How to Identify if a String Is a Number in C#
-
C# Program to Identify if a
string
Is a Number UsingEnumerable.All()
Method -
C# Program to Identify if a
string
Is a Number UsingRegex.IsMatch()
Method -
C# Program to Identify if a
string
Is a Number UsingInt32.TryParse()
Method -
C# Program to Identify if a
string
Is a Number Usingforeach
Loop
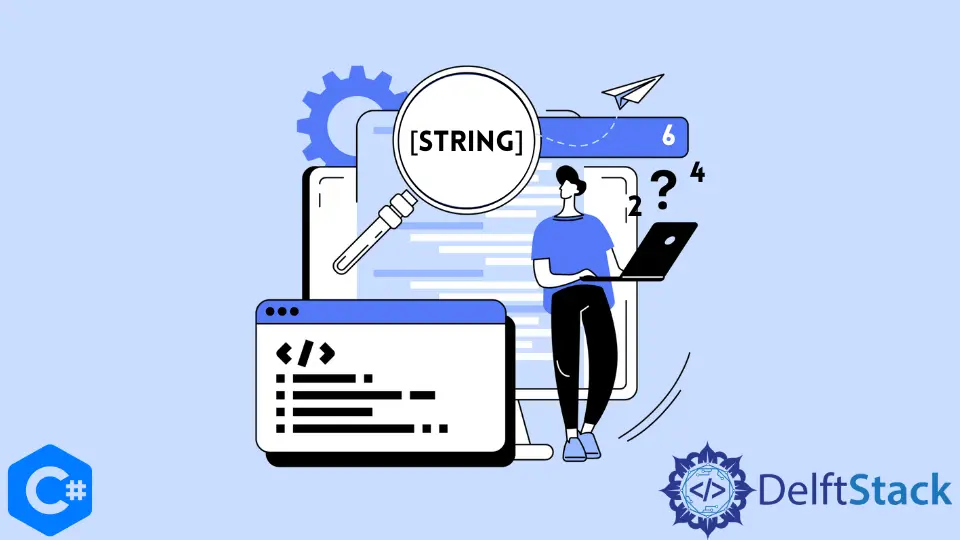
While dealing with real-world problems, sometimes, we face a problem where we want to take input as a string
but use it as an integer
. To make it possible we always have to confirm either the input string
is a number or not.
In C#, we can use many methods to identify if an input string
is a number or not. You will discover these methods while reading this article till the end.
C# Program to Identify if a string
Is a Number Using Enumerable.All()
Method
Enumerable.All()
method belongs to LINQ
. LINQ
is a part of C# and is used to access different databases and data sources. We can modify this method to check if a string
is a number. We will pass char.IsDigit()
method as a parameter to Enumerable.All()
method.
The correct syntax to use this method is as follows:
StringName.All(char.IsDigit);
Example Code:
using System;
using System.Linq;
public class IdentifyString {
public static void Main() {
string number = "123456";
if (number.All(char.IsDigit)) {
Console.WriteLine("The Given String is a Number.");
} else {
Console.WriteLine("The Given String is Not a Number.");
}
}
}
Output:
The Given String is a Number.
C# Program to Identify if a string
Is a Number Using Regex.IsMatch()
Method
In C# we can use regular expressions
to check various patterns. A regular expression
is a specific pattern to perform a specific action. In C#, we have ^[0-9]+$
and ^\d+$
regular expressions to check if a string
is a number.
The correct syntax to use this method is as follows:
Regex.IsMatch(StringName, @"Expression");
Example Code:
using System;
using System.Text.RegularExpressions;
public class IdentifyString {
public static void Main() {
string number = "123456";
if (Regex.IsMatch(number, @"^[0-9]+$")) {
Console.WriteLine("The Given String is a Number.");
} else {
Console.WriteLine("The Given String is Not a Number.");
}
}
}
Output:
The Given String is a Number.
Here, the important point is that the two regular expressions
, ^[0-9]+$
and ^\d+$
are not the same in their functioning. ^[0-9]+$
is used for the basic 0-9 characters but ^\d+$
is used for Decimal digit
characters in the Unicode, without RegexOptions.ECMAScript
specified(default). For example, ๔
as 4
in Thai, is also identified as a number.
C# Program to Identify if a string
Is a Number Using Int32.TryParse()
Method
Int32.TryParse()
method is used to convert the string
of numbers into a 32-bit signed integer
. If the string
is not numeric, it is not converted successfully, and hence this method returns false.
The correct syntax to use this method is as follows:
Int32.TryParse(StringName, out intvariable);
Here intvariable
is any non-initialized integer
variable.
Example Code:
using System;
public class IdentifyString {
public static void Main() {
int n;
string number = "123456";
bool result = Int32.TryParse(number, out n);
if (result) {
Console.WriteLine("The Given String is a Number.");
} else {
Console.WriteLine("The Given String is Not a Number.");
}
}
}
Output:
The Given String is a Number.
C# Program to Identify if a string
Is a Number Using foreach
Loop
This is the most basic process to identify if a string
is a number. In this process, we will check every character of the string
to be a number using the foreach
loop.
The correct syntax to use foreach
loop is as follows:
foreach (datatype variablename in somecollection) {
// steps to iterate
}
Example Code:
using System;
public class IdentifyString {
// custom method to check if a string is a number
public static bool CustomMethod(string number) {
foreach (char c in number) {
if (c >= '0' && c <= '9') {
return true;
}
}
return false;
}
public static void Main() {
string number = "123456";
if (CustomMethod(number)) {
Console.WriteLine("The Given String is a Number.");
} else {
Console.WriteLine("The Given String is Not a Number.");
}
}
}
Output:
The Given String is a Number.