java.io.*;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
class ConcurrentSet {
public static void main(String[] args) {
// Create a concurrent set using concorrentHashMap and newkeyset()
Set<String> newKeySet_conc_set = ConcurrentHashMap.newKeySet();
newKeySet_conc_set.add("Mike");
newKeySet_conc_set.add("Michelle");
newKeySet_conc_set.add("John");
newKeySet_conc_set.add("Jack");
// Print the concurrent set
System.out.println("The concurrent set before adding the element: " + newKeySet_conc_set);
// Add new element
newKeySet_conc_set.add("Delftstack");
// Show the change
System.out.println("The concurrent set after adding the element: " + newKeySet_conc_set);
// Check any element using contains
if (newKeySet_conc_set.contains("Delftstack")) {
System.out.println("Delftstack is a member of the set");
} else {
System.out.println("Delftstack is not a member of the set");
}
// Remove any element from the concurrent set
newKeySet_conc_set.remove("Delftstack");
System.out.println("The concurrent set after removing the element: " + newKeySet_conc_set);
}
}
The above code generates a concurrent set from ConcurrentHashMap
and the newKeySet
function and then add, remove, and check for the elements.
Output:
The concurrent set before adding the element: [Michelle, Mike, John, Jack]
The concurrent set after adding the element: [Michelle, Mike, Delftstack, John, Jack]
Delftstack is a member of the set
The concurrent set after removing the element: [Michelle, Mike, John, Jack]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook