How to Repeat String X Times in C#
-
Repeat a String X Times in C# With the
string
Class Constructor -
Repeat a String X Times in C# With the
StringBuilder
Class - Repeat a String X Times in C# With the LINQ Method
-
Repeat a String X Times in C# Using a
for
Loop -
Repeat a String X Times in C# Using
string.Concat
andEnumerable.Range
-
Repeat a String X Times in C# Using
string.Join
and an Array of Strings - Conclusion
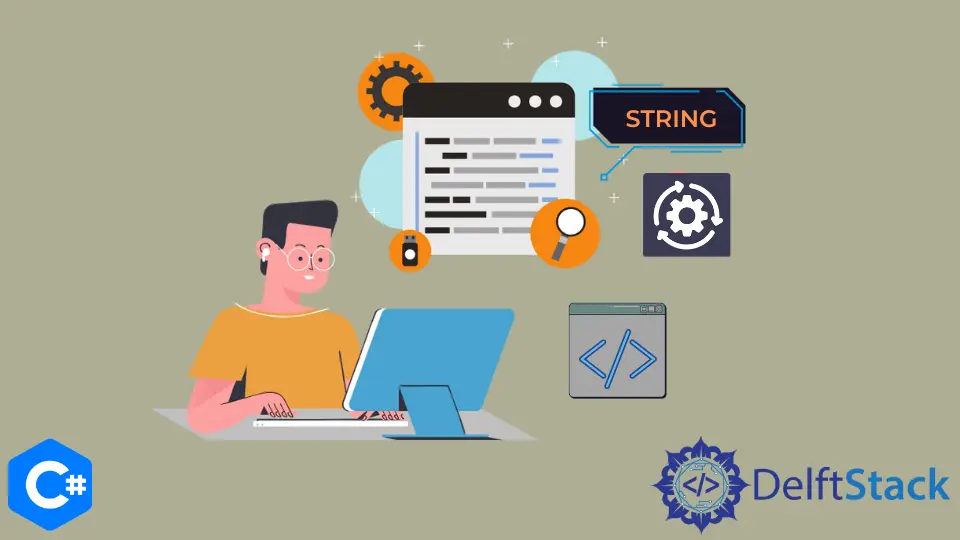
In C#, repeating a string a specific number of times is a common programming task with several approaches to achieve the desired result. Each method has its advantages, and the choice depends on your specific use case and coding style.
In this article, we will explore five different methods to repeat a string x
times in C# and discuss when to use each one.
Repeat a String X Times in C# With the string
Class Constructor
The constructor of the string
class can be used to repeat a specific character to a specified number of times inside a string in C#. We can pass the character to be repeated and the number of times it should be repeated to the constructor of the string
class in C#.
The string(c, x)
constructor gives us a string where the character c
is repeated x
times. See the following code example.
using System;
namespace repeat_string_x_times
{
class Program
{
static void Main(string[] args)
{
string str = new string('e', 3);
Console.WriteLine(str);
}
}
}
Output:
eee
In the above code, we repeated the character e
three times and saved it inside the string variable str
with the string('e', 3)
constructor in C#. This method can only be used to repeat a single character x times inside a string.
Repeat a String X Times in C# With the StringBuilder
Class
The StringBuilder class in C# provides a powerful mechanism to create mutable strings. Unlike the string
class, which is immutable, StringBuilder
allows for in-place modifications, making it highly efficient for string concatenation, especially when dealing with repetitive operations.
To repeat a string using StringBuilder
, we can utilize the Insert
method, which allows us to insert a string a specified number of times at a specified position within the StringBuilder
.
Here’s the syntax of the Insert
method:
public StringBuilder Insert(int startIndex, string value, int count);
Parameters:
startIndex
: The index at which the insertion will start within theStringBuilder
. The first character has an index of 0.value
: The string or character sequence to be inserted.count
: The number of times thevalue
should be inserted.
The StringBuilder
class is more efficient for concatenating strings, especially when dealing with a large number of repetitions.
Let’s begin by examining the provided code example:
using System;
using System.Text;
namespace repeat_string_x_times
{
class Program
{
static void Main(string[] args)
{
string str = new StringBuilder("TEXT".Length * 3).Insert(0, "TEXT", 3).ToString();
Console.WriteLine(str);
}
}
}
Output:
TEXTTEXTTEXT
In this example, we start by initializing a StringBuilder
object with a preallocated capacity based on the length of the original string "TEXT"
multiplied by the desired repetition count (3
times).
string str = new StringBuilder("TEXT".Length * 3).Insert(0, "TEXT", 3).ToString();
Then, the Insert
method of StringBuilder
is used to insert the original string "TEXT"
a specified number of times (3
times) at the beginning (index 0
) of the StringBuilder
.
new StringBuilder("TEXT".Length * 3).Insert(0, "TEXT", 3)
Finally, we convert the StringBuilder
to a regular string using the ToString
method.
string str = new StringBuilder("TEXT".Length * 3).Insert(0, "TEXT", 3).ToString();
The resulting repeated string is then printed to the console using the following line.
Repeat a String X Times in C# With the LINQ Method
In C#, the Enumerable.Repeat
function, part of the LINQ namespace, is a valuable tool for repeating an item, such as a string, a certain number of times. It creates an enumerable that contains the specified item repeated the specified number of times.
For repeating a string, we can use this function to create an enumerable with the desired string repeated x
times.
Here’s the signature of the Repeat
method:
public static IEnumerable<TSource> Repeat<TSource>(TSource element, int count);
Parameters:
TSource
: The type of the elements in the collection.element
: The element to be repeated in the collection.count
: The number of times to repeat the element.
Let’s examine a sample C# program that demonstrates how to repeat a string using LINQ:
using System;
using System.Linq;
namespace repeat_string_x_times
{
class Program
{
static void Main(string[] args)
{
string str = string.Concat(Enumerable.Repeat("TEXT", 3));
Console.WriteLine(str);
}
}
}
Output:
TEXTTEXTTEXT
In the Main
method, we define a string variable str
to store the resulting repeated string. Here, we utilize Enumerable.Repeat("TEXT", 3)
, a function that generates a sequence repeating the string "TEXT"
three times.
The string.Concat
method comes into play, allowing us to concatenate these repeated strings into a unified string, which we then assign to the str
variable.
Finally, we display the concatenated string, showing the result of repeating the string "TEXT"
three times.
Repeat a String X Times in C# Using a for
Loop
The for
loop in C# is a versatile construct that allows you to iterate over a range of values and execute a set of statements for each iteration.
To repeat a string x
times using a for
loop, we need to initialize a counter variable, set the loop’s termination condition based on x
, and increment the counter within each iteration.
Let’s break down the steps to achieve this:
-
We start by initializing a counter variable to keep track of the number of repetitions. We’ll also declare a variable to hold the original string we want to repeat.
Consider the following:
string originalString = "Hello, "; int repeatCount = 5; // Change this to the desired number of repetitions (x) string repeatedString = "";
-
We then utilize a
for
loop to iteraterepeatCount
times and concatenate the original string to our result in each iteration.for (int i = 0; i < repeatCount; i++) { repeatedString += originalString; }
In this
for
loop:i
is the loop counter variable, starting from 0.- The loop continues as long as
i
is less thanrepeatCount
. - In each iteration, we concatenate
originalString
torepeatedString
.
-
Finally, we display the resulting repeated string.
Console.WriteLine("Resulting String: " + repeatedString);
Let’s put the above steps together into a complete C# program to demonstrate how to repeat a string x
times using a for
loop:
using System;
class Program
{
static void Main(string[] args)
{
string originalString = "Hello, ";
int repeatCount = 5;
string repeatedString = "";
for (int i = 0; i < repeatCount; i++)
{
repeatedString += originalString;
}
Console.WriteLine("Resulting String: " + repeatedString);
}
}
Output:
Resulting String: Hello, Hello, Hello, Hello, Hello,
Here’s how the code works:
In the Main
method, three variables are initialized:
originalString
: Contains the base string to be repeated ("Hello, "
in this case).repeatCount
: An integer representing the number of times the string should be repeated.repeatedString
: Initially an empty string to hold the resulting repeated string.
The for
loop iterates repeatCount
times, with the loop counter i
starting at 0 and incrementing by 1 in each iteration. Within the loop, the originalString
is concatenated to repeatedString
, effectively repeating the string repeatCount
times.
Finally, the program outputs the resulting repeated string using Console.WriteLine
. The output displays the concatenated string, showcasing the repetition achieved based on the value of repeatCount
.
While simple and intuitive, this method can be less efficient for a large number of repetitions due to string concatenation’s inherent overhead.
Repeat a String X Times in C# Using string.Concat
and Enumerable.Range
The string.Concat
method in C# allows you to concatenate multiple strings into a single string. It is a static method that takes an IEnumerable<string>
as a parameter, enabling you to concatenate a collection of strings seamlessly.
This method creates a new string that contains the concatenated values of the input strings.
Here’s the basic syntax of string.Concat
:
public static string Concat(IEnumerable<string> values);
Parameter:
values
: AnIEnumerable<string>
that contains the strings to concatenate.
On the other hand, Enumerable.Range
is a method in C# provided by the LINQ (Language Integrated Query) extension methods.
It generates a sequence of integers within a specified range. The method takes two parameters: the start index and the number of elements in the sequence.
Here’s the basic syntax of Enumerable.Range
:
public static IEnumerable<int> Range(int start, int count);
Parameters:
start
: The starting value of the sequence.count
: The number of elements to generate in the sequence.
Combining these two components (string.Concat
and Enumerable.Range
), we can create a sequence of the original string repeated x
times and then concatenate them into a single string using string.Concat
.
Follow these steps to repeat a string using string.Concat
and Enumerable.Range
:
-
Start by utilizing
Enumerable.Range
to generate a sequence of integers representing the desired repeat count, denoted asx
. -
For each integer in the generated sequence, repeat the original string.
-
Leverage
string.Concat
to concatenate the sequence of repeated strings into one cohesive final string.
Let’s put the steps into action with a complete C# code example:
using System;
class Program
{
static void Main(string[] args)
{
string originalString = "Hi, ";
int repeatCount = 5; // Change this to the desired number of repetitions (x)
var repeatedStrings = Enumerable.Range(0, repeatCount)
.Select(i => originalString);
string result = string.Concat(repeatedStrings);
Console.WriteLine("Resulting String: " + result);
}
}
Output:
Resulting String: Hi, Hi, Hi, Hi, Hi,
First, we set the original string we want to repeat and specify how many times we want to repeat it by setting repeatCount
.
Then, we use Enumerable.Range
to generate a sequence of integers from 0 up to repeatCount - 1
. Each integer in this sequence represents an iteration to repeat the original string.
Using the Select
method, we map these integers to the original string, essentially repeating it for each iteration. Afterward, we use string.Concat
to concatenate all these repeated strings into a single string.
Finally, we display this resulting repeated string using Console.WriteLine
.
Repeat a String X Times in C# Using string.Join
and an Array of Strings
We’ll use an array of strings to store the original string x
times. Then, we’ll utilize string.Join
to concatenate these strings into a single string.
string.Join
is a powerful method in C# that allows us to concatenate an array of strings using a specified separator if needed.
Here are the steps to repeat a string using string.Join
and an array of strings:
-
Begin by creating an array of strings where each element is the original string you want to repeat. The number of elements in this array should correspond to the desired number of repetitions, denoted as
x
. -
Employ the
string.Join
method to concatenate the strings from the array into one final string. This method takes an array of strings and concatenates them, providing a seamless way to repeat the original string.
Let’s exemplify the steps using a complete C# code example:
using System;
class Program
{
static void Main(string[] args)
{
string originalString = "OK, ";
int repeatCount = 4; // Change this to the desired number of repetitions (x)
string[] repeatedStrings = new string[repeatCount];
for (int i = 0; i < repeatCount; i++)
{
repeatedStrings[i] = originalString;
}
string result = string.Join("", repeatedStrings);
Console.WriteLine("Resulting String: " + result);
}
}
Output:
Resulting String: OK, OK, OK, OK,
In this C# code, we start by setting up the originalString
variable to hold the string we want to repeat, in this case, "OK, "
. The variable repeatCount
is set to 4
, indicating how many times we want to repeat the originalString
.
To achieve this repetition, we create an array of strings called repeatedStrings
with a length equal to the repeatCount
. This array will hold our repeated strings.
We then use a for
loop to iterate repeatCount
times.
In each iteration, we assign the originalString
to an element in the repeatedStrings
array. Essentially, we’re filling the array with repetitions of the originalString
.
Next, we utilize the string.Join
method to concatenate the strings from the repeatedStrings
array into a single string. In this case, we use an empty string as the separator, meaning we concatenate them without any additional characters between the repeated strings.
Finally, we display the resulting repeated string using Console.WriteLine
, making it visible to us in the console.
Conclusion
The best method to repeat a string x
times depends on your specific requirements. You can use the for
loop for simplicity and small repetitions and StringBuilder
for better performance, especially with a large number of repetitions.
Utilize LINQ and Enumerable.Repeat
for a concise and expressive approach and Enumerable.Range
with string.Concat
for a more functional-style approach.
You can also use string.Join
with an array of strings for a simple and intuitive solution. Select the method that aligns with your needs in terms of efficiency, readability, and coding style.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn