在 C# 中將字串陣列轉換為 Int 陣列
Haider Ali
2023年12月11日
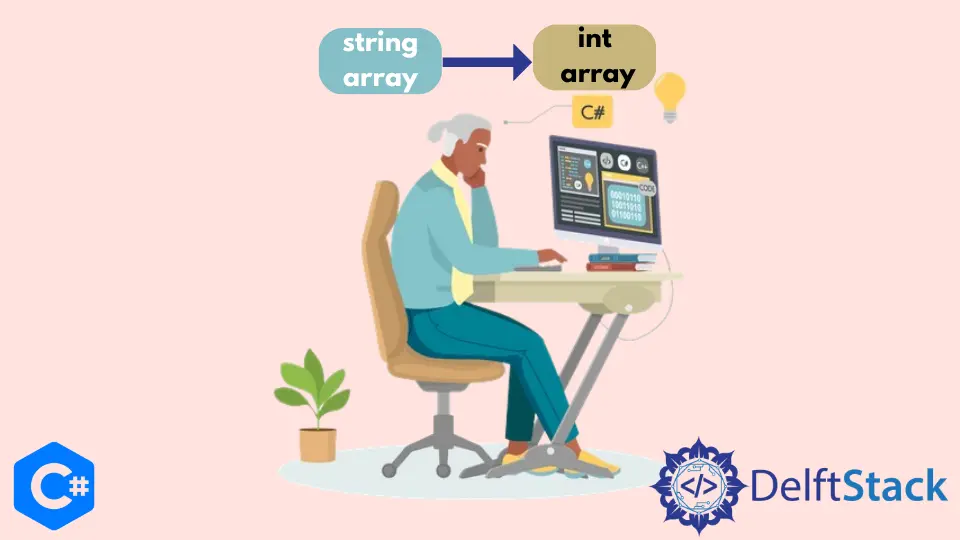
本指南將教我們在 C# 中將字串陣列轉換為 int 陣列。
有兩種方法可以將 String 轉換為 int。這兩種方法都非常簡單,也很容易實現。
在 C#
中使用 Array.ConvertAll()
方法將字串陣列轉換為 Int 陣列
每當我們談論通過理解其內容將字串轉換為不同的資料型別時,都會涉及到解析。例如,字串 321
可以轉換為 321。
你可以使用的第一種方法是 Array.ConvertAll()
方法。讓我們看看這個方法的實現。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
// method 1 using Array.ConvertAll
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int = Array.ConvertAll(temp_str, s => int.Parse(s));
for (int i = 0; i < temp_int.Length; i++) {
Console.WriteLine(temp_int[i]); // Printing After Conversion.............
}
Console.ReadKey();
}
}
}
我們在上面的程式碼中使用了 Array.Convertall()
方法並傳遞陣列。我們正在使用 int.Parse()
將字串陣列解析為一個 int 陣列。
輸出:
1000
2000
3000
在 C#
中使用 LINQ 的 Select()
方法將字串陣列轉換為 Int 陣列
我們可以使用 next 方法將字串陣列轉換為 int 陣列,方法是將 int.Parse()
方法傳遞給 LINQ 的 Select()
方法。我們還需要呼叫 ToArray()
方法來獲取陣列。
讓我們看看實現。在此處瞭解有關 LINQ 的更多資訊。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
Console.WriteLine("===================Using LINQ=======================================");
// Method Using LINQ.........................
// We can also pass the int.Parse() method to LINQ's Select() method and then call ToArray to
// get an array.
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int1 = temp_str.Select(int.Parse).ToArray();
for (int i = 0; i < temp_int1.Length; i++) {
Console.WriteLine(temp_int1[i]); // Printing After Conversion.............
}
Console.ReadKey();
}
}
}
輸出:
===================Using LINQ=======================================
1000
2000
3000
作者: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn