How to Truncate a String in C#
-
Use the
String.Substring()
Method to Truncate a String in C# -
Use the
PadRight
andSubstring
Method to Truncate a String in C# - Use LINQ to Truncate a String in C#
-
Use the
Remove()
Method to Truncate a String in C# - Conclusion
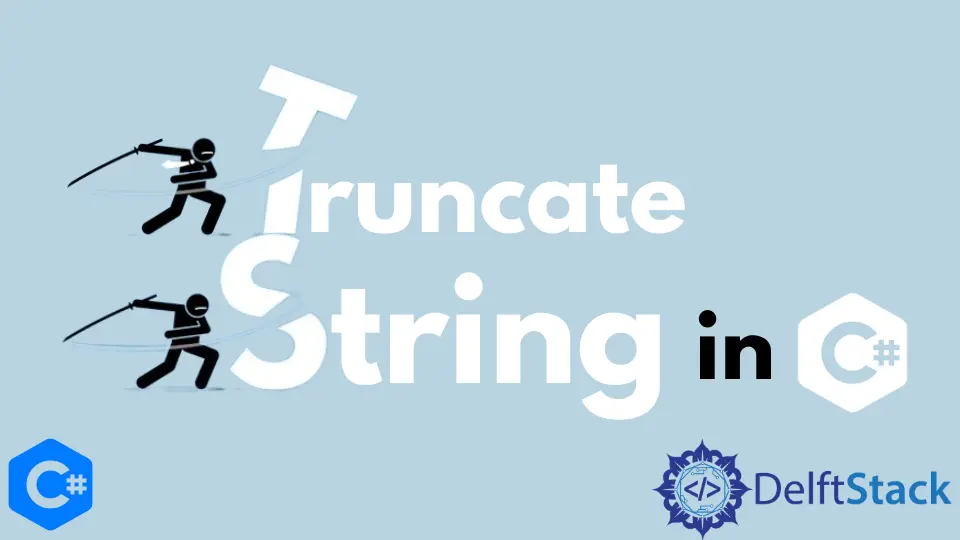
This tutorial will introduce the methods to truncate a string variable to a specified length in C#. While C# lacks a built-in method for string truncation, we can implement custom logic to achieve this.
Use the String.Substring()
Method to Truncate a String in C#
Unfortunately, there is no built-in method to truncate a string in C#. We have to write code with our logic for this purpose.
The String.Substring(x, y)
method retrieves a substring from the string that starts from the x
index and has a length of y
. We can use the String.Substring()
method and the LINQ to create an extension method that works the string variable.
Code Example:
using System;
namespace truncate_string {
public static class StringExt {
public static string Truncate(this string variable, int Length) {
if (string.IsNullOrEmpty(variable))
return variable;
return variable.Length <= Length ? variable : variable.Substring(0, Length);
}
}
class Program {
static void Main(string[] args) {
string variable = "This is a long, very long string and we want to truncate it.";
variable = variable.Truncate(22);
Console.WriteLine(variable);
}
}
}
Output:
This is a long, very l
In the code, we have a program that utilizes a custom extension method, Truncate
, to shorten a given string to a specified length. The extension method is housed in the StringExt
class, offering a reusable way to truncate strings, and it checks if the input string is either null or empty and returns it unchanged if so.
If the string’s length is less than or equal to the specified length, the original string is returned unaltered. However, if the string is longer than the desired length, the method uses the Substring
function to extract a substring starting from index 0
and having a length equal to the specified truncation length.
In the Main
method, we initialize a string variable, variable
, with a lengthy string. We then apply the Truncate
method to limit it to 22
characters and print the result to the console using Console.WriteLine
.
The output will be This is a long, very l
representing the truncated string.
Use the PadRight
and Substring
Method to Truncate a String in C#
Using the PadRight
and Substring
methods to truncate a string involves padding the original string to the desired length and then taking a substring of the specified length.
Code Example:
using System;
namespace truncate_string {
public static class StringExt {
public static string Truncate(this string variable, int length) {
if (string.IsNullOrEmpty(variable))
return variable;
return variable.PadRight(length).Substring(0, length);
}
}
class Program {
static void Main(string[] args) {
string variable = "This is a long, very long string and we want to truncate it.";
variable = variable.Truncate(22);
Console.WriteLine(variable);
}
}
}
Output:
This is a long, very l
In this code, we have a program designed to truncate a string to a specified length using a custom extension method named Truncate
. The Truncate
method is a part of the StringExt
class and is applied to a string variable.
First, it checks if the input string is null or empty, and if so, it returns the string unchanged. Otherwise, it uses the combination of PadRight
and Substring
methods to truncate the string.
The PadRight
function is employed to add padding to the right of the original string until it reaches the specified length. Subsequently, the Substring
method extracts a substring starting from index 0
with a length equal to the specified truncation length.
In the Main
method, we initialize a string variable, variable
, with a long string. We then use the Truncate
method to limit it to 22
characters and print the result to the console using Console.WriteLine
.
The output will be This is a long, very l
representing the truncated string.
Use LINQ to Truncate a String in C#
Using LINQ to truncate a string in C# involves using the Take()
method to select a specified number of characters from the beginning of the string.
Code Example:
using System;
using System.Linq;
namespace truncate_string {
public static class StringExt {
public static string Truncate(this string variable, int length) {
if (string.IsNullOrEmpty(variable))
return variable;
return new string(variable.Take(length).ToArray());
}
}
class Program {
static void Main(string[] args) {
string variable = "This is a long, very long string and we want to truncate it.";
variable = variable.Truncate(22);
Console.WriteLine(variable);
}
}
}
Output:
This is a long, very l
In this code, we have a program designed to truncate a string to a specified length using a custom extension method named Truncate
. The Truncate
method, residing in the StringExt
class, utilizes LINQ to achieve the truncation.
First, it checks if the input string is null or empty, and if so, it returns the string unchanged. Otherwise, it employs LINQ’s Take()
method to select the first length
characters from the original string.
The result is then converted back to a string using ToArray()
and new string(...)
. In the Main
method, we initialize a string variable, variable
, with a long string.
We then use the Truncate
method to limit it to 22
characters and print the result to the console using Console.WriteLine
. The output will be This is a long, very l
representing the truncated string.
Use the Remove()
Method to Truncate a String in C#
Using the Remove
method to truncate a string involves removing characters from a specified position to the end of the string.
Code Example:
using System;
namespace truncate_string {
public static class StringExt {
public static string Truncate(this string variable, int length) {
if (string.IsNullOrEmpty(variable))
return variable;
return variable.Length <= length ? variable : variable.Remove(length);
}
}
class Program {
static void Main(string[] args) {
string variable = "This is a long, very long string and we want to truncate it.";
variable = variable.Truncate(22);
Console.WriteLine(variable);
}
}
}
Output:
This is a long, very l
In this code, we’ve crafted a program to truncate a string to a specified length using a custom extension method called Truncate
. The Truncate
method, situated within the StringExt
class, checks if the input string is either null or empty, and if it is, we return the string unchanged.
Otherwise, we evaluate if the length of the string is less than or equal to the specified truncation length. If true, we return the original string, and if false, we use the Remove()
method to eliminate characters from the specified length to the end of the string.
In the Main
method, we initialize a string variable, variable
, with a long string. We then apply the Truncate
method to limit it to 22
characters and print the result to the console using Console.WriteLine
.
The output will be This is a long, very l
representing the truncated string.
Conclusion
In conclusion, we have several options when it comes to truncating strings in C#. Whether through substring manipulation, padding, or LINQ, each method provides a unique approach to tailor string lengths based on specific requirements.
Understanding these techniques empowers developers to choose the most suitable method for their applications, ensuring efficient and effective string truncation in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn