How to Add Objects to an Array in Java
- Importance of Adding an Object to an Array
- Methods for Adding Objects to Arrays
- Best practices for Adding Objects
- Conclusion
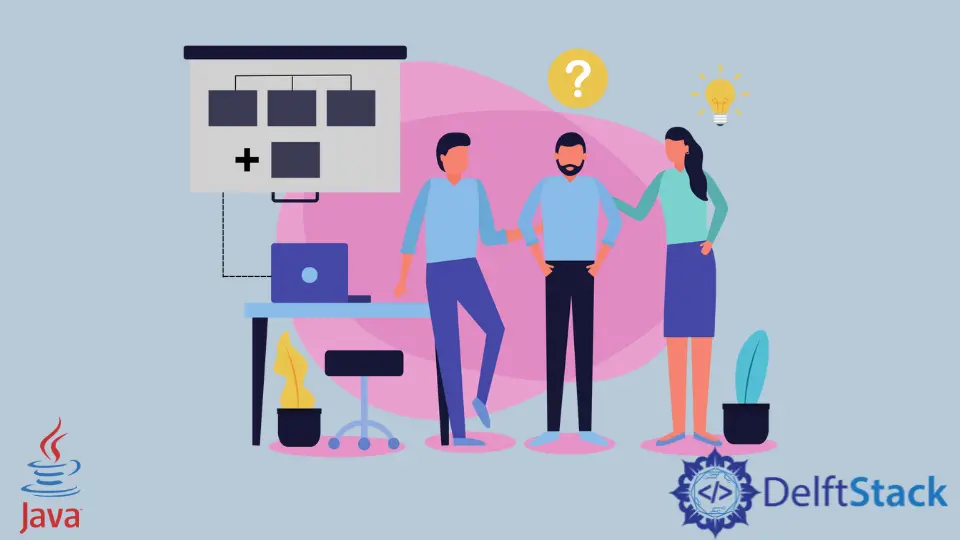
This article explores the crucial task of adding objects to arrays in Java, addressing its importance, diverse methods, and best practices for optimal code efficiency and scalability.
Importance of Adding an Object to an Array
Adding an object to an array is crucial for dynamic data management in Java. It allows for the flexible expansion of data structures, accommodating new elements without the need to predefine array sizes.
This adaptability is vital in scenarios where the number of elements is not known in advance or may change over time. By incorporating objects into arrays, Java programs gain the ability to dynamically scale and manage data, enhancing the efficiency and responsiveness of applications in handling evolving datasets.
Methods for Adding Objects to Arrays
Creating a New Array with a Larger Size
Creating a new array with a larger size when adding an object in Java is straightforward and effective. This method involves manually copying existing elements to a new array, accommodating the new element seamlessly.
While it may seem less concise than some alternatives, it offers transparency in resizing operations, providing developers with direct control over the process.
Let’s dive into a practical example where we have an array of students, and we need to add a new student to it. The objective is to showcase the technique of creating a new array with a larger size to facilitate the addition seamlessly.
public class StudentArrayExample {
public static void main(String[] args) {
// Existing array of students
Student[] students = {new Student("Alice"), new Student("Bob"), new Student("Charlie")};
// New student to be added
Student newStudent = new Student("David");
// Creating a new array with a larger size
Student[] newStudents = new Student[students.length + 1];
// Copying existing students to the new array
for (int i = 0; i < students.length; i++) {
newStudents[i] = students[i];
}
// Adding the new student to the end of the new array
newStudents[newStudents.length - 1] = newStudent;
// Updating the reference to the original array
students = newStudents;
// Displaying the updated array of students
for (Student student : students) {
System.out.println("Student: " + student.getName());
}
}
}
class Student {
private String name;
public Student(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
In this example, we start with an existing array of students and a new student object to be added. The key lies in creating a new array (newStudents
) with a size one unit larger than the existing array (students
).
We then iterate through the existing array, copying each student to the new array. After that, we add the new student to the end of the new array.
Finally, we update the reference to the original array with the new array.
Output:
When you run this code, you’ll observe that the new student, David
, seamlessly integrates into the array, and the updated array is displayed, demonstrating the successful addition of an object using the method of creating a new array with a larger size.
Using the Arrays.copyOf()
Method
Using Arrays.copyOf()
in Java for adding an object to an array is advantageous due to its simplicity and conciseness. It efficiently handles the creation of a new array with a specified size, streamlining the process of accommodating additional elements and enhancing code readability.
Consider a scenario where we have an array of students, and we want to add a new student to it. The Arrays.copyOf()
method will be employed to gracefully extend the array and include the new student.
import java.util.Arrays;
public class StudentArrayExample {
public static void main(String[] args) {
// Existing array of students
Student[] students = {new Student("Alice"), new Student("Bob"), new Student("Charlie")};
// New student to be added
Student newStudent = new Student("David");
// Using Arrays.copyOf() to create a new array with a larger size
students = Arrays.copyOf(students, students.length + 1);
// Adding the new student to the end of the array
students[students.length - 1] = newStudent;
// Displaying the updated array of students
for (Student student : students) {
System.out.println("Student: " + student.getName());
}
}
}
class Student {
private String name;
public Student(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
In this example, we start with an existing array of students and a new student object.
The pivotal moment occurs with the line students = Arrays.copyOf(students, students.length + 1);
. This single line gracefully creates a new array with a size one unit larger than the existing array (students
), copying all existing elements.
Next, we add the new student to the end of the array, leveraging the increased size. The reference to the original array is then updated to point to the new array, effectively incorporating the new student.
Output:
When executed, the code seamlessly adds the new student, David
, to the array, and the updated array is displayed. The beauty of using Arrays.copyOf()
lies in its simplicity, providing an efficient means to dynamically add objects to arrays in Java.
Using the ArrayList
Method
Unlike traditional arrays, ArrayList
is a dynamic array that can dynamically grow or shrink in size, making it a flexible and efficient choice for scenarios where the number of elements may change. Using ArrayList
in Java to add objects to an array is advantageous because it provides dynamic resizing capabilities, eliminating the need for manual size management and offering a more flexible and convenient approach for handling changing data sets.
Imagine a scenario where we have an array of students and need to seamlessly integrate a new student into it. The ArrayList
method offers an elegant solution for achieving this dynamically.
import java.util.ArrayList;
public class StudentArrayListExample {
public static void main(String[] args) {
// Existing ArrayList of students
ArrayList<Student> students = new ArrayList<>();
students.add(new Student("Alice"));
students.add(new Student("Bob"));
students.add(new Student("Charlie"));
// New student to be added
Student newStudent = new Student("David");
// Adding the new student to the ArrayList
students.add(newStudent);
// Displaying the updated ArrayList of students
for (Student student : students) {
System.out.println("Student: " + student.getName());
}
}
}
class Student {
private String name;
public Student(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
In this example, we begin with an ArrayList
of students and a new student object. The beauty of using ArrayList
is evident in its dynamic nature, allowing us to add elements without specifying the size beforehand.
Adding the new student is as straightforward as invoking the add()
method on the ArrayList
. This method automatically handles the resizing of the underlying array, sparing the developer from managing array sizes explicitly.
Output:
Upon execution, the code seamlessly incorporates the new student, David
, into the ArrayList
. The updated list is then displayed, showcasing the flexibility and ease of using ArrayList
for dynamic array manipulation.
This method proves invaluable in scenarios where the number of elements is subject to change, emphasizing the adaptability and efficiency of Java’s ArrayList
class.
Best practices for Adding Objects
Consider the Initial Array Size
Ensure the initial size of the array is chosen wisely based on an estimation of the expected number of elements. This helps minimize unnecessary resizing operations.
Use Dynamic Data Structures
Consider utilizing dynamic data structures like ArrayList
when frequent additions or removals are expected. These structures automatically handle resizing and offer flexibility.
Prefer ArrayList
for Dynamism
For scenarios where the size of the array is likely to change, consider using ArrayList
due to its inherent dynamic resizing capabilities.
Avoid Frequent Array Resizing
Minimize the frequency of resizing operations to enhance performance. If possible, estimate the maximum size needed initially to reduce resizing overhead.
Optimize Array Copying
When manually copying elements to a new array, leverage efficient methods such as Arrays.copyOf()
for readability and conciseness.
Encapsulate Logic in Methods
Abstract the array manipulation logic into methods, promoting code reusability and maintaining a clear and modular code structure.
Consider Performance Implications
Be mindful of the performance implications, especially in critical sections of your code. Evaluate whether the chosen method aligns with the specific requirements and constraints.
Use the Collections
Framework for Complex Structures
For complex data structures or scenarios involving collections of objects, consider using more advanced features provided by the Java Collections
Framework.
Document Array Resizing Strategies
If manual resizing is performed, document the resizing strategy employed, especially if it deviates from standard practices. This enhances code readability and maintainability.
Test with Varying Data Sets
Perform thorough testing with different data sets to ensure the chosen method scales appropriately and meets performance expectations across diverse scenarios.
Conclusion
Dynamically adding objects to arrays in Java is a fundamental skill, offering adaptability in managing evolving data sets. We explored various methods, from traditional array manipulation to leveraging dynamic structures like ArrayList
.
Each method has its merits, emphasizing the significance of choosing the right approach based on specific requirements. By understanding the best practices, such as optimizing array copying and considering initial array size, developers can ensure efficient and scalable solutions.
Mastering these techniques enhances code flexibility, maintainability, and overall proficiency in Java programming.