C# 识别字符串是否为数字
Minahil Noor
2023年10月12日
Csharp
Csharp String
-
C# 使用
Enumerable.All()
方法来识别字符串是否为数字 -
C# 使用
Regex.IsMatch()
方法来识别字符串是否为数字 -
C# 使用
Int32.TryParse()
方法来识别字符串是否为数字 -
C# 使用
foreach
循环来识别字符串是否为数字
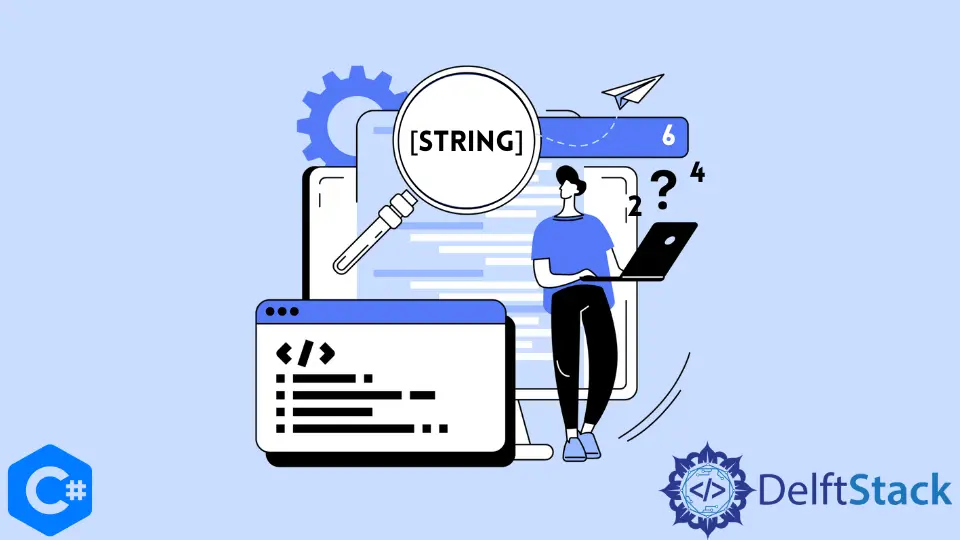
在处理现实世界中的问题时,我们希望将输入作为字符串,而将其用作整数。为了使之成为可能,我们总是必须确认输入的字符串是否为数字。
在 C# 中,我们可以使用许多方法来识别输入字符串是否为数字。
C# 使用 Enumerable.All()
方法来识别字符串是否为数字
Enumerable.All()
方法属于 LINQ。LINQ 是 C# 的一部分,用于访问不同的数据库和数据源。我们可以修改此方法以检查字符串是否为数字。我们将把 char.IsDigit()
方法作为参数传递给 Enumerable.All()
方法。
此方法的正确语法如下:
StringName.All(char.IsDigit);
示例代码:
using System;
using System.Linq;
public class IdentifyString {
public static void Main() {
string number = "123456";
if (number.All(char.IsDigit)) {
Console.WriteLine("The Given String is a Number.");
} else {
Console.WriteLine("The Given String is Not a Number.");
}
}
}
输出:
The Given String is a Number.
C# 使用 Regex.IsMatch()
方法来识别字符串是否为数字
在 C# 中,我们可以使用正则表达式来检查各种模式。正则表达式是执行特定动作的特定模式。在 C# 中,我们使用^[0-9]+$
和^\d+$
正则表达式来检查字符串是否为数字。
此方法的正确语法如下:
Regex.IsMatch(StringName, @"Expression");
示例代码:
using System;
using System.Text.RegularExpressions;
public class IdentifyString {
public static void Main() {
string number = "123456";
if (Regex.IsMatch(number, @"^[0-9]+$")) {
Console.WriteLine("The Given String is a Number.");
} else {
Console.WriteLine("The Given String is Not a Number.");
}
}
}
输出:
The Given String is a Number.
在这里,重要的一点是两个正则表达式 ^[0-9]+$
和 ^\d+$
的功能并不相同。^[0-9]+$
用于基本的 0-9 字符,而^\d+$
用于 Unicode 中的十进制数字,未指定 RegexOptions.ECMAScript
的类别。例如,泰语中的 ๔
为 4
,也被标识为数字。
C# 使用 Int32.TryParse()
方法来识别字符串是否为数字
Int32.TryParse()
方法用于将数字的字符串转换为 32 位带符号整数。如果字符串不是数字,则不会成功转换,因此此方法返回 false。
此方法的正确语法如下:
Int32.TryParse(StringName, out intvariable);
这里的 intvariable
是任何未初始化的 integer
变量。
示例代码:
using System;
public class IdentifyString {
public static void Main() {
int n;
string number = "123456";
bool result = Int32.TryParse(number, out n);
if (result) {
Console.WriteLine("The Given String is a Number.");
} else {
Console.WriteLine("The Given String is Not a Number.");
}
}
}
输出:
The Given String is a Number.
C# 使用 foreach
循环来识别字符串是否为数字
这是识别字符串是否为数字的最基本过程。在这个过程中,我们将使用 foreach
循环将字符串的每个字符检查为数字。
使用 foreach
循环的正确语法如下:
foreach (datatype variablename in somecollection) {
// steps to iterate
}
示例代码:
using System;
public class IdentifyString {
// custom method to check if a string is a number
public static bool CustomMethod(string number) {
foreach (char c in number) {
if (c >= '0' && c <= '9') {
return true;
}
}
return false;
}
public static void Main() {
string number = "123456";
if (CustomMethod(number)) {
Console.WriteLine("The Given String is a Number.");
} else {
Console.WriteLine("The Given String is Not a Number.");
}
}
}
输出:
The Given String is a Number.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe