在 C# 中初始化一個位元組陣列
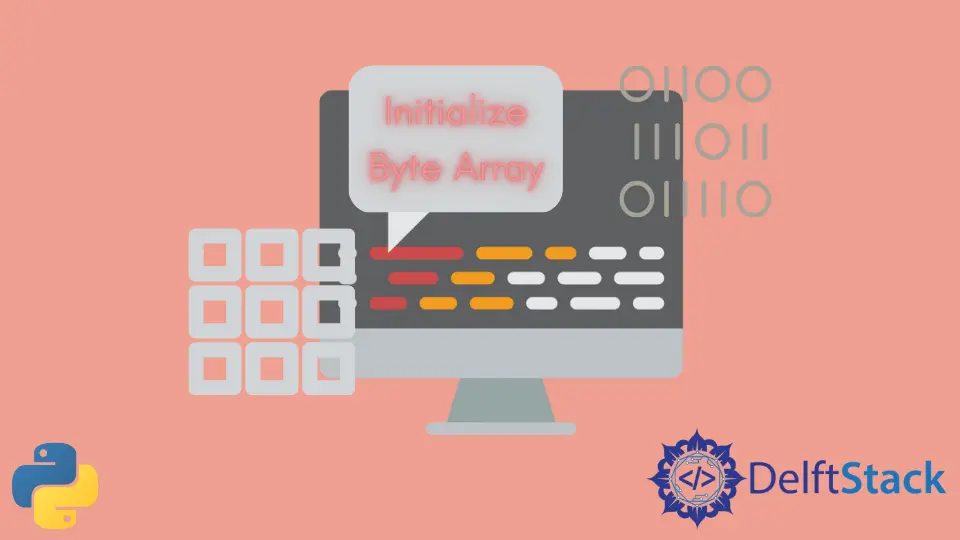
這篇文章解釋瞭如何在 C# 中把一個位元組陣列初始化為一個特定的值。
在 C#
中位元組陣列的用法
二進位制資料可以儲存在位元組陣列中。這些資訊可能在一個資料檔案、一個影象檔案、一個壓縮檔案或下載的伺服器響應中。
我們將演示如何啟動一個指定長度的位元組陣列。讓我們從實現開始。
首先,我們匯入 System
庫。這個庫將允許我們在 C# 程式中使用其功能和方法。
using System;
然後我們建立一個 ByteArray
類,由 Main()
方法組成。
class ByteArray {
static void Main() {}
}
在我們的 Main()
方法中,讓我們用一個 byte[]
陣列初始化一個叫做 byteItems
的變數。陣列的長度可以通過以下兩種方式之一來指定。
首先,我們把這個值緊緊地放在方括號 []
內。它將通知陣列,你的長度已經被設定。
var byteItems = new byte[7];
另一種方法是在方 []
括號後面的大括號 {}
內賦值,如下圖所示。我們將在我們的例子中使用這種方法。
var byteItems = new byte[] { 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10 };
現在我們已經確定了陣列的長度,讓我們給每個索引分配一個值。一個 for
迴圈在 byteItems
陣列的長度上迴圈,在每個索引上分配所提供的值。
此外,我們將利用陣列的每個索引來列印其中包含的值。
for (int x = 0; x < byteItems.Length; x++) {
byteItems[x] = 9;
Console.WriteLine(byteItems[x]);
}
最後,我們將輸出陣列的總長度。
Console.WriteLine("The length of the array: {0}", byteItems.Length);
完整的原始碼。
using System;
class ByteArray {
static void Main() {
var byteItems = new byte[] { 0x10, 0x10, 0x10, 0x10, 0x10, 0x10, 0x10 };
for (int x = 0; x < byteItems.Length; x++) {
byteItems[x] = 9;
Console.WriteLine(byteItems[x]);
}
Console.WriteLine("The length of the array: {0}", byteItems.Length);
}
}
輸出:
9
9
9
9
9
9
9
The length of the array: 7
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn