C#에서 문자열 배열을 Int 배열로 변환
-
Array.ConvertAll()
메서드를 사용하여C#
에서 문자열 배열을 Int 배열로 변환 -
LINQ의
Select()
메서드를 사용하여C#
에서 문자열 배열을 Int 배열로 변환
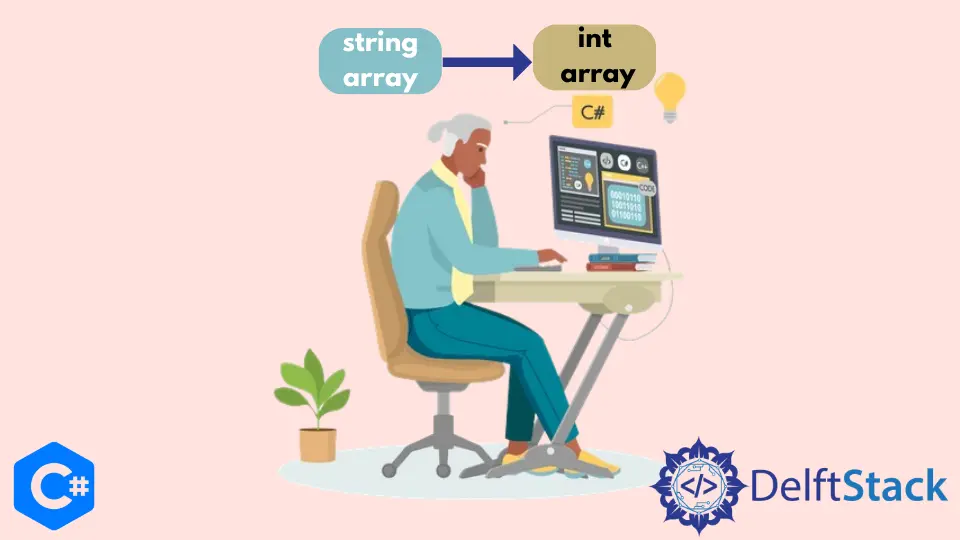
이 가이드에서는 C#에서 string형 배열을 int 배열로 변환하는 방법을 알려줍니다.
String을 int로 변환하는 두 가지 방법이 있습니다. 이 두 가지 방법 모두 매우 간단하고 구현하기가 매우 쉽습니다.
Array.ConvertAll()
메서드를 사용하여 C#
에서 문자열 배열을 Int 배열로 변환
내용을 이해하여 문자열을 다른 데이터 유형으로 변환하는 것에 대해 이야기할 때마다 구문 분석이 필요합니다. 예를 들어, 문자열 321
은 321로 변환될 수 있습니다.
사용할 수 있는 첫 번째 방법은 Array.ConvertAll()
방법입니다. 이 메소드의 구현을 봅시다.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
// method 1 using Array.ConvertAll
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int = Array.ConvertAll(temp_str, s => int.Parse(s));
for (int i = 0; i < temp_int.Length; i++) {
Console.WriteLine(temp_int[i]); // Printing After Conversion.............
}
Console.ReadKey();
}
}
}
위의 코드에서 Array.Convertall()
메서드를 사용하여 배열을 전달합니다. int.Parse()
를 사용하여 문자열 배열을 int 배열로 구문 분석합니다.
출력:
1000
2000
3000
LINQ의 Select()
메서드를 사용하여 C#
에서 문자열 배열을 Int 배열로 변환
다음 메서드를 사용하여 int.Parse()
메서드를 LINQ의 Select()
메서드에 전달하여 문자열 배열을 int 배열로 변환할 수 있습니다. 배열을 가져오려면 ToArray()
메서드도 호출해야 합니다.
구현을 봅시다. 여기에서 LINQ에 대해 자세히 알아보세요.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
Console.WriteLine("===================Using LINQ=======================================");
// Method Using LINQ.........................
// We can also pass the int.Parse() method to LINQ's Select() method and then call ToArray to
// get an array.
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int1 = temp_str.Select(int.Parse).ToArray();
for (int i = 0; i < temp_int1.Length; i++) {
Console.WriteLine(temp_int1[i]); // Printing After Conversion.............
}
Console.ReadKey();
}
}
}
출력:
===================Using LINQ=======================================
1000
2000
3000
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn