How to Check if TextBox Is Empty in C#
-
Check if a TextBox Is Empty With the
String.IsNullOrEmpty()
Function inC#
-
Check if a TextBox Is Empty With the
TextBox.Text.Length
Property inC#
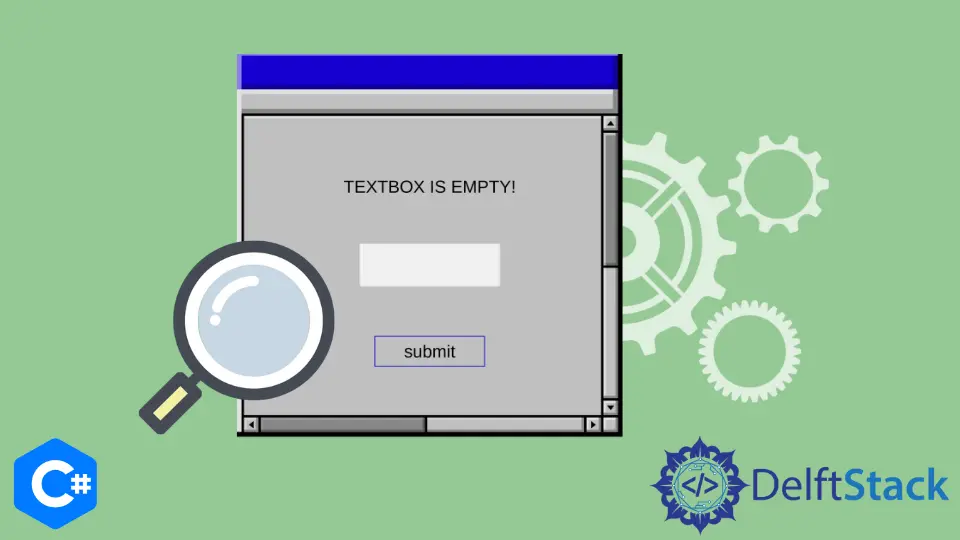
This tutorial will discuss how to check if a text box is empty or not in C#.
Check if a TextBox Is Empty With the String.IsNullOrEmpty()
Function in C#
The String.IsNullOrEmpty()
function checks whether a string is null or empty or not in C#. The String.IsNullOrEmpty()
function has a boolean return type and returns true
if the string is either null
or empty and otherwise returns false
. We can use the String.IsNullOrEmpty()
function on the string inside the TextBox.Text
property to check whether the text inside the text box is empty or not. See the following code example.
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
if (String.IsNullOrEmpty(textBox1.Text)) {
label1.Text = "TEXT BOX IS EMPTY";
}
}
}
}
Output:
In the above code, we checked whether the text box is empty or not with the String.IsEmptyOrNot()
function in C#. We can also use the String.IsNullOrWhitespace()
function to check whether there are whitespaces inside the text box or not, as shown below.
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
if (String.IsNullOrWhitespace(textBox1.Text)) {
label1.Text = "TEXT BOX IS EMPTY";
}
}
}
}
This approach also takes the whitespaces into consideration and displays the error message TEXT BOX IS EMPTY
if there are only whitespaces inside the text box.
Check if a TextBox Is Empty With the TextBox.Text.Length
Property in C#
The TextBox.Text.Length
property gets the length of the text inside the text box in C#. We can use the TextBox.Text.Length == 0
condition inside the if
statement to check if the text box is empty or not. See the following code example.
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
if (textBox1.Text.Length == 0) {
label1.Text = "TEXT BOX IS EMPTY";
}
}
}
}
Output:
In the above code, we checked whether the text box is empty or not with the TextBox.Text.Length
property in C#. This method is not recommended because it does not take whitespaces into consideration.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn