C# 텍스트 파일을 한 줄씩 읽습니다
-
C#에서
File.ReadLines()
메서드를 사용하여 한 줄씩 텍스트 파일을 읽습니다 -
C#에서
File.ReadAllLines()
메서드를 사용하여 한 줄씩 텍스트 파일을 읽습니다 -
C#에서
StreamReader.ReadLine()
메서드를 사용하여 한 줄씩 텍스트 파일을 읽습니다
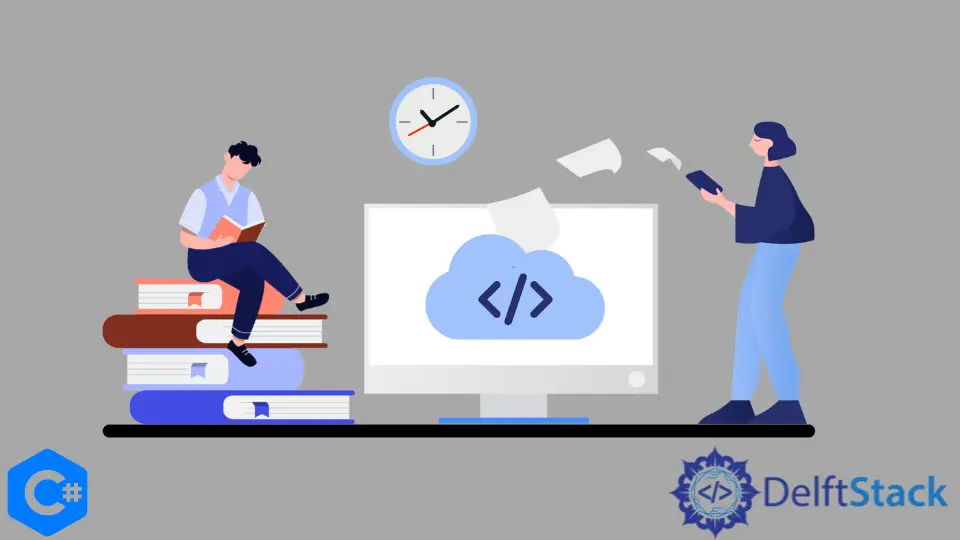
텍스트 파일에 대해 여러 가지 작업을 수행 할 수 있습니다. 모든 프로그램에서이 데이터를 사용하려면 먼저 해당 데이터를 적절한 데이터 구조로 읽어야합니다.
C#에서는 텍스트 파일을 한 줄씩 효율적으로 읽는 여러 가지 방법이 있습니다. 이러한 방법을 찾으려면 아래를 계속 읽으십시오.
C#에서File.ReadLines()
메서드를 사용하여 한 줄씩 텍스트 파일을 읽습니다
File.ReadLines()
메소드는 텍스트 파일을 한 줄씩 효율적으로 읽는 가장 좋은 방법입니다. 이 메소드는 큰 텍스트 파일에 대해 Enumerable
을 리턴하므로 텍스트 파일을 저장하기 위해 Enumerable string
오브젝트를 작성했습니다.
이 방법을 사용하는 올바른 구문은 다음과 같습니다.
File.ReadLines(FileName);
예제 코드:
using System;
using System.Collections.Generic;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
// Creating enumerable object
IEnumerable<string> line = File.ReadLines(FileToRead);
Console.WriteLine(String.Join(Environment.NewLine, line));
}
}
출력:
// All the text, the file contains will display here.
File.ReadLines()
메소드는 파일을 여는 데 문제가 있으면IOException
을, 요청 된 파일이 없으면FileNotFoundException
을 발생시킵니다.
C#에서File.ReadAllLines()
메서드를 사용하여 한 줄씩 텍스트 파일을 읽습니다
File.ReadAllLines()
메소드를 사용하여 파일을 한 줄씩 읽을 수도 있습니다. Enumerable
은 반환하지 않지만 텍스트 파일의 모든 줄을 포함하는 문자열 배열을 반환합니다.
이 방법을 사용하는 올바른 구문은 다음과 같습니다.
File.ReadAllLines(FileName);
예제 코드:
using System;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
// Creating string array
string[] lines = File.ReadAllLines(FileToRead);
Console.WriteLine(String.Join(Environment.NewLine, lines));
}
}
출력:
// All the text, the file contains will display here.
이 메소드는 File.ReadLines()
메소드와 마찬가지로 예외를 발생시킵니다. 이러한 예외는try-catch
블록을 사용하여 처리됩니다.
C#에서StreamReader.ReadLine()
메서드를 사용하여 한 줄씩 텍스트 파일을 읽습니다
C#의StreamReader
클래스는StreamReader.ReadLine()
메소드를 제공합니다. 이 메소드는 텍스트 파일을 줄 단위로 읽습니다.
이 방법을 사용하는 올바른 구문은 다음과 같습니다.
// We have to create Streader Object to use this method
StreamReader ObjectName = new StreamReader(FileName);
ObjectName.ReadLine();
예제 코드:
using System;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
using (StreamReader ReaderObject = new StreamReader(FileToRead)) {
string Line;
// ReaderObject reads a single line, stores it in Line string variable and then displays it on
// console
while ((Line = ReaderObject.ReadLine()) != null) {
Console.WriteLine(Line);
}
}
}
}
출력:
// All the text, the file contains will display here.