How to Convert String Array to Int Array in C#
-
Use the
Array.ConvertAll()
Method to Convert String Array to Int Array in C# -
Use LINQ’s
Select()
Method andint.Parse
to Convert String Array to Int Array in C# -
Use LINQ’s
Select()
Method andint.TryParse
to Convert String Array to Int Array in C# - Conclusion
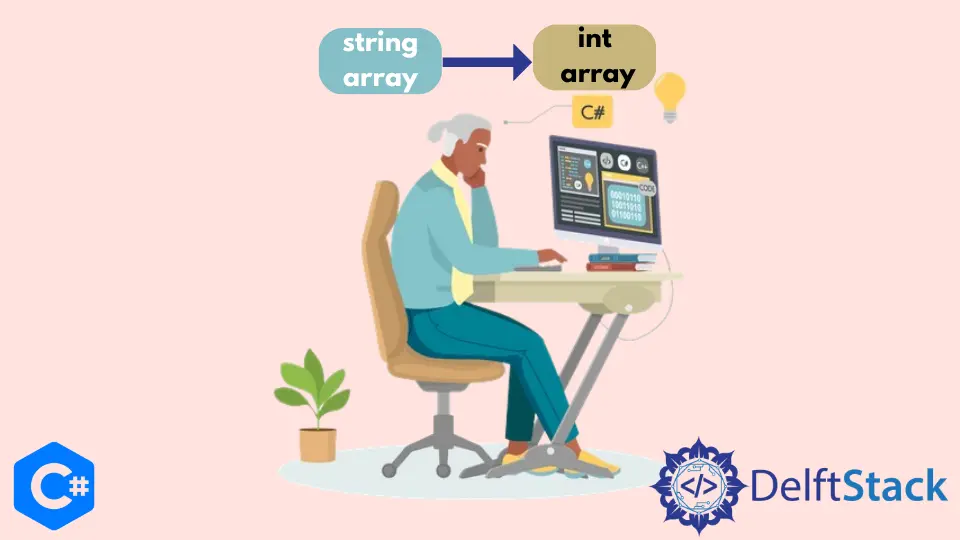
This guide will teach us to convert a string array to an int array in C#.
Let’s discuss the three ways to convert string to int. These methods are quite simple and are very easy to implement.
Use the Array.ConvertAll()
Method to Convert String Array to Int Array in C#
This method employs the Array.ConvertAll()
method to seamlessly convert a string array to an integer array in C#. The ConvertAll()
method streamlines the conversion process by applying a specified conversion function to each element of the string array.
In the following code, we use the Array.Convertall()
method and pass the array. We are parsing the string array into an int array using int.Parse()
.
Basic Syntax:
string[] stringArray = { "10", "20", "30", "40", "50" };
int[] intArray = Array.ConvertAll(stringArray, int.Parse);
Parameters:
- Source Array (
stringArray
): This is the array you want to convert. In this case,stringArray
is a string array containing numerical values represented as strings. - Converter Function (
int.Parse
): This is the method or delegate that defines how each element of the source array is converted to the target type. In this example,int.Parse
is a method that converts each string element of thestringArray
to an integer.
using System;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int = Array.ConvertAll(temp_str, s => int.Parse(s));
for (int i = 0; i < temp_int.Length; i++) {
Console.WriteLine(temp_int[i]);
}
Console.ReadKey();
}
}
}
Output:
1000
2000
3000
Use LINQ’s Select()
Method and int.Parse
to Convert String Array to Int Array in C#
This method uses LINQ’s Select()
method along with int.Parse
to swiftly convert a string array to an integer array in C#. The Select()
method efficiently transforms each element of the string array into its integer equivalent using the int.Parse
function.
This concise and readable approach simplifies the conversion process, making it a convenient choice for scenarios where a direct conversion from string to integer is appropriate.
Basic Syntax:
string[] stringArray = { "10", "20", "30", "40", "50" };
int[] intArray = stringArray.Select(s => int.Parse(s)).ToArray();
Parameters:
- Source Array (
stringArray
): This is the array of strings that you want to convert to integers. - Projection Function (
int.Parse
): This is the function that defines the conversion from the source type (string
) to the target type (int
). In this case,int.Parse
is used to convert each string element ofstringArray
to an integer. - Result (
intArray
): This is the resulting integer array after applying the projection function to each element of the source array.
using System;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int1 = temp_str.Select(int.Parse).ToArray();
for (int i = 0; i < temp_int1.Length; i++) {
Console.WriteLine(temp_int1[i]);
Console.ReadKey();
}
}
}
}
Output:
1000
2000
3000
Use LINQ’s Select()
Method and int.TryParse
to Convert String Array to Int Array in C#
The method utilizes LINQ’s Select()
method in conjunction with int.TryParse
to efficiently convert a string array to an integer array in C#. The Select()
method projects each element of the string array into an integer, employing int.TryParse
for parsing.
This approach accommodates potential parsing errors by providing a default value in case of failure. The resulting integers are then collected into an array using ToArray()
.
Basic Syntax:
int[] intArray = stringArray.Select(s => int.TryParse(s, out int result)? result: 0).ToArray();
Parameters:
- Source Array (
stringArray
): This is the array of strings that you want to convert to integers. - Projection Function (
s => int.TryParse(s, out int result) ? result : 0
): This is a lambda expression acting as the projection function, where each string elements
is attempted to be parsed into an integer usingint.TryParse
. The expression returns either the parsed result or a default value of0
if parsing fails. - Result (
intArray
): This is the resulting integer array after applying the projection function to each element of the source array.
using System;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int = temp_str.Select(s => int.TryParse(s, out int result) ? result : 0).ToArray();
foreach (int num in temp_int) {
Console.WriteLine(num);
}
Console.ReadKey();
}
}
}
Output:
1000
2000
3000
Conclusion
In conclusion, this guide has provided three simple and easy-to-implement methods for converting a string array to an int array in C#. The first method employs the Array.ConvertAll()
method, streamlining the conversion process by applying a specified conversion function to each element of the string array.
The second method utilizes LINQ’s Select()
method along with int.Parse
, providing a concise and readable approach for direct conversion from a string to an integer. The third method enhances error tolerance by using LINQ’s Select()
method in conjunction with int.TryParse
, allowing for graceful handling of parsing failures with a default value.
Each method is exemplified with clear syntax and parameters, making it accessible for developers to choose the approach that best fits their requirements.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn