C# 숫자 만 허용하는 텍스트 상자 만들기
-
C#에서
KeyPressEventArgs
클래스를 사용하여 ‘숫자’만 허용하는 ‘텍스트 상자’를 만듭니다 -
C#에서
Regex.IsMatch()
메서드를 사용하여 숫자 만 허용하는 ‘텍스트 상자’를 만듭니다 -
NumericUpDown
메서드를 사용하여 숫자 만 허용하는 텍스트 상자 만들기
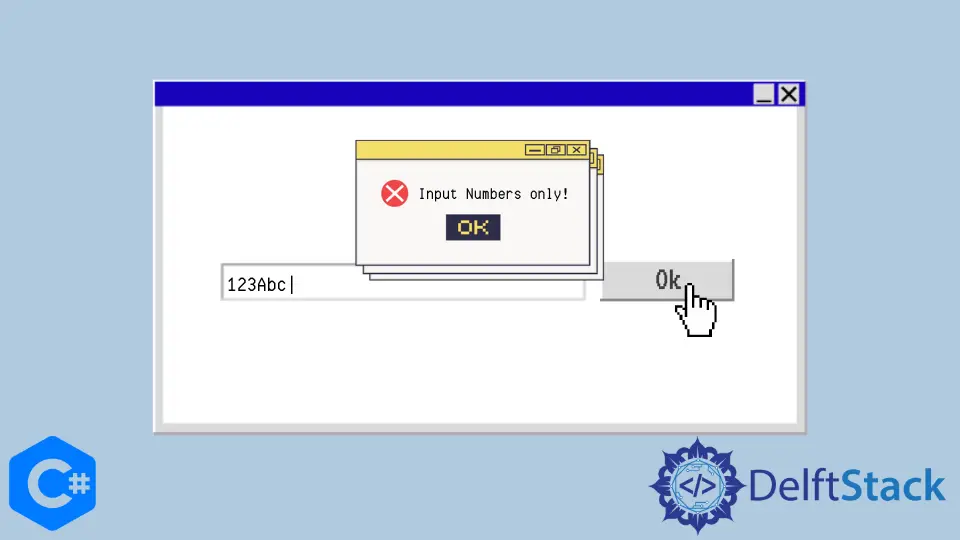
Windows Forms를 만드는 동안 일부 텍스트 필드에는 숫자 값만 필요합니다. 예를 들어, 사용자로부터 전화 번호를 얻으려면 ‘텍스트 상자’를 숫자 값으로 만 제한해야합니다.
이 기사에서는 숫자 만 허용하는 ‘텍스트 상자’를 만드는 방법에 중점을 둘 것입니다.
C#에서KeyPressEventArgs
클래스를 사용하여 ‘숫자’만 허용하는 ‘텍스트 상자’를 만듭니다
KeyPressEventArgs
는 사용자가 입력 할 때 입력 한 문자를 지정하는 C# 클래스입니다. 키를 누른다. KeyChar 속성은 사용자가 입력 한 문자를 반환합니다. 여기서는 KeyPress
이벤트를 사용하여 textbox
를 숫자 값으로 만 제한했습니다.
이 작업을 수행하는 키 코드는 다음과 같습니다.
!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.');
e
는KeyChar
속성을 사용하여 입력 된 키를 가져 오는KeyPressEventArgs
객체입니다.
예제 코드:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_KeyPress(object sender, KeyPressEventArgs e) {
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.')) {
e.Handled = true;
}
// only allow one decimal point
if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1)) {
e.Handled = true;
}
}
}
}
출력:
//only allows numeric values in the textbox
C#에서Regex.IsMatch()
메서드를 사용하여 숫자 만 허용하는 ‘텍스트 상자’를 만듭니다
C#에서는 정규식을 사용하여 다양한 패턴을 확인할 수 있습니다. 정규식은 특정 작업을 수행하기위한 특정 패턴입니다. RegularExpressions
는Regex.IsMatch()
메소드에 대한 정의를 포함하는 C# 클래스입니다. C#에는^[0-9]+$
및^\d+$
정규식이있어string
이 숫자인지 확인합니다.
이 방법을 사용하는 올바른 구문은 다음과 같습니다.
Regex.IsMatch(StringName, @"Expression");
예제 코드:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_TextChanged(object sender, EventArgs e) {
if (System.Text.RegularExpressions.Regex.IsMatch(textBox1.Text, " ^ [0-9]")) {
textBox1.Text = "";
}
}
}
}
출력:
//A textbox created that only accepts numbers.
NumericUpDown
메서드를 사용하여 숫자 만 허용하는 텍스트 상자 만들기
NumericUpDown
은 텍스트 상자가 제공하는 상향 및 하향 버튼을 사용하여 수치를 입력할 수 있는 인터페이스를 사용자에게 제공합니다.너는 간단히 공구상자에서 NumericUpDown
을 끌어다 놓아서 숫자만 받는 텍스트 상자를 만들 수 있다.
NumericUpDown
객체를 동적으로 생성 할 수도 있습니다. NumericUpDown
을 생성하는 코드는 다음과 같습니다.
private System.Windows.Forms.NumericUpDown numericUpDown;
this.numericUpDown = new System.Windows.Forms.NumericUpDown();
속성 창을 열어서 수정할 수있는 몇 가지 속성이 있습니다.
출력: