在 C# 中重複字串 X 次
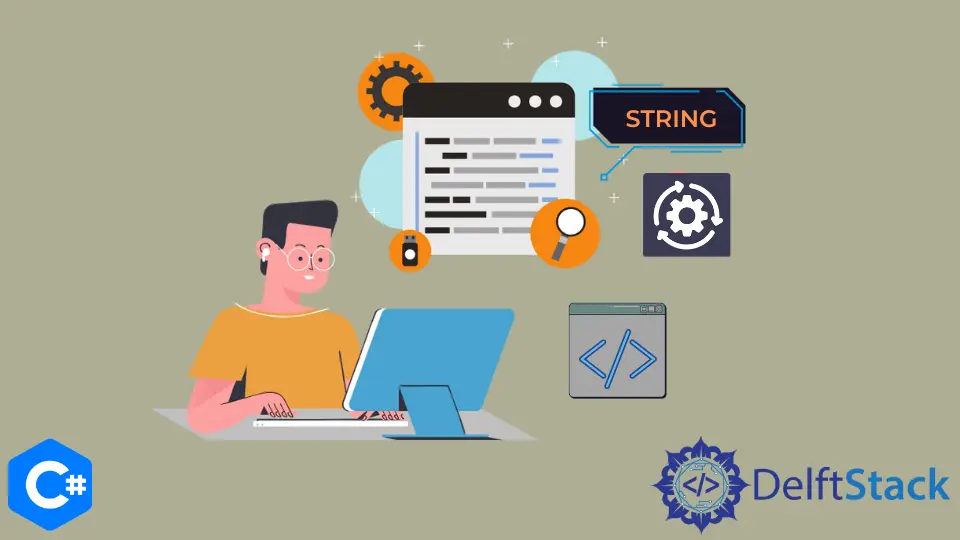
本教程將介紹在 C# 中將字串重複 x 次的方法。
用 C# 中的 string
類建構函式重複執行 X 次字串
string
類的建構函式可用於在 C# 的字串中將特定字元重複指定的次數。我們可以將要重複的字元及其應重複的次數傳遞給 C# 中的 string
類的建構函式。string(c, x)
建構函式為我們提供了一個字串,其中字元 c
重複了 x
次。請參見以下程式碼示例。
using System;
namespace repeat_string_x_times {
class Program {
static void Main(string[] args) {
string str = new string('e', 3);
Console.WriteLine(str);
}
}
}
輸出:
eee
在上面的程式碼中,我們重複了字元 e
3 次,並使用 C# 中的 string('e', 3)
建構函式將其儲存在字串變數 str
中。此方法只能用於在字串中重複單個字元 x 次。
使用 C# 中的 StringBuilder
類將字串重複 X 次
StringBuilder
類還可以用於在 C# 中將字串重複 x 次。StringBuilder
類在 C# 中建立一個可變長度的可變字串,該字串具有一定的長度。然後,我們可以使用 StringBuilder.Insert(s,x)
函式插入字串 s
並重復 x
次。請參見以下程式碼示例。
using System;
using System.Text;
namespace repeat_string_x_times {
class Program {
static void Main(string[] args) {
string str = new StringBuilder("TEXT".Length * 3).Insert(0, "TEXT", 3).ToString();
Console.WriteLine(str);
}
}
}
輸出:
TEXTTEXTTEXT
在上面的程式碼中,我們重複了字串 TEXT
3 次,並使用 C# 中的 StringBuilder
類將其儲存在字串變數 str
中。我們首先建立了一個可變字串,其組合長度為 TEXT.Length * 3
個字元。然後,我們從索引 0
開始,將字串 TEXT
插入可變字串內 3 次。然後,使用 C# 中的 ToString()
函式,將可變字串轉換為常規字串。此方法可用於重複字串,而不是先前方法中的字元。
在 C# 中使用 LINQ 方法將字串重複 X 次
LINQ(也稱為語言整合查詢)用於將 SQL 查詢功能與 C# 中的資料結構整合在一起。我們可以使用 LINQ 的 Enumerable.Repeat()
函式在 C# 中重複一個字串 x 次。Enumerable.Repeat(s, x)
函式有兩個引數,字串變數 s
和整數變數 x
,即字串變數必須重複的次數。請參見以下程式碼示例。
using System;
using System.Linq;
namespace repeat_string_x_times {
class Program {
static void Main(string[] args) {
string str = string.Concat(Enumerable.Repeat("TEXT", 3));
Console.WriteLine(str);
}
}
}
輸出:
TEXTTEXTTEXT
在上面的程式碼中,我們重複了字串 TEXT
3 次,並使用 C# 中 LINQ 的 Enumerable.Repeat("TEXT", 3)
函式將其儲存在字串變數 str
中。我們使用 Enumerable.Repeat()
函式重複該字串,並使用 String.Concat()
函式將這些值連線為一個字串。建議使用此方法,因為它與以前的方法具有相同的作用,並且相對簡單。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn