C# 逐行读取文本文件
Minahil Noor
2023年10月12日
-
使用 C# 中的
File.ReadLines()
方法逐行读取文本文件 -
使用 C# 中的
File.ReadAllLines()
方法逐行读取文本文件 -
使用 C# 中的
StreamReader.ReadLine()
方法逐行读取文本文件
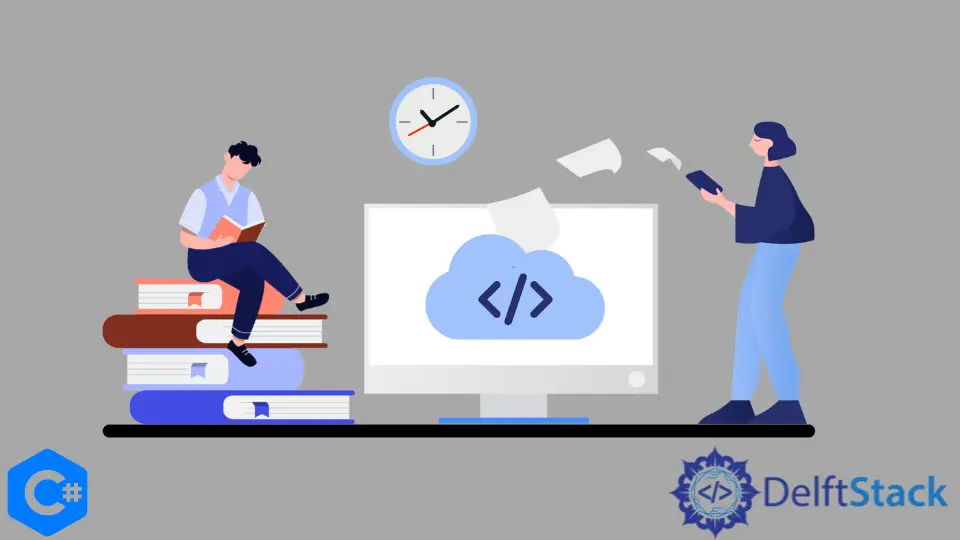
我们可以对文本文件执行多种操作。要在任何程序中使用文件中的数据,我们首先需要以适当的数据结构读取该数据。
在 C# 中,有几种有效地逐行读取文本文件的方法。
使用 C# 中的 File.ReadLines()
方法逐行读取文本文件
File.ReadLines()
方法是高效地逐行读取文本文件的最佳方法。这个方法为大型文本文件返回一个枚举类型 Enumerable
,这就是为什么我们创建了一个 Enumerable string
对象来存储文本文件的原因。
使用此方法的正确语法如下:
File.ReadLines(FileName);
示例代码:
using System;
using System.Collections.Generic;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
// Creating enumerable object
IEnumerable<string> line = File.ReadLines(FileToRead);
Console.WriteLine(String.Join(Environment.NewLine, line));
}
}
输出:
// All the text, the file contains will display here.
如果打开文件时出现问题,File.ReadLines()
方法将抛出 IOException
;如果请求的文件不存在,则抛出 FileNotFoundException
。
使用 C# 中的 File.ReadAllLines()
方法逐行读取文本文件
File.ReadAllLines()
方法也可用于逐行读取文件。它不返回 Enumerable
。它返回一个字符串数组,其中包含文本文件的所有行。
使用此方法的正确语法如下:
File.ReadAllLines(FileName);
示例代码:
using System;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
// Creating string array
string[] lines = File.ReadAllLines(FileToRead);
Console.WriteLine(String.Join(Environment.NewLine, lines));
}
}
输出:
// All the text, the file contains will display here.
这个方法也抛出异常,就像 File.ReadLines()
方法一样。然后使用 try-catch
块来处理这些异常。
使用 C# 中的 StreamReader.ReadLine()
方法逐行读取文本文件
C# 中的 StreamReader
类提供了 StreamReader.ReadLine()
方法。此方法逐行将文本文件读取到末尾。
StreamReader.ReadLine()
方法的正确语法如下:
// We have to create Streader Object to use this method
StreamReader ObjectName = new StreamReader(FileName);
ObjectName.ReadLine();
示例代码:
using System;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
using (StreamReader ReaderObject = new StreamReader(FileToRead)) {
string Line;
// ReaderObject reads a single line, stores it in Line string variable and then displays it on
// console
while ((Line = ReaderObject.ReadLine()) != null) {
Console.WriteLine(Line);
}
}
}
}
输出:
// All the text, the file contains will display here.