How to Repeat String in C#
-
Repeat String With the
String
Class Constructor inC#
-
Repeat String With the LINQ Method in
C#
-
Repeat String With the
StringBuilder
Class inC#
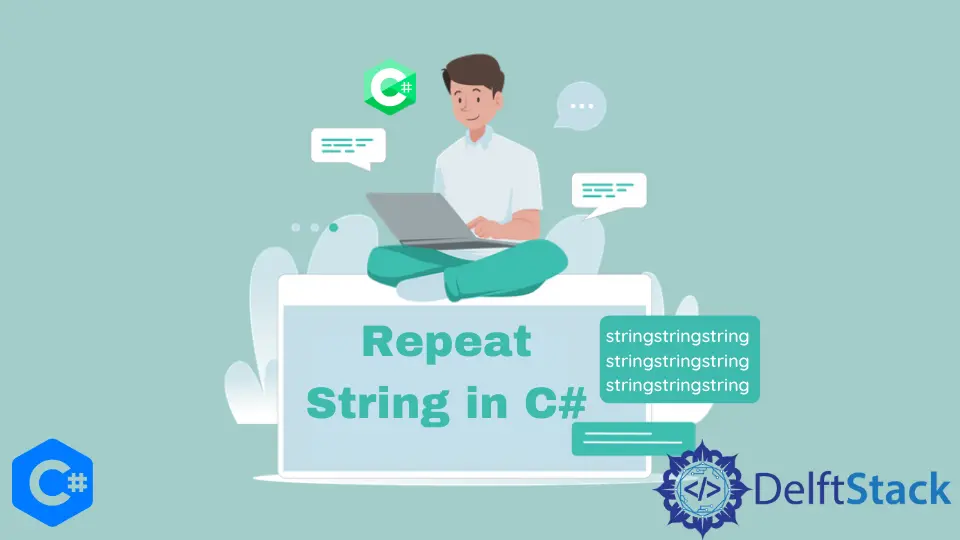
This tutorial will discuss the methods to repeat a string in C#.
Repeat String With the String
Class Constructor in C#
The constructor of the String
class can be used to repeat a specific string to a specified number of times in C#. The String
class constructor takes two arguments, the character to be repeated and the number of times it should repeat. See the following code example.
using System;
namespace repeat_string {
class Program {
static void Main(string[] args) {
string dimensions = new String('D', 3);
Console.WriteLine(dimensions);
}
}
}
Output:
DDD
In the above code, we repeated the character D
3 times and saved it in the string variable dimensions
with the String('D', 3)
constructor in C#. This approach’s drawback is that we cannot repeat any strings; we can repeat a character. This drawback is addressed in the next section.
Repeat String With the LINQ Method in C#
The LINQ integrates query functionality with data structures in C#. The Enumerable.Repeat()
function of LINQ can be used to repeat a string to a specified number of times in C#. The Enumerable.Repeat()
function takes two parameters, a string variable and the number of times that string variable must be repeated. The following code example shows us how we can repeat a string with the Enumerable.Repeat()
function of LINQ in C#.
using System;
using System.Linq;
namespace repeat_string {
class Program {
static void Main(string[] args) {
string alphabets = string.Concat(Enumerable.Repeat("ABC", 3));
Console.WriteLine(alphabets);
}
}
}
Output:
ABCABCABC
In the above code, we repeated the string ABC
3 times and saved it in the string variable alphabets
with the Enumerable.Repeat("ABC", 3)
function of LINQ in C#. This approach repeats strings instead of repeating characters like the previous approach.
Repeat String With the StringBuilder
Class in C#
Another approach that can be used to repeat a string is to use the StringBuilder
class in C#. The StringBuilder
class is used to create a mutable string of characters in C#. See the following code example.
using System;
using System.Text;
namespace repeat_string {
class Program {
static void Main(string[] args) {
string alphabets = new StringBuilder("ABC".Length * 3).Insert(0, "ABC", 3).ToString();
Console.WriteLine(alphabets);
}
}
}
Output:
ABCABCABC
In the above code, we repeated the string ABC
3 times and stored it in the string variable alphabets
with the StringBuilder
class and StringBuilder.Insert()
function in C#. First, we created a new string with the combined length of the repeated string with the StringBuilder("ABC".Length*3)
constructor. It created a string of length 9 because the length of ABC
is 3, and it must be repeated 3 times. Then, we start from the index 0
and insert the string ABC
3 times into the newly created string with the Insert()
function. In the end, we convert the resultant value to a string with the ToString()
function in C#. This approach is not recommended because it does the same job as the previous approach but is more complex.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn