C# 数字のみを受け入れるテキストボックスを作成する
-
C# の
KeyPressEventArgs
クラスを使用して、Numbers
のみを受け入れるTextbox
を作成する -
C# で
Regex.IsMatch()
メソッドを使用して、Numbers
のみを受け入れるTextbox
を作成する -
NumericUpDown
メソッドを使用して、Numbers
のみを受け入れるTextbox
を作成する
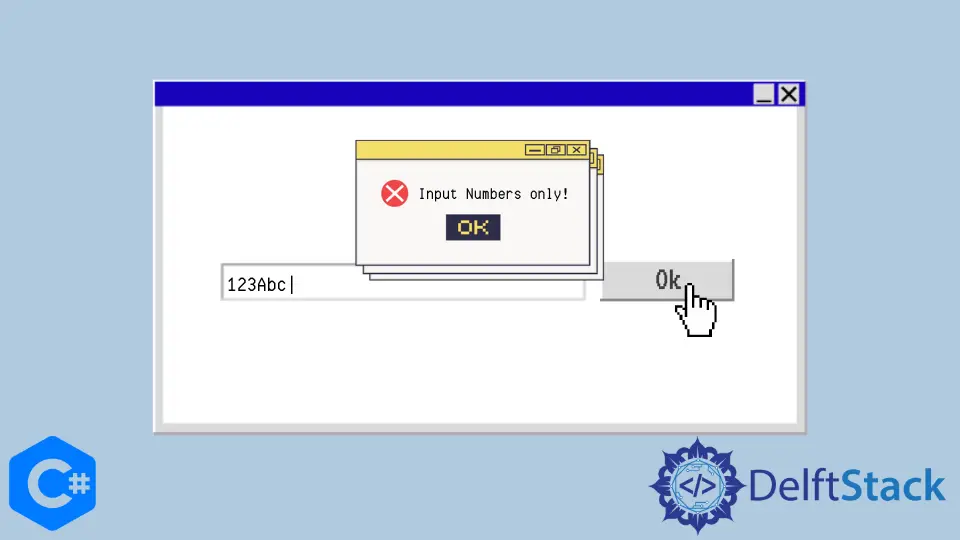
Windows フォーム
を作成している間、一部のテキストフィールドには数値しか必要ありません。たとえば、ユーザーから電話番号を取得する場合は、textbox
を数値のみに制限する必要があります。
この記事では、数値のみを受け入れる textbox
を作成するメソッドを中心に紹介します。
C# の KeyPressEventArgs
クラスを使用して、Numbers
のみを受け入れる Textbox
を作成する
KeyPressEventArgs
は、ユーザーが入力したときに入力された文字を指定する C# クラスですキーを押します。その KeyChar
プロパティはユーザーが入力した文字を返します。ここでは、KeyPress
イベントを使用して、textbox
を数値のみに制限しています。
このアクションを実行するキーコードは次のとおりです。
!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.');
ここで e
は、KeyChar
プロパティを使用して入力されたキーを取得する KeyPressEventArgs
オブジェクトです。
コード例:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_KeyPress(object sender, KeyPressEventArgs e) {
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.')) {
e.Handled = true;
}
// only allow one decimal point
if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1)) {
e.Handled = true;
}
}
}
}
出力:
//only allows numeric values in the textbox
C# で Regex.IsMatch()
メソッドを使用して、Numbers
のみを受け入れる Textbox
を作成する
C# では、正規表現を使用してさまざまなパターンをチェックできます。正規表現は、特定のアクションを実行するための特定のパターンです。RegularExpressions
は C# クラスで、Regex.IsMatch()
メソッドの定義が含まれています。C# には、^[0-9]+$
および ^\d+$
正規表現があり、string
が数値であるかどうかを確認できます。
このメソッドを使用するための正しい構文は次のとおりです。
Regex.IsMatch(StringName, @"Expression");
コード例:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_TextChanged(object sender, EventArgs e) {
if (System.Text.RegularExpressions.Regex.IsMatch(textBox1.Text, " ^ [0-9]")) {
textBox1.Text = "";
}
}
}
}
出力:
//A textbox created that only accepts numbers.
NumericUpDown
メソッドを使用して、Numbers
のみを受け入れる Textbox
を作成する
NumericUpDown
は、textbox
で指定された上下ボタンを使用して数値を入力するためのインターフェースをユーザーに提供します。NumericUpDown
をツールボックス
からドラッグアンドドロップするだけで、数値
のみを受け入れるテキストボックス
を作成できます。
NumericUpDown
オブジェクトを動的に作成することもできます。NumericUpDown
を生成するコードは次のとおりです。
private System.Windows.Forms.NumericUpDown numericUpDown;
this.numericUpDown = new System.Windows.Forms.NumericUpDown();
これにはいくつかのプロパティがあり、プロパティウィンドウ
を開いて変更できます。
出力: