How to Add Placeholder to a Textbox in C#
-
Create a Textbox With Placeholder Text in
C#
-
Use
Enter
andLeave
Focus Events in Visual Studio IDE to Add Placeholder Text to a Textbox inC#
-
Install
Bunifu UI
in Visual Studio to Add the Textbox With a Placeholder in C# Projects
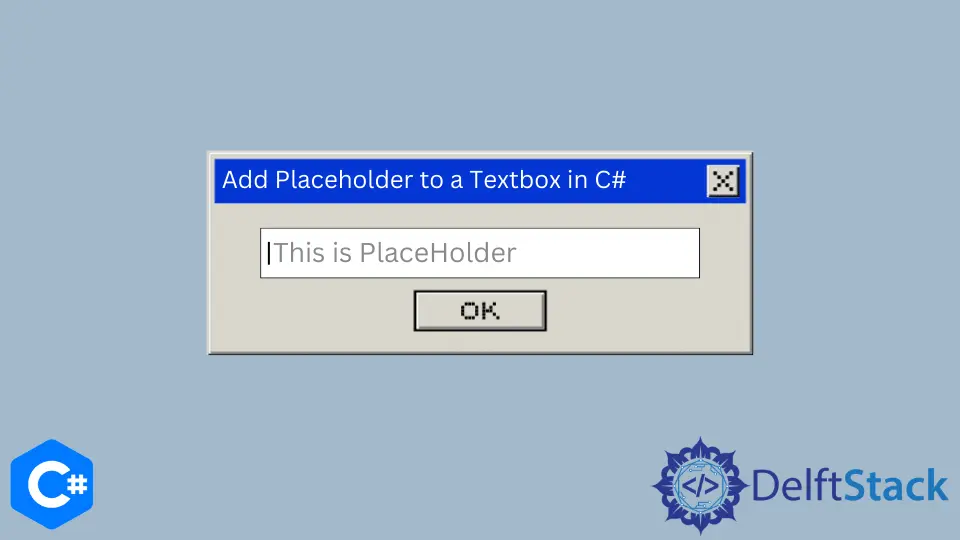
This tutorial will teach the three methods to add a placeholder to a textbox in C#. As placeholders should be treated as a hint, they are not replacements for labels
or the title
.
Create a Textbox With Placeholder Text in C#
Creating a textbox with a placeholder text that disappears and allows the users to enter their text gives freedom of complete customization to developers. Furthermore, it allows you to style the placeholder text of a form element.
Generally, a textbox contains a placeholder; when it gets focused, the placeholder is removed for the user to add text. If the textbox loses the focus and the user hasn’t entered any text, the placeholder is added back to the textbox.
The following C# code will create a textbox with a placeholder.
Form1.Designer.cs
:
// `Form1` as your C# project form
// `Form1.Designer.cs` Code to create a `PlaceholderTxtB` textbox
namespace TextboxPlaceholder {
partial class Form1 {
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing) {
if (disposing && (components != null)) {
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
private void InitializeComponent() {
this.PlaceholderTxtB = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// PlaceholderTxtB
//
this.PlaceholderTxtB.Location = new System.Drawing.Point(12, 12);
this.PlaceholderTxtB.Name = "PlaceholderTxtB";
this.PlaceholderTxtB.Size = new System.Drawing.Size(188, 20);
this.PlaceholderTxtB.TabIndex = 0;
//
// Create a `button1` button to lose focus from the `PlaceholderTxtB` textbox
//
this.button1.Location = new System.Drawing.Point(57, 39);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(75, 23);
this.button1.TabIndex = 1;
this.button1.Text = "Focus";
this.button1.UseVisualStyleBackColor = true;
//
// Form1
//
this.ClientSize = new System.Drawing.Size(284, 261);
this.Controls.Add(this.button1);
this.Controls.Add(this.PlaceholderTxtB);
this.Name = "Form1";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.TextBox PlaceholderTxtB;
private System.Windows.Forms.Button button1;
}
}
Form1.cs
:
// `Form1.cs` code to add a placeholder text to `PlaceholderTxtB` textbox
using System;
using System.Drawing;
using System.Windows.Forms;
namespace TextboxPlaceholder {
public partial class Form1 : Form {
string ph = "Type your user name here...";
public Form1() {
InitializeComponent();
PlaceholderTxtB.GotFocus += RemoveText;
PlaceholderTxtB.LostFocus += AddText;
}
private void Form1_Load(object sender, EventArgs e) {}
public void RemoveText(object sender, EventArgs e) {
if (PlaceholderTxtB.Text == ph)
PlaceholderTxtB.Text = "";
}
public void AddText(object sender, EventArgs e) {
if (String.IsNullOrWhiteSpace(PlaceholderTxtB.Text)) {
PlaceholderTxtB.ForeColor = Color.Gray;
PlaceholderTxtB.Text = ph;
}
}
}
}
The Form1
load event contains InitializeComponent()
method which holds the code to create the PlaceholderTxtB
textbox. In Form1.cs
, create a .GotFocus
and .LostFocus
events of a textbox.
Whenever the PlaceholderTxtB
textbox gets the focus, the placeholder text will be removed. However, when the textbox loses the user focus and remains empty, the placeholder text will re-appear.
Use Enter
and Leave
Focus Events in Visual Studio IDE to Add Placeholder Text to a Textbox in C#
In Visual Studio, each component of a form got events. In the case of a textbox, you can find Focus
events under Properties
of your C# project forms.
Use Enter
and Leave
events to create a placeholder for your textbox.
// `Form1.cs` code for `Enter Focus` and `Leave Focus` events to add a placeholder text to a
// `textBox1` textbox
string phd = "Password@123";
private void Enter_Focus(object sender, EventArgs e) {
// textBox1.ForeColor = Color.Gray;
if (textBox1.Text == phd)
textBox1.Text = "";
}
private void Leave_Focus(object sender, EventArgs e) {
textBox1.ForeColor = Color.Gray;
if (textBox1.Text == "")
textBox1.Text = phd;
}
The textBox1.Text = phd
is a placeholder text shown when the input is empty, and the user loses focus of a textbox to suggest a possible value. The Enter_Focus
and Leave_Focus
events of a textbox get or set the placeholder text displayed when the control has no text and does not have the focus.
Install Bunifu UI
in Visual Studio to Add the Textbox With a Placeholder in C# Projects
Use NuGet
to easily install and activate Bunifu UI
. In Visual Studio IDE, NuGet
offers a faster and easier way of installing, activating, and deploying your applications seamlessly.
To install Bunifu UI
via NuGet Package Manager Console
, run:
Install-Package Bunifu.UI.WinForms
In your C# project, go to Tools
and click Manage NuGet Packages for Solution...
by accessing NuGet Package Manager
. Afterward, you will see the NuGet - Solution
tab, navigate its Browse
section and search Bunifu
.
You will find the Bunifu.UI.WinForms
package in the search results and can install it. Add it to your C# project to see its list of controls in the Toolbar
tab.
It offers a textbox with pre-defined text placeholders. Locate BunifuTextBox
inside the Toolbox
of your C# Visual Studio project and drag it onto your form.
Customize it by modifying its properties, and you will have a textbox with a placeholder text.
In this tutorial, you have learned the three different methods to add placeholder text in the text area of a textbox to provide a clearer interface for users. However, disappearing placeholders can confuse you if there’s nothing else labeling your text boxes.
Fortunately, with the solutions you will get in this tutorial, the placeholder text remains until the user starts entering text. Placeholders as inline identify what information to input in a textbox, limiting its accessibility.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub