How to Download a File From a URL in C#
Minahil Noor
Feb 16, 2024
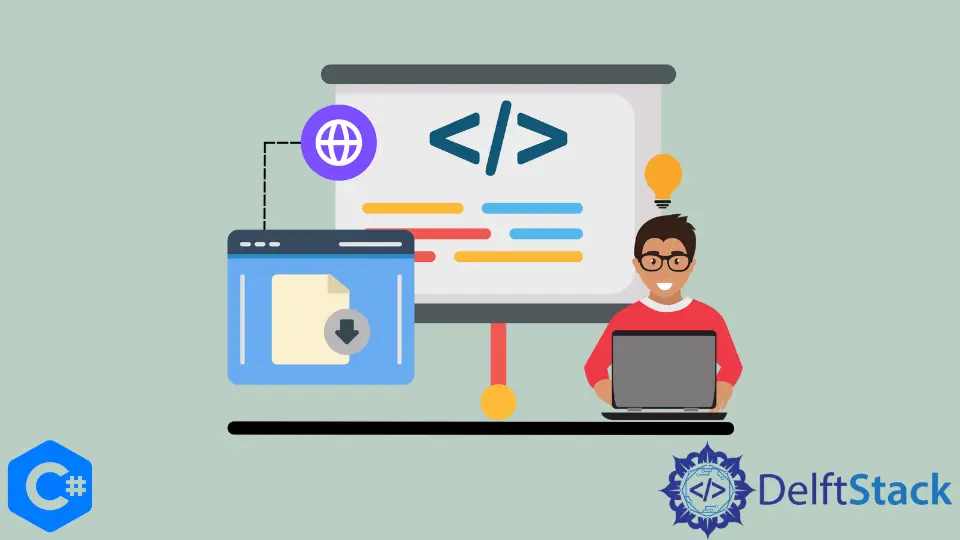
In this article, we will introduce a method to download a file from a URL
.
- Using the
DownloadFile()
method
Use the DownloadFile()
Method to Download a File From a URL in C#
We will use the system-defined method DownloadFile()
to download a file from a URL
. We will have to create a new WebClient
object to call this method. The correct syntax to use this function is as follows.
WebClient.DownloadFile(Uri address, string fileName);
The built-in method DownloadFile()
has two parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
address |
mandatory | It is the URL from where we want to download the file. |
fileName |
mandatory | It is the name of the file that we want to download. |
The program below shows how we can use the DownloadFile()
method to download a file from a URL.
using System;
using System.Net;
class DownloadFile {
static void Main() {
WebClient mywebClient = new WebClient();
mywebClient.DownloadFile("http://mysite.com/myfile.txt", @"d:\myfile.txt");
}
}
The file has been downloaded to the local disk D.