How to Read a Text File Line by Line in C#
-
Read a Text File Line by Line by Using
File.ReadLines()
Method inC#
-
Read a Text File Line by Line by Using
File.ReadAllLines()
Method inC#
-
Read a Text File Line by Line by Using
StreamReader.ReadLine()
Method inC#
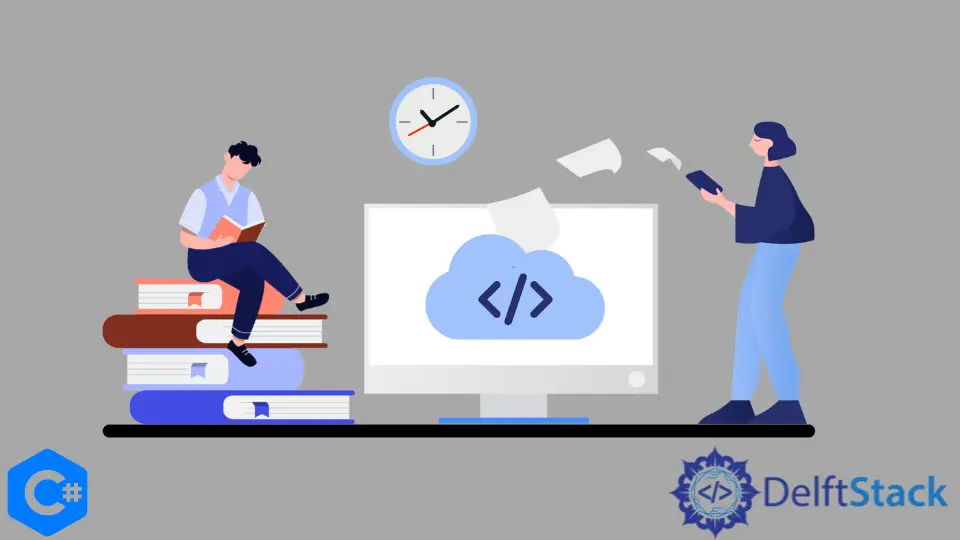
We can perform several operations on a text file. To use this data in any program, we first need to read that data in a proper data structure.
In C#, there are several ways to read a text file line by line efficiently. Continue reading below to find these ways.
Read a Text File Line by Line by Using File.ReadLines()
Method in C#
File.ReadLines()
method is the best method found to read a text file line by line efficiently. This method returns an Enumerable
for large text files, that’s why we have created an Enumerable string
object to store the text file.
The correct syntax to use this method is as follows:
File.ReadLines(FileName);
Example Code:
using System;
using System.Collections.Generic;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
// Creating enumerable object
IEnumerable<string> line = File.ReadLines(FileToRead);
Console.WriteLine(String.Join(Environment.NewLine, line));
}
}
Output:
// All the text, the file contains will display here.
File.ReadLines()
method throws an IOException
if there is an issue opening the file and FileNotFoundException
if the requested file does not exist.
Read a Text File Line by Line by Using File.ReadAllLines()
Method in C#
File.ReadAllLines()
method can also be used to read a file line by line. It does not return an Enumerable
but returns a string array that contains all the lines of the text file.
The correct syntax to use this method is as follows:
File.ReadAllLines(FileName);
Example Code:
using System;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
// Creating string array
string[] lines = File.ReadAllLines(FileToRead);
Console.WriteLine(String.Join(Environment.NewLine, lines));
}
}
This method also throws exceptions just like the File.ReadLines()
method. These exceptions are then handled using a try-catch
block.
Read a Text File Line by Line by Using StreamReader.ReadLine()
Method in C#
The StreamReader
class in C# provides a method StreamReader.ReadLine()
. This method reads a text file to the end line by line.
The correct syntax to use this method is as follows:
// We have to create Streader Object to use this method
StreamReader ObjectName = new StreamReader(FileName);
ObjectName.ReadLine();
Example Code:
using System;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
using (StreamReader ReaderObject = new StreamReader(FileToRead)) {
string Line;
// ReaderObject reads a single line, stores it in Line string variable and then displays it on
// console
while ((Line = ReaderObject.ReadLine()) != null) {
Console.WriteLine(Line);
}
}
}
}
Output:
// All the text, the file contains will display here.