在 C# 中重複字串
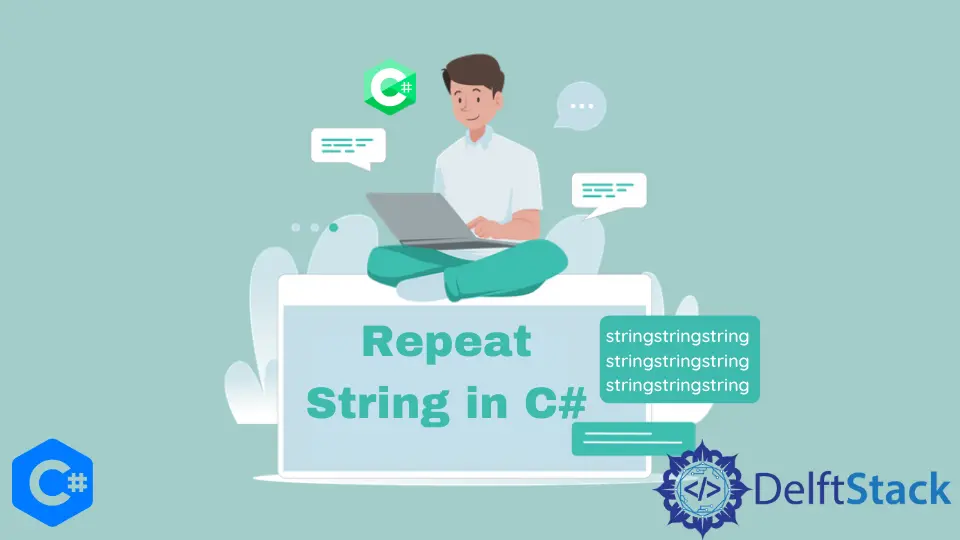
本教程將討論在 C# 中重複字串的方法。
在 C# 中使用 String
類建構函式重複字串
String
類的建構函式可用於在 C# 中將特定字串重複指定次數。String
類建構函式帶有兩個引數,即要重複的字元和應重複的次數。請參見以下程式碼示例。
using System;
namespace repeat_string {
class Program {
static void Main(string[] args) {
string dimensions = new String('D', 3);
Console.WriteLine(dimensions);
}
}
}
輸出:
DDD
在上面的程式碼中,我們重複了字元 D
3 次,並使用 C# 中的 String('D', 3)
建構函式將其儲存在字串變數 dimensions
中。這種方法的缺點是我們不能重複任何字串。我們可以重複一個字元。下一部分將解決此缺點。
在 C# 中使用 LINQ 方法重複字串
LINQ 將查詢功能與 C# 中的資料結構整合在一起。LINQ 的 Enumerable.Repeat()
函式可用於在 C# 中將字串重複指定的次數。Enumerable.Repeat()
函式有兩個引數,一個字串變數和該字串變數必須重複的次數。下面的程式碼示例向我們展示瞭如何在 C# 中使用 LINQ 的 Enumerable.Repeat()
函式重複一個字串。
using System;
using System.Linq;
namespace repeat_string {
class Program {
static void Main(string[] args) {
string alphabets = string.Concat(Enumerable.Repeat("ABC", 3));
Console.WriteLine(alphabets);
}
}
}
輸出:
ABCABCABC
在上面的程式碼中,我們重複了字串 ABC
3 次,並使用 C# 中 LINQ 的 Enumerable.Repeat("ABC", 3)
函式將其儲存在字串變數 alphabets
中。這種方法重複字串,而不是像以前的方法那樣重複字元。
在 C# 中使用 StringBuilder
類重複字串
可以用來重複字串的另一種方法是在 C# 中使用 StringBuilder
類。StringBuilder
類用於在 C# 中建立可變的字串。請參見以下程式碼示例。
using System;
using System.Text;
namespace repeat_string {
class Program {
static void Main(string[] args) {
string alphabets = new StringBuilder("ABC".Length * 3).Insert(0, "ABC", 3).ToString();
Console.WriteLine(alphabets);
}
}
}
輸出:
ABCABCABC
在上面的程式碼中,我們重複了字串 ABC
3 次,並使用 StringBuilder
類和 C# 中的 StringBuilder.Insert()
函式將其儲存在字串變數 alphabets
中。首先,我們使用 StringBuilder("ABC".Length*3)
建構函式建立了一個具有重複字串總長度的新字串。它建立了一個長度為 9 的字串,因為 ABC
的長度為 3,並且必須重複 3 次。然後,我們從索引 0
開始,並使用 Insert()
函式將字串 ABC
插入 3 次到新建立的字串中。最後,我們使用 C# 中的 ToString()
函式將結果值轉換為字串。不建議使用此方法,因為它與以前的方法具有相同的功能,但是更復雜。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn