How to Rename a File in C#
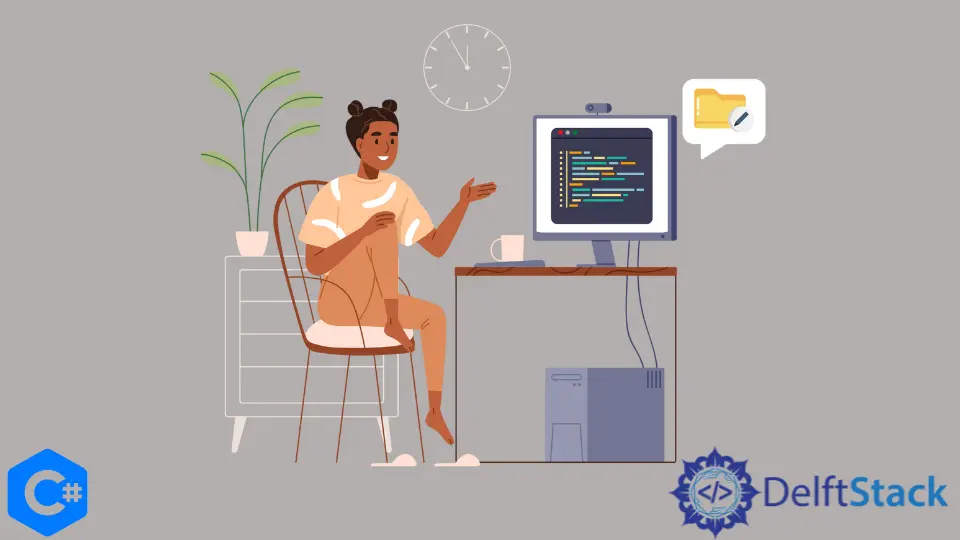
In this article, we will introduce different methods to rename a file using C# code.
- Using
Move()
method - Using
Copy()
method
Use the Move()
Method to Rename a File in C#
We will use the system-defined method Move()
to rename a file. We will move the old file to a new file with a new name. The correct syntax to use this method is as follows.
File.Move(string oldFile, string newFile);
The built-in method Move()
has two parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
oldFile |
mandatory | It is the file we want to rename. It is a string variable. |
newFile |
mandatory | It is the new name and path of the new file. |
The program below shows how we can use the Move()
method to rename a file.
using System;
using System.IO;
class RenameFile {
static void Main() {
string oldName = "D:\myfolder\myfile.txt";
string newName = "D:\myfolder\mynewfile.txt";
System.IO.File.Move(oldName, newName);
}
}
The file must exist in the specified directory. If it does not exist, then the function will throw the FileNotFoundException
error.
Use Copy()
Method to Rename a File in C#
We will use the Copy()
method to rename a file. This method copies the file to a new file and changes its directory to the specified directory. The correct syntax to use this method is as follows.
File.Copy(string oldFile, string newFile);
The built-in method Copy()
has two parameters. The details of its parameters are as follows.
Parameters | Description | |
---|---|---|
oldFile |
mandatory | It is the file that we want to copy to a new file. It is a string variable. |
newFile |
mandatory | It is the new file. It is a string variable. |
The program below shows how we can use the Copy()
method to rename a file.
using System;
using System.IO;
public class RenameFile {
public static void Main() {
string oldFile = @"D:\oldfile.txt";
string newFile = @"D:\newfile.txt";
File.Copy(oldFile, newFile);
}
}