在 C# 中截斷字串
Muhammad Maisam Abbas
2023年12月11日
Csharp
Csharp String
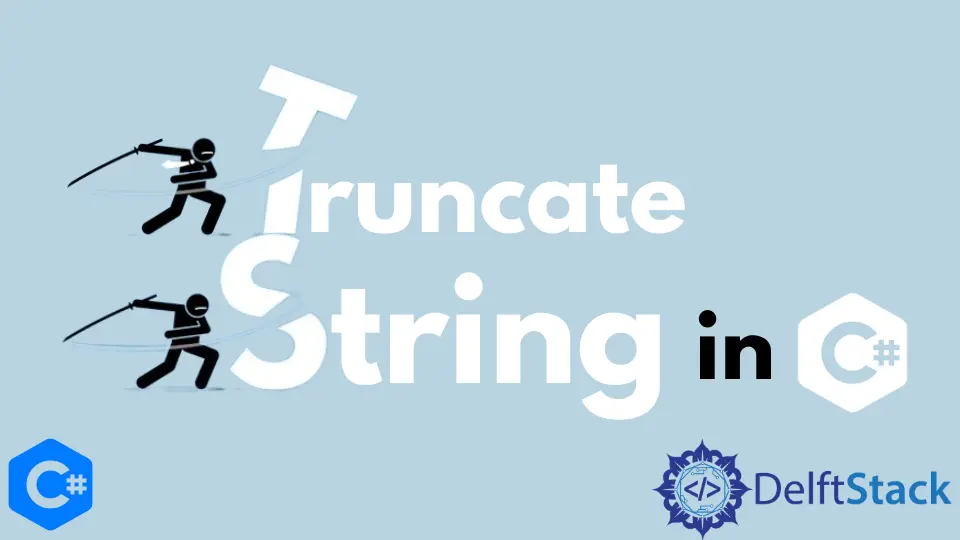
本教程將介紹在 C# 中將字串變數截斷為指定長度的方法。
在 C# 中使用 String.Substring()
方法截斷字串
不幸的是,沒有內建的方法來截斷 C# 中的字串。為此,我們必須使用自己的邏輯編寫程式碼。String.Substring(x, y)
方法從字串中檢索一個子字串,該子字串從 x
索引開始,長度為 y
。我們可以使用 String.Substring()
方法和 LINQ 來建立一個擴充套件方法,該擴充套件方法可以處理字串變數。以下程式碼示例向我們展示瞭如何使用 C# 中的 String.Substring()
方法截斷字串變數。
using System;
namespace truncate_string {
public static class StringExt {
public static string Truncate(this string variable, int Length) {
if (string.IsNullOrEmpty(variable))
return variable;
return variable.Length <= Length ? variable : variable.Substring(0, Length);
}
}
class Program {
static void Main(string[] args) {
string variable = "This is a long, very long string and we want to truncate it.";
variable = variable.Truncate(22);
Console.WriteLine(variable);
}
}
}
輸出:
This is a long, very l
在上面的程式碼中,我們使用 C# 中的 String.Substring()
方法將字串變數 variable
截短為 22
個字元。然後,我們建立了擴充套件方法 Truncate()
,該方法採用所需的長度並將字串截斷為所需的長度。如果字串變數為 null
或為空,則 Truncate()
方法將返回字串。如果所需的長度大於字串的長度,則它將返回原始字串。如果較小,則 Truncate()
方法使用 String.Substring()
方法將字串截斷為所需的長度,然後返回該字串的新截斷副本。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn