How to Read Embedded Resource Text File in C#
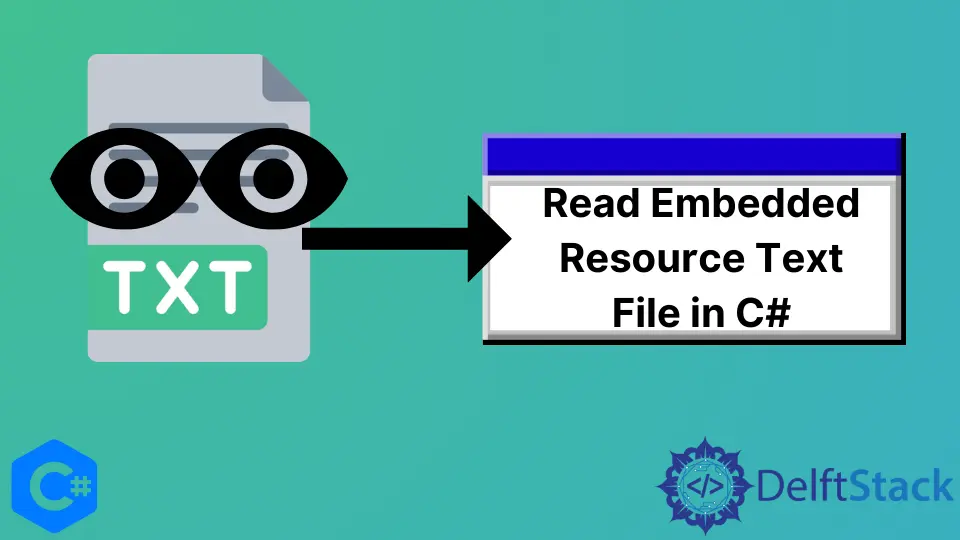
This tutorial demonstrates reading embedded resource text files using the C# programming language.
Read Embedded Resource Text File
The embedded files are referred to as Embedded Resources, and you can access these files at runtime by using the Assembly class located in the System.Reflection
namespace. An embedded file may be created from any file currently part of the Project.
To read an embedded resource text file, we’ve to follow the below steps:
-
Add Folder and File
First, we’ll add a folder named
files
to the Project. Then we’ll add the file embedded within that folder by utilizing theAdd -> Existing Item
option that can be found in theContext Menu
of theSolution Explorer
, as shown below.It will allow us to add the file embedded within the folder.
After the files that are going to be embedded have been added, you will need to right-click the
File
, then click on theProperties
.Now, follow the screenshot below to change the value of the
Build Action
attribute fromContent
to the embedded resource. -
Create Windows Form
First, add a
TextBox
to windows form and name ittxtTextBox
from itsProperties
.Now, add a button named
btnText
and showRead Embed Text
text on the button as shown below: -
Write Windows Form Code
First, we’ve to import the following libraries:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.IO; using System.Reflection;
By right-clicking on the
Read Embed Text
button to create its event, here, we’ll write button code as follows:We’ll create an
Assembly
method object namedassembly
:var asmbly = Assembly.GetExecutingAssembly();
Then, we’ll create a
var
type variable namedfilepath
. This variable will hold the text file path.var filePath = "ReadEmbedTextbyZeeshan.files.Shani.txt";
Lastly, We’ll show embedded file text using
StreamReader
, which will read a text file from the given path.using (Stream s = asmbly.GetManifestResourceStream(filePath)) using ( StreamReader sr = new StreamReader(s)) { txtTextBox.Text = sr.ReadToEnd(); }
-
Complete Source Code
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.IO; using System.Reflection; namespace ReadEmbedTextbyZeeshan { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void btnText_Click(object sender, EventArgs e) { var asmbly = Assembly.GetExecutingAssembly(); var filePath = "ReadEmbedTextbyZeeshan.files.Shani.txt"; using (Stream s = asmbly.GetManifestResourceStream(filePath)) using ( StreamReader sr = new StreamReader(s)) { txtTextBox.Text = sr.ReadToEnd(); } } } }
When we click on the
Read Embed Text
button, it will show the below output:Output:
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn