C# 新建一个仅接受数字的文本框
Minahil Noor
2024年2月16日
- 使用 C# 中的 KeyPressEventArgs 类创建一个仅接受数字的文本框
-
使用 C# 中的
Regex.IsMatch()
方法创建一个仅接受数字的文本框Textbox
-
使用
NumericUpDown
方法创建一个仅接受数字的文本框Textbox
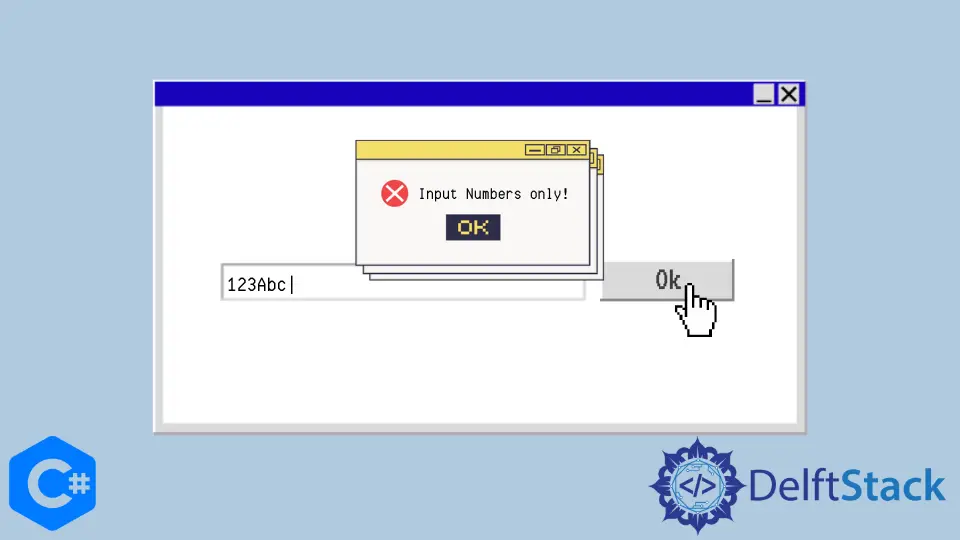
在制作 Windows 窗体时,某些文本字段只需要一个数字值。例如,如果我们想从用户那里获得电话号码,那么我们将不得不将文本框限制为仅接受数字值。
在本文中,我们将重点介绍构成仅接受数字的文本框的方法。
使用 C# 中的 KeyPressEventArgs 类创建一个仅接受数字的文本框
KeyPressEventArgs
是一个 C# 类,用于指定用户输入的字符按下一个键。它的 KeyChar
属性返回用户键入的字符。在这里,我们使用了 KeyPress
事件将文本框限制为仅数字值。
执行此操作的关键代码如下:
!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.');
这里 e
是一个 KeyPressEventArgs
对象,该对象使用 KeyChar
属性来获取输入的键值。
示例代码:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_KeyPress(object sender, KeyPressEventArgs e) {
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.')) {
e.Handled = true;
}
// only allow one decimal point
if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1)) {
e.Handled = true;
}
}
}
}
输出:
//only allows numeric values in the textbox
使用 C# 中的 Regex.IsMatch()
方法创建一个仅接受数字的文本框 Textbox
在 C# 中,我们可以使用正则表达式来检查各种模式。正则表达式是执行特定操作的特定模式。RegularExpressions 是一个 C# 类,其中包含 Regex.IsMatch()方法的定义。在 C# 中,我们使用 ^[0-9]+$
和 ^\d+$
正则表达式来检查字符串是否为数字。
此方法的正确语法如下:
Regex.IsMatch(StringName, @"Expression");
示例代码:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_TextChanged(object sender, EventArgs e) {
if (System.Text.RegularExpressions.Regex.IsMatch(textBox1.Text, " ^ [0-9]")) {
textBox1.Text = "";
}
}
}
}
输出:
//A textbox created that only accepts numbers.
使用 NumericUpDown
方法创建一个仅接受数字的文本框 Textbox
NumericUpDown
为用户提供了使用文本框提供的向上和向下按钮输入数值的界面。你可以简单地从工具箱中拖放 NumericUpDown
来创建仅接受数字的文本框。
你还可以动态创建一个 NumericUpDown
对象。生成 NumericUpDown
的代码如下:
private System.Windows.Forms.NumericUpDown numericUpDown;
this.numericUpDown = new System.Windows.Forms.NumericUpDown();
它具有几个属性,你可以通过打开属性窗口来更改。
输出: