How to Simulate a Key Press in C#
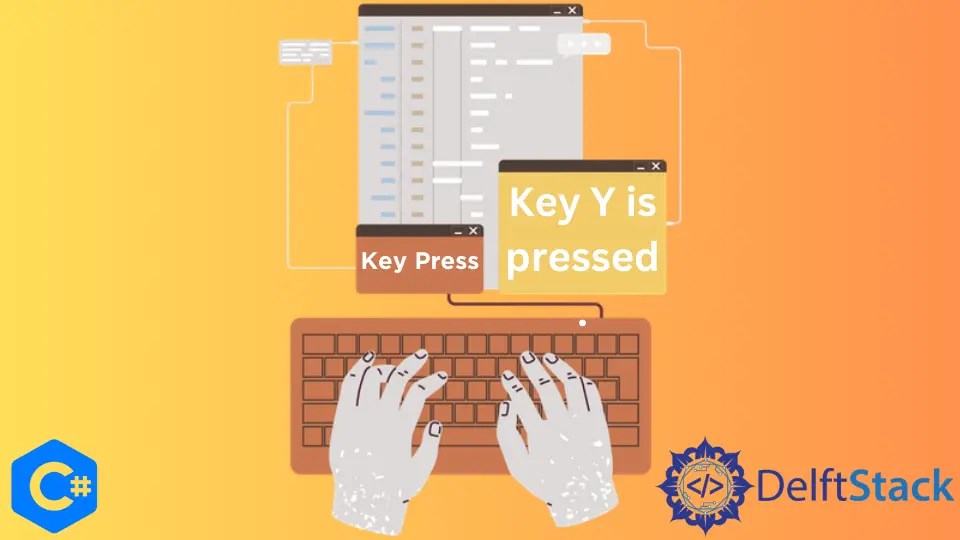
This article demonstrates a quick and straightforward approach to simulate a keypress in C#.
During testing, you may need to transmit a sequence of pushed buttons (a keystroke) to the chosen object - a control or a window. You may simulate keystrokes from keyword tests and scripts by transmitting key codes to the chosen control or window.
Create a Form Application in C#
Firstly, Create a new Windows Form Application in Visual Studio.
Open your .cs
Design File and create a label named numberLabel
and assign it some text that can be "Text To Be Displayed"
, set its alignment to Middle Center to look clean.
Let’s create numPadButton
and alphabetButton
, and add the "Click Me"
text to both buttons. Double click on your numPadButton
, which will navigate you to the numPadButton_Click
function.
Inside this function, assign some text to the numberLabel
. This text will show up once the key we’ll assign is pressed.
private void numPadButton_Click(object sender, EventArgs e) {
numberLabel.Text = "Numpad Button 0 Pressed";
}
Perform the same steps with the alphabetButton
. Inside the alphabetButton_Click
function, assign the numberLabel
some different text as this text will be shown on a different key pressed.
private void alphabetButton_Click(object sender, EventArgs e) {
numberLabel.Text = "Enter Pressed";
}
Output:
We need to enable the KeyPreview
functionality for our form that determines if the keyboard events are registered with the form or not. So, click on the whole form, and from the properties panel on your right, set the KeyPreview
value to true.
Then, we need a KeyDown
event that will identify and handle the keypress event, and to add this, go to the properties panel and open the events tab with a lightning symbol. Scroll down to the KeyDown
event and double-click on it.
It will create a Form1_KeyDown()
method and navigates you to this method. This method takes two arguments, a sender
of type object
and an event e
of type KeyEventArgs
.
private void Form1_KeyDown(object sender, KeyEventArgs e) {}
Now we must use the button click to imitate the pressing of a certain key. This function will receive the pushed key, and we’ll verify if the received key is NumPad0
and then perform the click on numPadButton
.
if (e.KeyCode == Keys.NumPad0) {
numPadButton.PerformClick();
}
With the alphabetButton
, we need to follow the same process. So, from the events tab, double-click the KeyUp
event, and inside the Form1_KeyUp()
function, check if the received key is Enter, then perform the click the alphabetButton
.
using System;
namespace SimulateKeyPress {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void numPadButton_Click(object sender, EventArgs e) {
numberLabel.Text = "Numpad Button 0 Pressed";
}
private void alphabetButton_Click(object sender, EventArgs e) {
numberLabel.Text = "Enter Pressed";
}
private void Form1_KeyDown(object sender, KeyEventArgs e) {
if (e.KeyCode == Keys.NumPad0) {
numPadButton.PerformClick();
}
}
private void Form1_KeyUp(object sender, KeyEventArgs e) {
if (e.KeyCode == Keys.Enter) {
alphabetButton.PerformClick();
}
}
private void numberLabel_Click(object sender, EventArgs e) {}
}
}
Output:
When the Numpad 0 is pressed from the keyboard.
When the Enter is pressed from the keyboard.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn