C# テキストファイルを 1 行ずつ読み取る
-
C# で
File.ReadLines()
メソッドを使用してテキストファイルを 1 行ずつ読み取る -
C# で
File.ReadAllLines()
メソッドを使用してテキストファイルを 1 行ずつ読み取る -
C# で
StreamReader.ReadLine()
メソッドを使用して行ごとにテキストファイルを読み取る
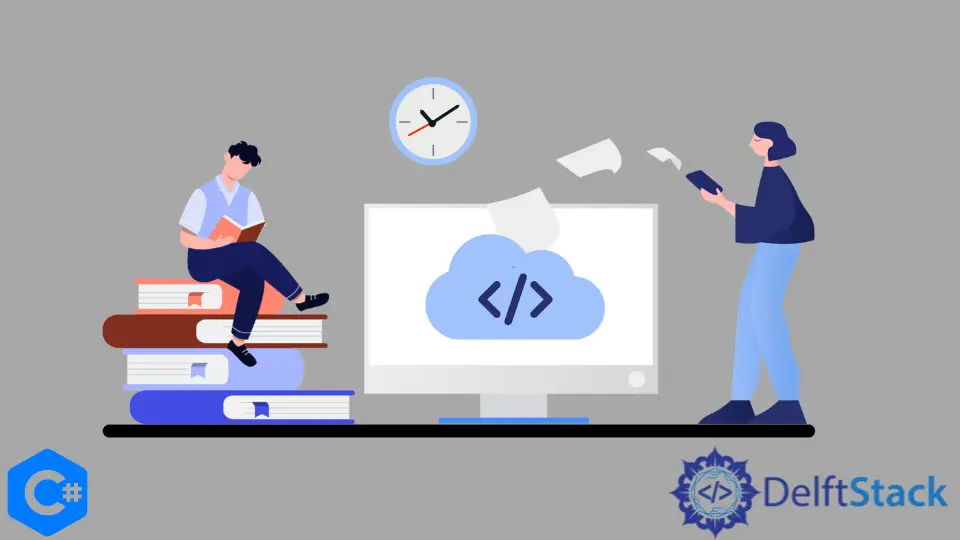
テキストファイルに対していくつかの操作を実行できます。このデータをプログラムで使用するには、まず適切なデータ構造でそのデータを読み取る必要があります。
C# では、テキストファイルを 1 行ずつ効率的に読み取る方法がいくつかあります。以下を読んで、これらの方法を見つけてください。
C# で File.ReadLines()
メソッドを使用してテキストファイルを 1 行ずつ読み取る
File.ReadLines()
メソッドは、テキストファイルを 1 行ずつ効率的に読み取るのに最適なメソッドです。このメソッドは、大きなテキストファイルに対して Enumerable
を返します。そのため、テキストファイルを格納するために Enumerable string
オブジェクトを作成しました。
このメソッドを使用するための正しい構文は次のとおりです。
File.ReadLines(FileName);
コード例:
using System;
using System.Collections.Generic;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
// Creating enumerable object
IEnumerable<string> line = File.ReadLines(FileToRead);
Console.WriteLine(String.Join(Environment.NewLine, line));
}
}
出力:
// All the text, the file contains will display here.
File.ReadLines()
メソッドは、ファイルを開くときに問題がある場合は IOException
をスローし、リクエストされたファイルが存在しない場合は FileNotFoundException
をスローします。
C# で File.ReadAllLines()
メソッドを使用してテキストファイルを 1 行ずつ読み取る
File.ReadAllLines()
メソッドを使用して、ファイルを 1 行ずつ読み取ることもできます。Enumerable
は返しません。テキストファイルのすべての行を含む文字列配列を返します。
このメソッドを使用するための正しい構文は次のとおりです。
File.ReadAllLines(FileName);
コード例:
using System;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
// Creating string array
string[] lines = File.ReadAllLines(FileToRead);
Console.WriteLine(String.Join(Environment.NewLine, lines));
}
}
出力:
// All the text, the file contains will display here.
このメソッドも File.ReadLines()
メソッドと同じように例外をスローします。これらの例外は、try-catch
ブロックを使用して処理されます。
C# で StreamReader.ReadLine()
メソッドを使用して行ごとにテキストファイルを読み取る
C# の StreamReader
クラスはメソッド StreamReader.ReadLine()
を提供します。このメソッドは、テキストファイルを行ごとに最後まで読み取ります。
このメソッドを使用するための正しい構文は次のとおりです。
// We have to create Streader Object to use this method
StreamReader ObjectName = new StreamReader(FileName);
ObjectName.ReadLine();
コード例:
using System;
using System.IO;
public class ReadFile {
public static void Main() {
string FileToRead = @"D:\New folder\textfile.txt";
using (StreamReader ReaderObject = new StreamReader(FileToRead)) {
string Line;
// ReaderObject reads a single line, stores it in Line string variable and then displays it on
// console
while ((Line = ReaderObject.ReadLine()) != null) {
Console.WriteLine(Line);
}
}
}
}
出力:
// All the text, the file contains will display here.