How to Initialize a Dictionary in C#
-
Initialize a Dictionary of Pre-Defined data types in
C#
-
Initialize a Dictionary of User-Defined data types in
C#
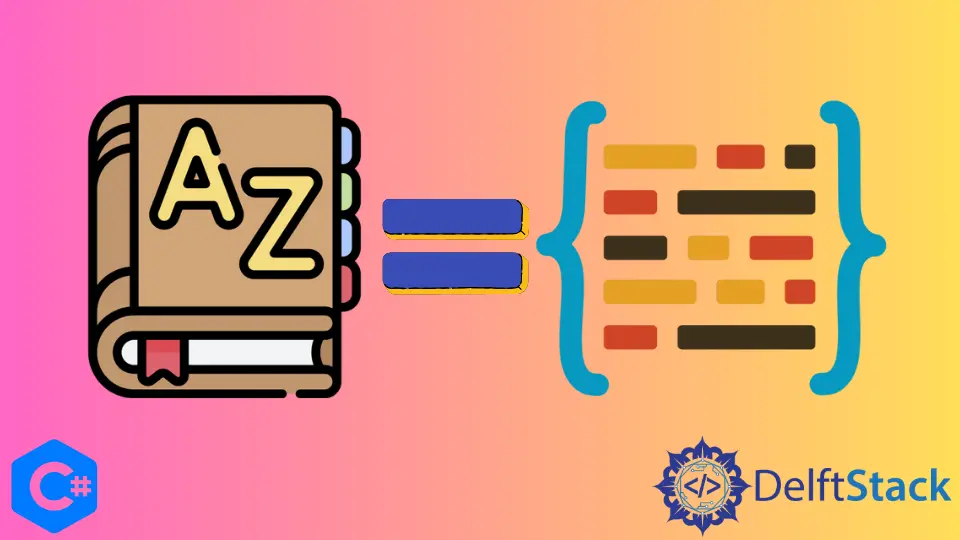
In this tutorial, we will discuss methods to initialize a dictionary in C#.
Initialize a Dictionary of Pre-Defined data types in C#
A Dictionary data structure holds data in the form of key/value
pairs. The Dictionary<key, value>
class can be used to create a dictionary in C#. We can use the constructor of the Dictionary<key, value>
class to initialize a dictionary in C#. The following code example shows us how we can initialize a dictionary with the constructor of the Dictionary<key, value>
class in C#.
using System;
using System.Collections.Generic;
namespace initialize_dictionary {
class Program {
static void Main(string[] args) {
Dictionary<string, string> myDictionary = new Dictionary<string, string> {
{ "Key1", "Value1" },
{ "Key2", "Value2" },
{ "Key3", "Value3" },
};
foreach (var x in myDictionary) {
Console.WriteLine(x);
}
}
}
}
Output:
[Key1, Value1]
[Key2, Value2]
[Key3, Value3]
We declared and initialized the dictionary myDictionary
with the constructor of the Dictionary<key, value>
class in C#. We can declare and initialize dictionaries of any pre-defined data type with this example but cannot declare and initialize a dictionary of user-defined data types with this method in C#.
Initialize a Dictionary of User-Defined data types in C#
We can initialize a dictionary of class objects with the new
operator in C#. The new
operator is used to allocate memory location to the class definition. The following code example shows us how we can initialize a dictionary of class objects with the new
operator in C#.
using System;
using System.Collections.Generic;
namespace initialize_dictionary {
public class Person {
private string name;
private string email;
public Person(string n, string e) {
name = n;
email = e;
}
class Program {
static void Main(string[] args) {
Dictionary<int, Person> myDictionary =
new Dictionary<int, Person> { { 1, new Person("Person 1", "email1") },
{ 2, new Person("Person 2", "email2") },
{ 3, new Person("Person 3", "email3") } };
}
}
}
We declared the class Person
with the attributes name
and email
of the string data type. We defined a constructor to initialize the name
and email
class members. We invoked the constructor of the Person
class inside the constructor of the Dictionary<key, value>
class with the new
operator in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn