C#에서 사전 초기화
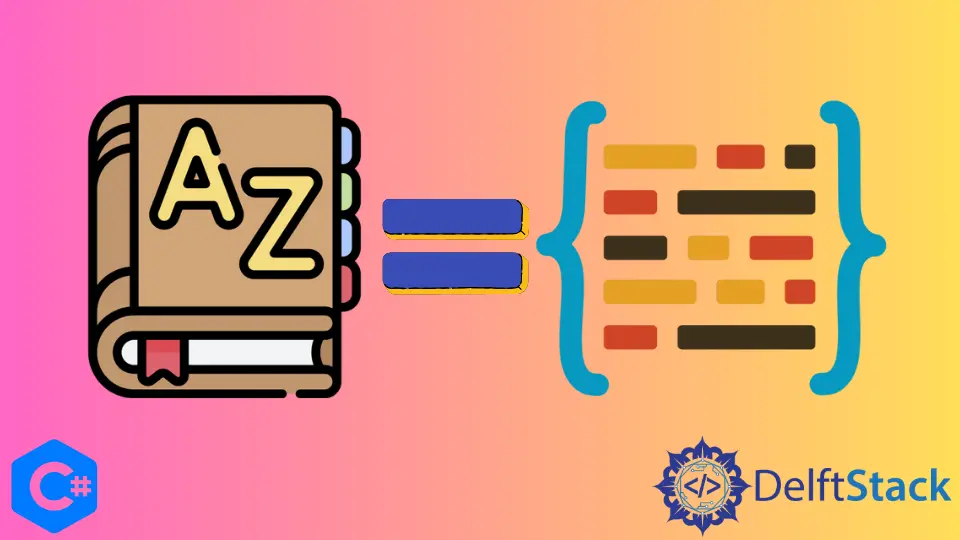
이 자습서에서는 C#에서 사전을 초기화하는 방법에 대해 설명합니다.
C#에서 사전 정의 된 데이터 형식의 사전 초기화
사전 데이터 구조는키/값
쌍의 형식으로 데이터를 보유합니다. Dictionary<key, value>
클래스를 사용하여 C#에서 사전을 만들 수 있습니다. Dictionary<key, value>
클래스의 생성자를 사용하여 C#에서 사전을 초기화 할 수 있습니다. 다음 코드 예제는 C#에서Dictionary<key, value>
클래스의 생성자를 사용하여 사전을 초기화하는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
namespace initialize_dictionary {
class Program {
static void Main(string[] args) {
Dictionary<string, string> myDictionary = new Dictionary<string, string> {
{ "Key1", "Value1" },
{ "Key2", "Value2" },
{ "Key3", "Value3" },
};
foreach (var x in myDictionary) {
Console.WriteLine(x);
}
}
}
}
출력:
[Key1, Value1]
[Key2, Value2]
[Key3, Value3]
C#에서Dictionary<key, value>
클래스의 생성자로myDictionary
사전을 선언하고 초기화했습니다. 이 예제에서는 미리 정의 된 데이터 형식의 사전을 선언하고 초기화 할 수 있지만 C#에서는이 메서드를 사용하여 사용자 정의 데이터 형식의 사전을 선언하고 초기화 할 수 없습니다.
C#에서 사용자 정의 데이터 형식 사전 초기화
C#에서new
연산자를 사용하여 클래스 객체 사전을 초기화 할 수 있습니다. new
연산자는 클래스 정의에 메모리 위치를 할당하는 데 사용됩니다. 다음 코드 예제는 C#에서new
연산자를 사용하여 클래스 객체 사전을 초기화하는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
namespace initialize_dictionary {
public class Person {
private string name;
private string email;
public Person(string n, string e) {
name = n;
email = e;
}
class Program {
static void Main(string[] args) {
Dictionary<int, Person> myDictionary =
new Dictionary<int, Person> { { 1, new Person("Person 1", "email1") },
{ 2, new Person("Person 2", "email2") },
{ 3, new Person("Person 3", "email3") } };
}
}
}
문자열 데이터 유형의name
및email
속성을 사용하여Person
클래스를 선언했습니다. name
및email
클래스 멤버를 초기화하는 생성자를 정의했습니다. C#에서new
연산자를 사용하여Dictionary<key, value>
클래스의 생성자 내에서Person
클래스의 생성자를 호출했습니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn