C# 新建一個僅接受數字的文字框
Minahil Noor
2024年2月16日
- 使用 C# 中的 KeyPressEventArgs 類建立一個僅接受數字的文字框
-
使用 C# 中的
Regex.IsMatch()
方法建立一個僅接受數字的文字框Textbox
-
使用
NumericUpDown
方法建立一個僅接受數字的文字框Textbox
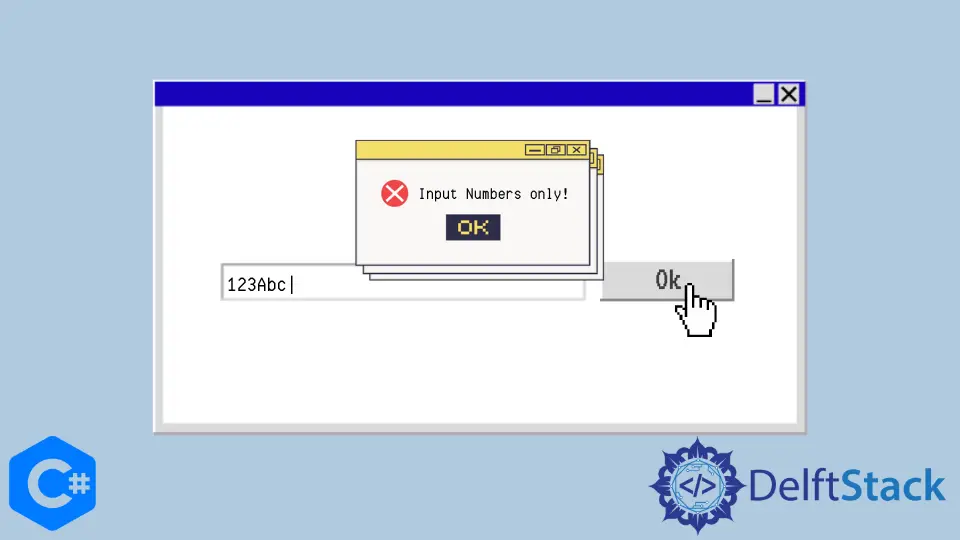
在製作 Windows 窗體時,某些文字欄位只需要一個數字值。例如,如果我們想從使用者那裡獲得電話號碼,那麼我們將不得不將文字框限制為僅接受數字值。
在本文中,我們將重點介紹構成僅接受數字的文字框的方法。
使用 C# 中的 KeyPressEventArgs 類建立一個僅接受數字的文字框
KeyPressEventArgs
是一個 C# 類,用於指定使用者輸入的字元按下一個鍵。它的 KeyChar
屬性返回使用者鍵入的字元。在這裡,我們使用了 KeyPress
事件將文字框限制為僅數字值。
執行此操作的關鍵程式碼如下:
!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.');
這裡 e
是一個 KeyPressEventArgs
物件,該物件使用 KeyChar
屬性來獲取輸入的鍵值。
示例程式碼:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_KeyPress(object sender, KeyPressEventArgs e) {
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.')) {
e.Handled = true;
}
// only allow one decimal point
if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1)) {
e.Handled = true;
}
}
}
}
輸出:
//only allows numeric values in the textbox
使用 C# 中的 Regex.IsMatch()
方法建立一個僅接受數字的文字框 Textbox
在 C# 中,我們可以使用正規表示式來檢查各種模式。正規表示式是執行特定操作的特定模式。RegularExpressions 是一個 C# 類,其中包含 Regex.IsMatch()方法的定義。在 C# 中,我們使用 ^[0-9]+$
和 ^\d+$
正規表示式來檢查字串是否為數字。
此方法的正確語法如下:
Regex.IsMatch(StringName, @"Expression");
示例程式碼:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_TextChanged(object sender, EventArgs e) {
if (System.Text.RegularExpressions.Regex.IsMatch(textBox1.Text, " ^ [0-9]")) {
textBox1.Text = "";
}
}
}
}
輸出:
//A textbox created that only accepts numbers.
使用 NumericUpDown
方法建立一個僅接受數字的文字框 Textbox
NumericUpDown
為使用者提供了使用文字框提供的向上和向下按鈕輸入數值的介面。你可以簡單地從工具箱中拖放 NumericUpDown
來建立僅接受數字的文字框。
你還可以動態建立一個 NumericUpDown
物件。生成 NumericUpDown
的程式碼如下:
private System.Windows.Forms.NumericUpDown numericUpDown;
this.numericUpDown = new System.Windows.Forms.NumericUpDown();
它具有幾個屬性,你可以通過開啟屬性視窗來更改。
輸出: