在 C# 中將佔位符新增到文字框
-
在
C#
中建立一個帶有佔位符文字的文字框 -
在 Visual Studio IDE 中使用
Enter
和Leave
焦點事件將佔位符文字新增到C#
中的文字框 -
在 Visual Studio 中安裝
Bunifu UI
以在 C# 專案中新增帶有佔位符的文字框
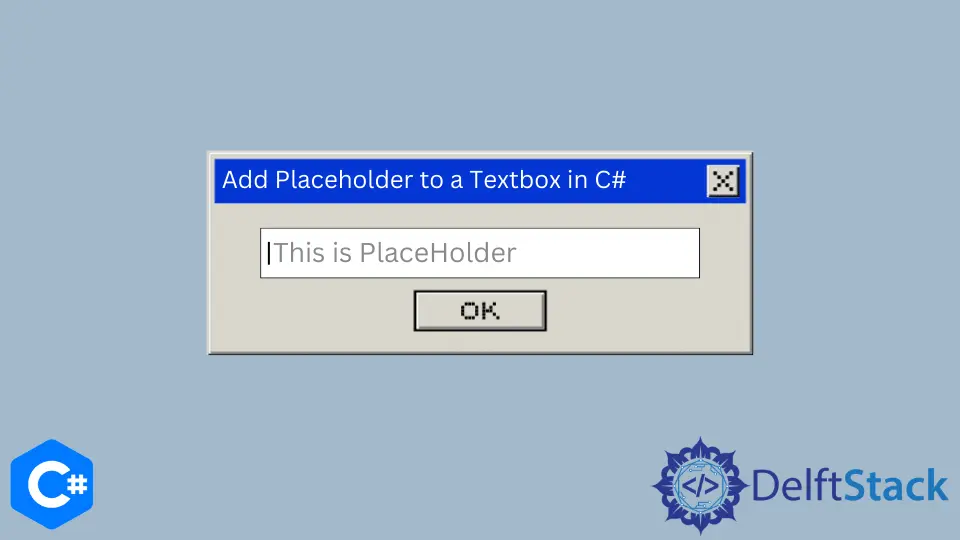
本教程將教授在 C# 中向文字框新增佔位符的三種方法。由於佔位符應被視為提示,因此它們不能替代 labels
或 title
。
在 C#
中建立一個帶有佔位符文字的文字框
建立一個帶有佔位符文字的文字框,該文字框會消失並允許使用者輸入他們的文字,從而為開發人員提供了完全自定義的自由。此外,它允許你設定表單元素的佔位符文字的樣式。
通常,文字框包含佔位符;當它被聚焦時,佔位符將被刪除,以便使用者新增文字。如果文字框失去焦點並且使用者沒有輸入任何文字,則佔位符將新增回文字框。
以下 C# 程式碼將建立一個帶有佔位符的文字框。
Form1.Designer.cs
:
// `Form1` as your C# project form
// `Form1.Designer.cs` Code to create a `PlaceholderTxtB` textbox
namespace TextboxPlaceholder {
partial class Form1 {
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing) {
if (disposing && (components != null)) {
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
private void InitializeComponent() {
this.PlaceholderTxtB = new System.Windows.Forms.TextBox();
this.button1 = new System.Windows.Forms.Button();
this.SuspendLayout();
//
// PlaceholderTxtB
//
this.PlaceholderTxtB.Location = new System.Drawing.Point(12, 12);
this.PlaceholderTxtB.Name = "PlaceholderTxtB";
this.PlaceholderTxtB.Size = new System.Drawing.Size(188, 20);
this.PlaceholderTxtB.TabIndex = 0;
//
// Create a `button1` button to lose focus from the `PlaceholderTxtB` textbox
//
this.button1.Location = new System.Drawing.Point(57, 39);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(75, 23);
this.button1.TabIndex = 1;
this.button1.Text = "Focus";
this.button1.UseVisualStyleBackColor = true;
//
// Form1
//
this.ClientSize = new System.Drawing.Size(284, 261);
this.Controls.Add(this.button1);
this.Controls.Add(this.PlaceholderTxtB);
this.Name = "Form1";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.TextBox PlaceholderTxtB;
private System.Windows.Forms.Button button1;
}
}
Form1.cs
:
// `Form1.cs` code to add a placeholder text to `PlaceholderTxtB` textbox
using System;
using System.Drawing;
using System.Windows.Forms;
namespace TextboxPlaceholder {
public partial class Form1 : Form {
string ph = "Type your user name here...";
public Form1() {
InitializeComponent();
PlaceholderTxtB.GotFocus += RemoveText;
PlaceholderTxtB.LostFocus += AddText;
}
private void Form1_Load(object sender, EventArgs e) {}
public void RemoveText(object sender, EventArgs e) {
if (PlaceholderTxtB.Text == ph)
PlaceholderTxtB.Text = "";
}
public void AddText(object sender, EventArgs e) {
if (String.IsNullOrWhiteSpace(PlaceholderTxtB.Text)) {
PlaceholderTxtB.ForeColor = Color.Gray;
PlaceholderTxtB.Text = ph;
}
}
}
}
Form1
載入事件包含 InitializeComponent()
方法,該方法包含建立 PlaceholderTxtB
文字框的程式碼。在 Form1.cs
中,建立一個文字框的 .GotFocus
和 .LostFocus
事件。
每當 PlaceholderTxtB
文字框獲得焦點時,佔位符文字將被刪除。但是,當文字框失去使用者焦點並保持為空時,佔位符文字將重新出現。
在 Visual Studio IDE 中使用 Enter
和 Leave
焦點事件將佔位符文字新增到 C#
中的文字框
在 Visual Studio 中,表單的每個元件都有事件。對於文字框,你可以在 C# 專案表單的 Properties
下找到 Focus
事件。
使用 Enter
和 Leave
事件為你的文字框建立一個佔位符。
// `Form1.cs` code for `Enter Focus` and `Leave Focus` events to add a placeholder text to a
// `textBox1` textbox
string phd = "Password@123";
private void Enter_Focus(object sender, EventArgs e) {
// textBox1.ForeColor = Color.Gray;
if (textBox1.Text == phd)
textBox1.Text = "";
}
private void Leave_Focus(object sender, EventArgs e) {
textBox1.ForeColor = Color.Gray;
if (textBox1.Text == "")
textBox1.Text = phd;
}
textBox1.Text = phd
是輸入為空時顯示的佔位符文字,並且使用者失去文字框的焦點以建議可能的值。文字框的 Enter_Focus
和 Leave_Focus
事件獲取或設定控制元件沒有文字且沒有焦點時顯示的佔位符文字。
在 Visual Studio 中安裝 Bunifu UI
以在 C# 專案中新增帶有佔位符的文字框
使用 NuGet
輕鬆安裝和啟用 Bunifu UI
。在 Visual Studio IDE 中,NuGet
提供了一種更快、更輕鬆的無縫安裝、啟用和部署應用程式的方法。
要通過 NuGet Package Manager Console
安裝 Bunifu UI
,請執行:
Install-Package Bunifu.UI.WinForms
在你的 C# 專案中,轉到工具
並通過訪問 NuGet 包管理器
單擊管理 NuGet 包以獲取解決方案...
。之後,你將看到 NuGet - 解決方案
選項卡,導航其瀏覽
部分並搜尋 Bunifu
。
你將在搜尋結果中找到 Bunifu.UI.WinForms
包並可以安裝它。將其新增到你的 C# 專案以在 工具欄
選項卡中檢視其控制元件列表。
它提供了一個帶有預定義文字佔位符的文字框。在 C# Visual Studio 專案的 Toolbox
中找到 BunifuTextBox
,並將其拖到你的表單上。
通過修改其屬性對其進行自定義,你將擁有一個帶有佔位符文字的文字框。
在本教程中,你學習了在文字框的文字區域中新增佔位符文字的三種不同方法,以便為使用者提供更清晰的介面。但是,如果沒有其他任何東西標記你的文字框,消失的佔位符可能會讓你感到困惑。
幸運的是,使用本教程中的解決方案,佔位符文字會一直保留到使用者開始輸入文字。內聯佔位符標識要在文字框中輸入的資訊,從而限制其可訪問性。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub