两个 Java 集合的并集和交集
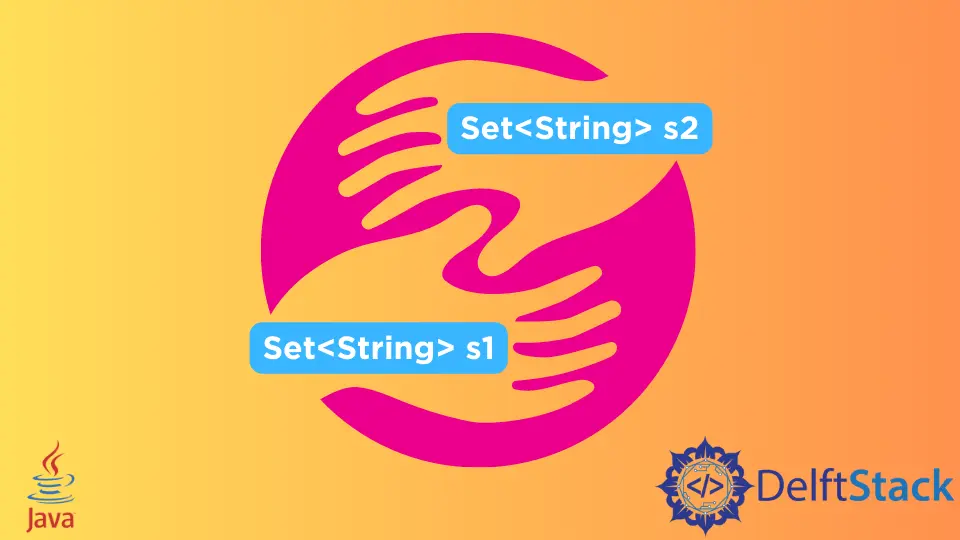
我们将在这个演示中实现两个程序。第一个示例将使你对确定并集和交集的纯 Java 方法有深入的了解。
第二个示例将让你使用 Google 的 Guava API 来操作集合。两种算法都成功地确定了结果,同时避免了脏代码。
在 Java 中定义并集和交集
- 并集 - 集合 A 或集合 B 中存在的实体数量是 A 和 B 集合的并集。
- 交集 - 两个数组 A 和 B 的交集确定属于 A 和 B 组的元素。
- 集合 - 确定两个 Java 集的并集/交集的最常用方法。
集合的语法:
// First of all, define your sets as
// Demo set 1
Set<String> s1 = new HashSet<String>();
s1.add("a");
s1.add("b");
s1.add("c");
// set 2
Set<String> s2 = new HashSet<String>();
s2.add("d");
s2.add("e");
s2.add("a");
并集和交集(最简单的方法):
// union
Set<String> union = new HashSet<>(s1);
union.addAll(s2);
// intersection
Set<String> intersect = new HashSet<String>(s1);
intersect.retainAll(s2);
了解用于并集和交集的 Java 方法
在前面的例子中,我们分别定义了两个数组集 (s1)
和 (s2)
。之后,我们利用两种方法确定了它们的并集和交集值。
例如,addAll()
表示并集,而 retainAll()
表示交集。
-
addAll()
语法:
Set<String> union = new HashSet<>(s1); union.addAll(s2); System.out.println("Result of union:" + union);
操作:
假设给定数组中的值尚不可用。它将它们添加到集合中。
同样,如果给定的集合也是一个集合,它会将这个集合的值更改为两个集合的并集。
假设在执行操作时修改了提供的数组。操作的状态未定义。
-
retainAll()
语法:
Set<String> intersect = new HashSet<String>(s1); intersect.retainAll(s2); System.out.println("Result of intersection:" + intersect);
操作:
它仅将给定实体保存在集合中提供的集合集合中。准确地说,它消除了该集合中不存在于给定数组中的所有项目。
虽然它还修改/更新这个集合,以便如果给定集合也是一个集合,它的值是两个组的交集。如果该集合已被修改,则其返回值为
true
。
使用纯 Java 实现两个字符串集的并集
先前的定义是形成清晰的必要先决条件。现在你必须熟悉 Java 角色中并集和交集的功能、语法和操作行为。
代码:
import java.util.*;
public class UnionIntersectionJavaExample {
public static void main(String[] args) {
// set 1
Set<String> s1 = new HashSet<String>();
s1.add("a");
s1.add("b");
s1.add("c");
// set 2
Set<String> s2 = new HashSet<String>();
s2.add("d");
s2.add("e");
s2.add("a");
// union
Set<String> union = new HashSet<>(s1);
union.addAll(s2);
System.out.println("Result of union:" + union);
// intersection
Set<String> intersect = new HashSet<String>(s1);
intersect.retainAll(s2);
System.out.println("Result of intersection:" + intersect);
}
}
输出:
Result of union:[a, b, c, d, e]
Result of intersection:[a]
Java 中使用 Guava 库的两个集合的并集和交集
尽管这取决于你的要求,但并不总是需要将 API 用于像并集和交集这样简单的问题陈述。
也就是说,使用这个 Guava 库还可以让你了解各种简单的方法。使用 Guava,指定集合比以前更简单。
Set<String> mysets1 = Sets.newHashSet("This", "is", "set", "one");
同样,使用方法也更容易。
// For union sets
// Sets.union (reserved function in Guava)
union = Sets.union(mysets1, mysets2);
// For interation
// Sets.intersect (reserved method in Guava)
intersection = Sets.intersection(mysets1, mysets2);
代码:(Guava)
import com.google.common.collect.Sets;
import java.util.Set;
public class UnionAndIntersectionGuava {
public static void main(String[] args) {
// Your first Set
Set<String> mysets1 = Sets.newHashSet("This", "is", "set", "one");
// Your Second Set
Set<String> mysets2 = Sets.newHashSet("Here", "is", "set", "two");
// We will use Guava's Sets.union() method
Set<String> union = Sets.union(mysets1, mysets2);
Set<String> intersection = Sets.intersection(mysets1, mysets2);
// Print the output
// System.out.println("Set 1:"+mysets1);
// System.out.println("Set 2:"+ mysets2);
System.out.println("Union:" + union);
// System.out.println("Set 1:"+mysets1);
// System.out.println("Set 2:"+ mysets2);
System.out.println("Intersection:" + intersection);
}
}
}
输出:
Union:[This, is, set, one, Here, two]
Intersection:[is, set]
你可以从此处下载此 API。之后,将其导入你的 IDE(当前项目源文件夹)并设置路径。
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn