Union and Intersection of Two Java Sets
- Define Union and Intersection in Java
- Understand Java Methods for Union and Intersection
- Union of Two String Sets Using Pure Java
- Union and Intersection of Two Sets Using Guava Library in Java
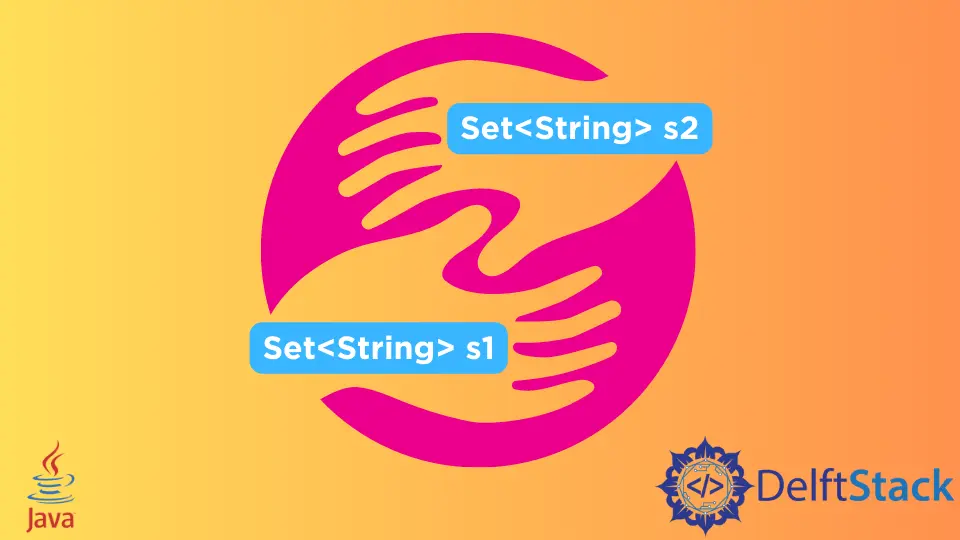
We will implement two programs in this demonstration. The first example will enable your solid understanding of the pure Java approach to determine union and intersection.
The second example will let you use Google’s Guava API to manipulate sets. Both algorithms successfully determined results while avoiding dirty code.
Define Union and Intersection in Java
- Union - The number of entities present in set A or set B is the union of A and B sets.
- Intersection - The intersection of two arrays, A and B, determines the elements belonging to both A and B groups.
- Sets - The most common way to determine the union/intersection of two Java sets.
Syntax of sets:
// First of all, define your sets as
// Demo set 1
Set<String> s1 = new HashSet<String>();
s1.add("a");
s1.add("b");
s1.add("c");
// set 2
Set<String> s2 = new HashSet<String>();
s2.add("d");
s2.add("e");
s2.add("a");
Union and Intersection (Easiest Method):
// union
Set<String> union = new HashSet<>(s1);
union.addAll(s2);
// intersection
Set<String> intersect = new HashSet<String>(s1);
intersect.retainAll(s2);
Understand Java Methods for Union and Intersection
In the previous example, we defined two array sets (s1)
and (s2)
, respectively. After that, we determined their union and intersection values by utilizing two methods.
For example, addAll()
for the union whereas retainAll()
of the intersection.
-
addAll()
Syntax:
Set<String> union = new HashSet<>(s1); union.addAll(s2); System.out.println("Result of union:" + union);
Operation:
Suppose the values in the given array aren’t already available. It adds them to a set.
Likewise, if the given collection is also a set, it changes the value of this set to be the union of the two sets.
Suppose the provided array is modified while the action is executing. The status of the action is undefined.
-
retainAll()
Syntax:
Set<String> intersect = new HashSet<String>(s1); intersect.retainAll(s2); System.out.println("Result of intersection:" + intersect);
Operation:
It keeps the given entities only in a set collection provided in a set. Precisely, it eliminates all of the items from this set that are not present in the given array.
While it also revises/updates this set so that its value is the intersection of the two groups if the given collection is also a set. Its return value is
true
if the set has been modified.
Union of Two String Sets Using Pure Java
The previous definitions were necessary prerequisites to form clarity. Now you must be familiar with the functions, syntax, and operation behavior of union and intersection within Java’s role.
Code:
import java.util.*;
public class UnionIntersectionJavaExample {
public static void main(String[] args) {
// set 1
Set<String> s1 = new HashSet<String>();
s1.add("a");
s1.add("b");
s1.add("c");
// set 2
Set<String> s2 = new HashSet<String>();
s2.add("d");
s2.add("e");
s2.add("a");
// union
Set<String> union = new HashSet<>(s1);
union.addAll(s2);
System.out.println("Result of union:" + union);
// intersection
Set<String> intersect = new HashSet<String>(s1);
intersect.retainAll(s2);
System.out.println("Result of intersection:" + intersect);
}
}
Output:
Result of union:[a, b, c, d, e]
Result of intersection:[a]
Union and Intersection of Two Sets Using Guava Library in Java
Although it depends on your requirements, it is not always necessary to use an API for a problem statement as straightforward as union and intersection.
That said, using this Guava library will also enable your understanding of a wide variety of easy methods. With Guava, specifying sets is more straightforward than before.
Set<String> mysets1 = Sets.newHashSet("This", "is", "set", "one");
Similarly, using methods is also easier.
// For union sets
// Sets.union (reserved function in Guava)
union = Sets.union(mysets1, mysets2);
// For interation
// Sets.intersect (reserved method in Guava)
intersection = Sets.intersection(mysets1, mysets2);
Code: (Guava)
import com.google.common.collect.Sets;
import java.util.Set;
public class UnionAndIntersectionGuava {
public static void main(String[] args) {
// Your first Set
Set<String> mysets1 = Sets.newHashSet("This", "is", "set", "one");
// Your Second Set
Set<String> mysets2 = Sets.newHashSet("Here", "is", "set", "two");
// We will use Guava's Sets.union() method
Set<String> union = Sets.union(mysets1, mysets2);
Set<String> intersection = Sets.intersection(mysets1, mysets2);
// Print the output
// System.out.println("Set 1:"+mysets1);
// System.out.println("Set 2:"+ mysets2);
System.out.println("Union:" + union);
// System.out.println("Set 1:"+mysets1);
// System.out.println("Set 2:"+ mysets2);
System.out.println("Intersection:" + intersection);
}
}
}
Output:
Union:[This, is, set, one, Here, two]
Intersection:[is, set]
You can download this API from here. After that, import it into your IDE (current project source folder) and set the path.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn