How to Initialize a Byte Array in C#
-
Use a
for
Loop to Initialize a Byte Array in C# -
Use the
Enumerable.Repeat
andToArray
Methods to Initialize a Byte Array in C# -
Use the
Buffer.BlockCopy
Method to Initialize a Byte Array in C# -
Use the
Array.Fill
Method to Initialize a Byte Array in C# - Conclusion
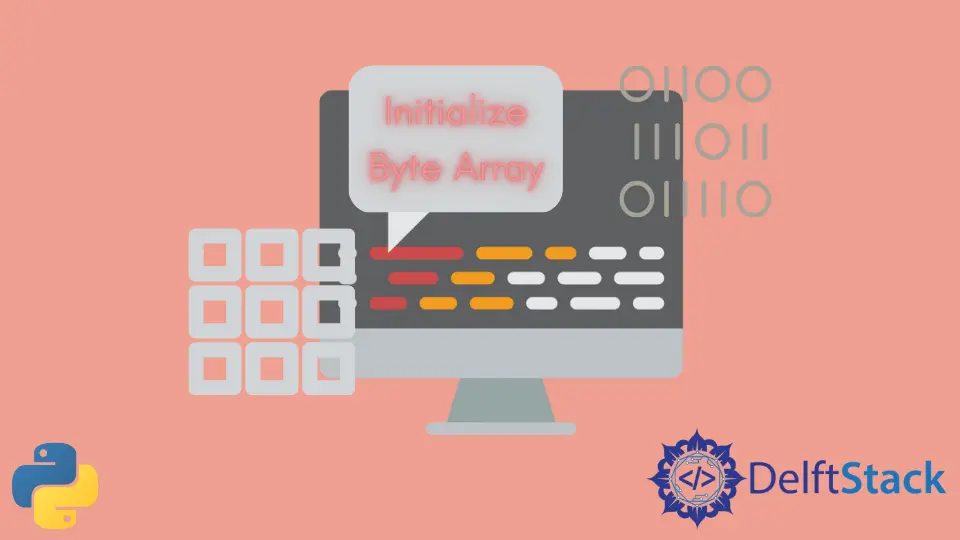
In C#, a byte array is a fundamental data structure that allows you to store and manipulate binary data. Initializing a byte array means setting its initial values, which is a crucial step before using it for various operations, such as network communication, cryptography, or data manipulation.
This article will explore different methods for initializing a byte array in C#. We’ll explore techniques ranging from simple loops to more advanced approaches like LINQ and specialized methods.
Use a for
Loop to Initialize a Byte Array in C#
Binary data can be stored in byte arrays. This information might be in a data file, an image file, a compressed file, or a downloaded server response.
We’ll demonstrate how to start a byte array of a specified length. Let’s start with the implementation.
Firstly, we import the System
library. This library will allow us to use its features and methods in our C# program.
using System;
We then create a ByteArray
class consisting of the Main()
method.
class ByteArray {
static void Main() {}
}
Within our Main()
method, let’s initialize a variable called byteItems
with a byte[]
array. The array’s length can be specified in one of two ways.
First, we place the value immediately within the square []
brackets. It will inform the array that your length has been set.
var byteItems = new byte[7];
The alternate method is to assign the values following the square []
brackets within the curly {}
braces, as seen below. We’ll use this in our example.
var byteItems = new byte[] { 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10 };
Now that we’ve determined the array’s length let’s assign a value to each index. A for
loop cycles through the length of the byteItems
array, assigning the provided value at each index.
In addition, we’ll utilize each index of the array to print the value contained within it.
for (int x = 0; x < byteItems.Length; x++) {
byteItems[x] = 9;
Console.WriteLine(byteItems[x]);
}
Finally, we’ll display the total length of the array in the console.
Console.WriteLine("The length of the array: {0}", byteItems.Length);
Full Source Code:
using System;
class ByteArray {
static void Main() {
var byteItems = new byte[] { 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10 };
for (int x = 0; x < byteItems.Length; x++) {
byteItems[x] = 9;
Console.WriteLine(byteItems[x]);
}
Console.WriteLine("The length of the array: {0}", byteItems.Length);
}
}
Output:
9
9
9
9
9
9
9
The length of the array: 7
Use the Enumerable.Repeat
and ToArray
Methods to Initialize a Byte Array in C#
The Enumerable.Repeat
method is part of the LINQ (Language Integrated Query) library in C#. It allows us to generate a sequence containing a specified element repeated a specified number of times.
Here’s the syntax:
Enumerable.Repeat<T>(T element, int count);
T
: The type of element you want to repeat.element
: The element to be repeated.count
: The number of times you want to repeat it.
Initializing a Byte Array
To initialize a byte array using Enumerable.Repeat
and ToArray
, follow these steps:
-
Decide on the value you want to repeat in the byte array. This could be any valid byte value (
0-255
). -
Determine the length of the byte array you want to create. This is essentially the number of times you want the value to be repeated.
-
Use
Enumerable.Repeat
:byte value = 42; // Example value (0-255) int length = 5; // Example length IEnumerable<byte> repeatedBytes = Enumerable.Repeat(value, length);
This creates an
IEnumerable<byte>
containingvalue
repeatedlength
times. -
Convert to an Array:
byte[] byteArray = repeatedBytes.ToArray();
The
ToArray
method converts theIEnumerable<byte>
to a byte array.
Here’s the full code that demonstrates initializing a byte array with Enumerable.Repeat
:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main() {
byte value = 42; // Example value (0-255)
int length = 5; // Example length
IEnumerable<byte> repeatedBytes = Enumerable.Repeat(value, length);
byte[] byteArray = repeatedBytes.ToArray();
Console.WriteLine("Initialized Byte Array:");
foreach (byte b in byteArray) {
Console.Write(b + " ");
}
}
}
In this example, a byte array of length 5
is created, with each element having the value 42
. The program then proceeds to print the initialized byte array. The loop iterates over each element in the array and prints it to the console.
If you were to run this program, the output would be:
Initialized Byte Array:
42 42 42 42 42
This demonstrates how to effectively initialize a byte array with a repeated value in C# using the Enumerable.Repeat
and ToArray
methods.
Use the Buffer.BlockCopy
Method to Initialize a Byte Array in C#
The Buffer.BlockCopy
method is part of the System
namespace in C#. It allows you to perform low-level memory operations on arrays.
Here’s the syntax:
public static void BlockCopy(Array src, int srcOffset, Array dst, int dstOffset, int count);
Here’s what each parameter represents:
src
: The source array from which data will be copied.srcOffset
: The starting index in the source array from which to begin copying.dst
: The destination array to which data will be copied.dstOffset
: The starting index in the destination array where data will be copied.count
: The number of elements to copy.
Initializing a Byte Array With Buffer.BlockCopy
To initialize a byte array using Buffer.BlockCopy
, you’ll need to follow these steps:
-
Create a Source Array: You need a source array that contains the data you want to copy into the byte array.
-
Specify Offsets: Determine the starting points (offsets) in both the source and destination arrays.
-
Invoke
Buffer.BlockCopy
: Call theBuffer.BlockCopy
method with the appropriate parameters. -
Verify the Result: Optionally, check the content of the byte array to ensure it has been initialized correctly.
Example 1: Initializing a Byte Array With Incrementing Values
Let’s illustrate the process mentioned above with an example. We’ll initialize a byte array with incrementing values.
int[] sourceArray = { 1, 2, 3, 4, 5 };
byte[] byteArray = new byte[sourceArray.Length * sizeof(int)];
Buffer.BlockCopy(sourceArray, 0, byteArray, 0, byteArray.Length);
Console.WriteLine("Byte Array:");
foreach (var element in byteArray) {
Console.Write(element + " ");
}
In this example, we have an integer array sourceArray
containing [1, 2, 3, 4, 5]
. We create a byte array byteArray
with a length equal to the length of sourceArray
times the size of an integer (since each integer occupies 4
bytes).
We then use Buffer.BlockCopy
to copy the data from sourceArray
to byteArray
. The entire contents of sourceArray
are copied into byteArray
.
After this operation, byteArray
will contain the binary representation of the integers in sourceArray
.
Output:
Byte Array:
1 0 0 0 2 0 0 0 3 0 0 0 4 0 0 0 5 0 0 0
Example 2: Initializing a Byte Array With a Pattern
You can also use Buffer.BlockCopy
to initialize a byte array with a specific pattern. For instance, if you want to fill the array with zeros, you can do the following:
byte[] byteArray = new byte[10];
// Filling the byteArray with zeros
Buffer.BlockCopy(new int[10], 0, byteArray, 0, byteArray.Length);
Console.WriteLine("Byte Array:");
foreach (var element in byteArray) {
Console.Write(element + " ");
}
In this example, we create a byte array byteArray
of length 10
. We then use Buffer.BlockCopy
to copy data from an integer array of the same length filled with zeros.
This effectively initializes byteArray
with zeros.
Output:
Byte Array:
0 0 0 0 0 0 0 0 0 0
Use the Array.Fill
Method to Initialize a Byte Array in C#
The Array.Fill
method is part of the System
namespace and is available in C# 7.2 and later versions. It allows you to assign a specific value to all elements within an array.
Here’s the syntax:
public static void Fill<T>(T[] array, T value);
Here, T
represents the type of the array elements, array
is the target array to be filled, and value
is the value that will be assigned to all elements.
Initializing a Byte Array With Array.Fill
-
Initialize a Byte Array
To begin, create a byte array that you want to initialize. For example:
byte[] byteArray = new byte[10];
Here, a byte array named
byteArray
is created with a length of10
. This means it can hold ten elements, each of which will be a byte (a value ranging from0
to255
). -
Set a Value to Assign
byte valueToSet = 255;
A variable named
valueToSet
is declared as a byte, and it is assigned the value255
. In binary, this is represented as11111111
. -
Use
Array.Fill
to Assign ValuesArray.Fill(byteArray, valueToSet);
The
Array.Fill
method is called to set all elements ofbyteArray
to the value specified invalueToSet
. In this case, all elements inbyteArray
will now be255
. -
Verify Initialization
You can verify the initialization by printing out the elements of the byte array:
foreach (var element in byteArray) { Console.WriteLine(element); }
This will output
255
ten times, indicating that the byte array has been successfully initialized.
Full Source Code:
using System;
class Program {
static void Main() {
byte[] byteArray = new byte[10];
byte valueToSet = 255;
Array.Fill(byteArray, valueToSet);
foreach (var element in byteArray) {
Console.WriteLine(element);
}
}
}
Output:
255
255
255
255
255
255
255
255
255
255
Conclusion
In this article, we’ve explored various methods to initialize a byte array to a certain value in C#. Each method has its advantages, so choose the one that best fits your specific scenario.
Whether you prefer a straightforward loop, a LINQ-based approach, or utilizing specialized methods like Buffer.BlockCopy
or Array.Fill
, you now have a solid understanding of how to perform this operation effectively in C#.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn